How to Use Python argparse Module
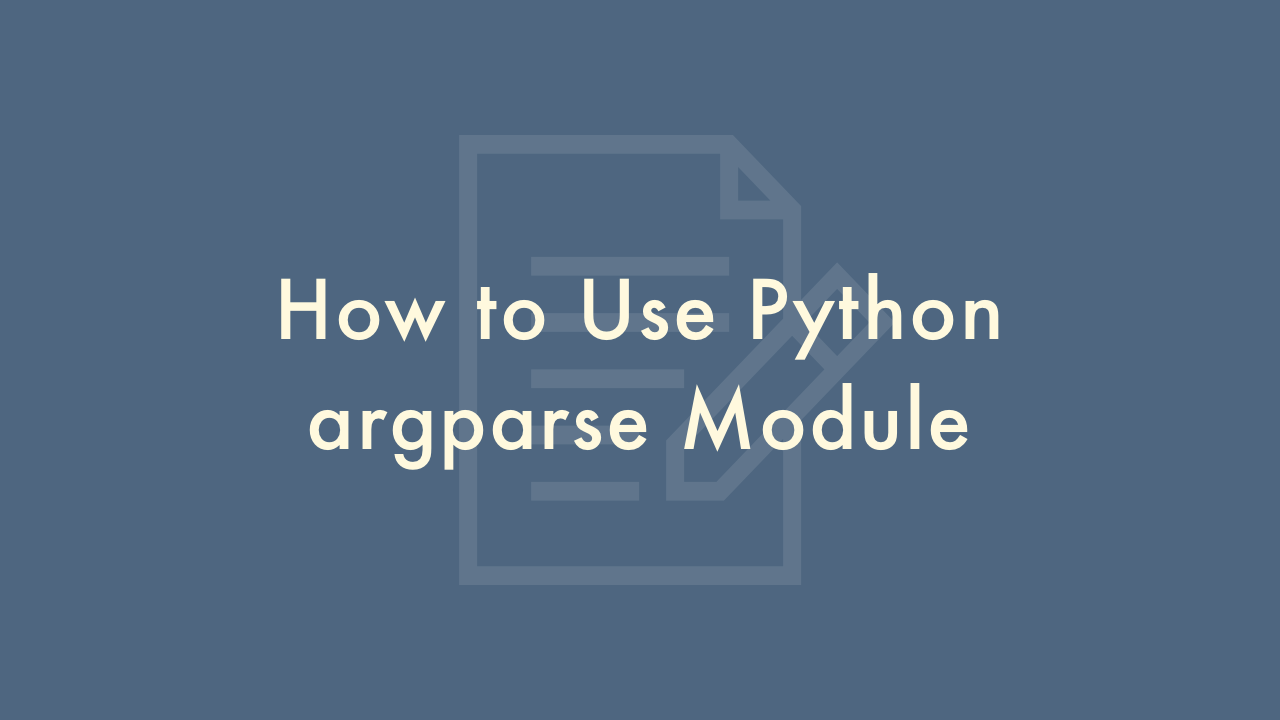
Contents
In this article, you will learn how to use Python argparse module.
Python argparse Module
Python’s argparse module provides an easy way to parse command-line arguments and options in a standardized and convenient way. Here are the basic steps to using argparse:
Import the argparse module:
import argparse
Create an instance of the ArgumentParser class:
parser = argparse.ArgumentParser()
Define the arguments and options your program will accept using the add_argument() method. For example, to add a required positional argument:
parser.add_argument("filename", help="the name of the file to process")
You can also add optional arguments using the — prefix or shorthand options using the – prefix, using the add_argument() method. For example, to add an optional –verbose argument with a shorthand -v option:
parser.add_argument("-v", "--verbose", help="increase output verbosity", action="store_true")
Once all arguments and options are defined, call the parse_args() method to parse the command line arguments and options into a namespace object:
args = parser.parse_args()
You can now access the values of the parsed arguments and options through the attributes of the args object. For example:
print(args.filename)
if args.verbose:
print("Verbose mode enabled")
Here’s a complete example that shows how to use argparse to accept a filename and a –verbose option:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("filename", help="the name of the file to process")
parser.add_argument("-v", "--verbose", help="increase output verbosity", action="store_true")
args = parser.parse_args()
print(args.filename)
if args.verbose:
print("Verbose mode enabled")
Assuming the code above is saved in a file named my_program.py, you could run it from the command line like this:
$ python my_program.py myfile.txt -v
This would print the filename and enable verbose mode.