How to Check If a Variable Exists in Python
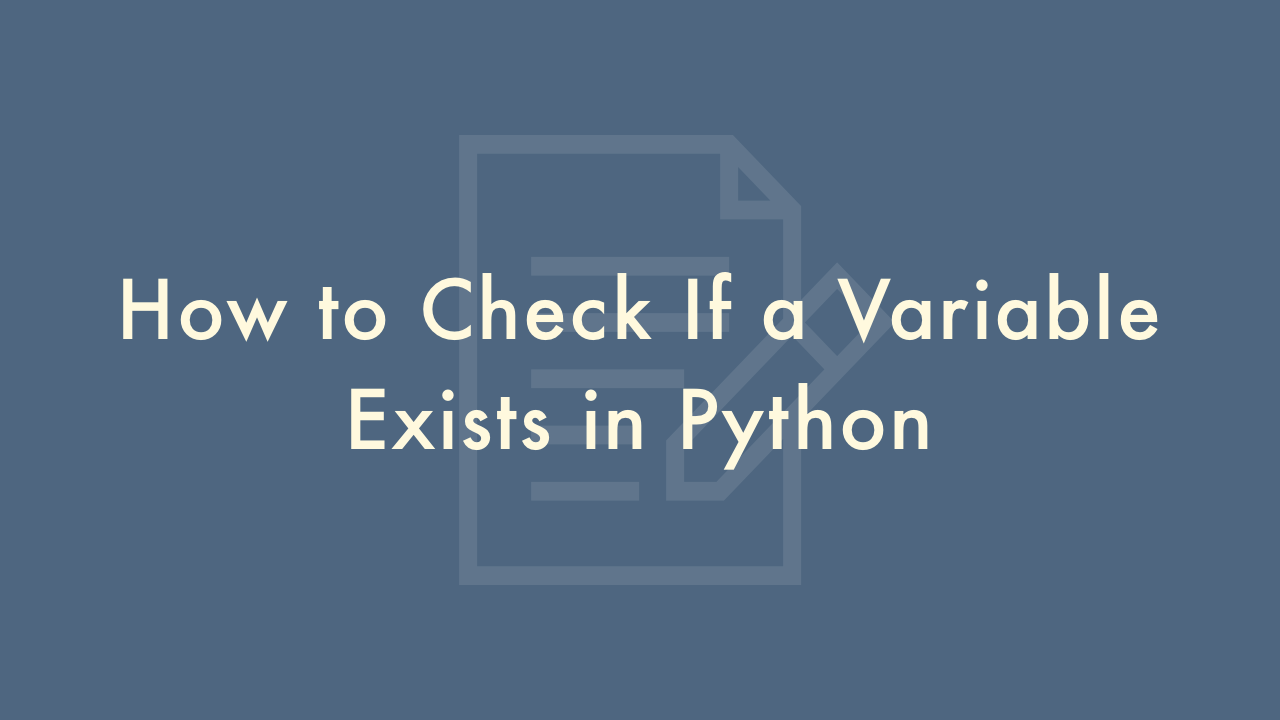
Contents
In this article, you will learn how to check if a variable exists in Python.
How to check if a variable exists
In Python, you can check if a variable exists by using the in operator with the locals() or globals() function, depending on where the variable is expected to be defined.
Here’s an example:
if 'my_variable' in locals():
print('my_variable exists in the current scope.')
else:
print('my_variable does not exist in the current scope.')
In this code snippet, locals() is a function that returns a dictionary representing the local symbol table. It contains the variables and their values defined in the current scope.
Similarly, you can use globals() to check for the existence of a variable in the global scope:
if 'my_variable' in globals():
print('my_variable exists in the global scope.')
else:
print('my_variable does not exist in the global scope.')
If the variable exists, the code will print a message indicating that it exists. If it does not exist, it will print a message indicating that it does not exist.
In addition to the in operator with locals() and globals(), there are a few other ways to check if a variable exists in Python:
- hasattr() function: This function checks if an object has an attribute with a given name. For example, you can check if a module has an attribute by using hasattr(module, ‘attribute_name’).
-
try-except block: You can also use a try-except block to catch a NameError exception if the variable does not exist. For example:
try: my_variable print('my_variable exists.') except NameError: print('my_variable does not exist.')
This code snippet attempts to access the my_variable variable, and if it does not exist, a NameError exception is raised and caught in the except block.
It’s important to note that using try-except blocks to check for variable existence can have some downsides. For example, it can be slower than using in with locals() or globals(), and it can also mask other potential errors that may occur in the code.