How to Use Python Datetime Module
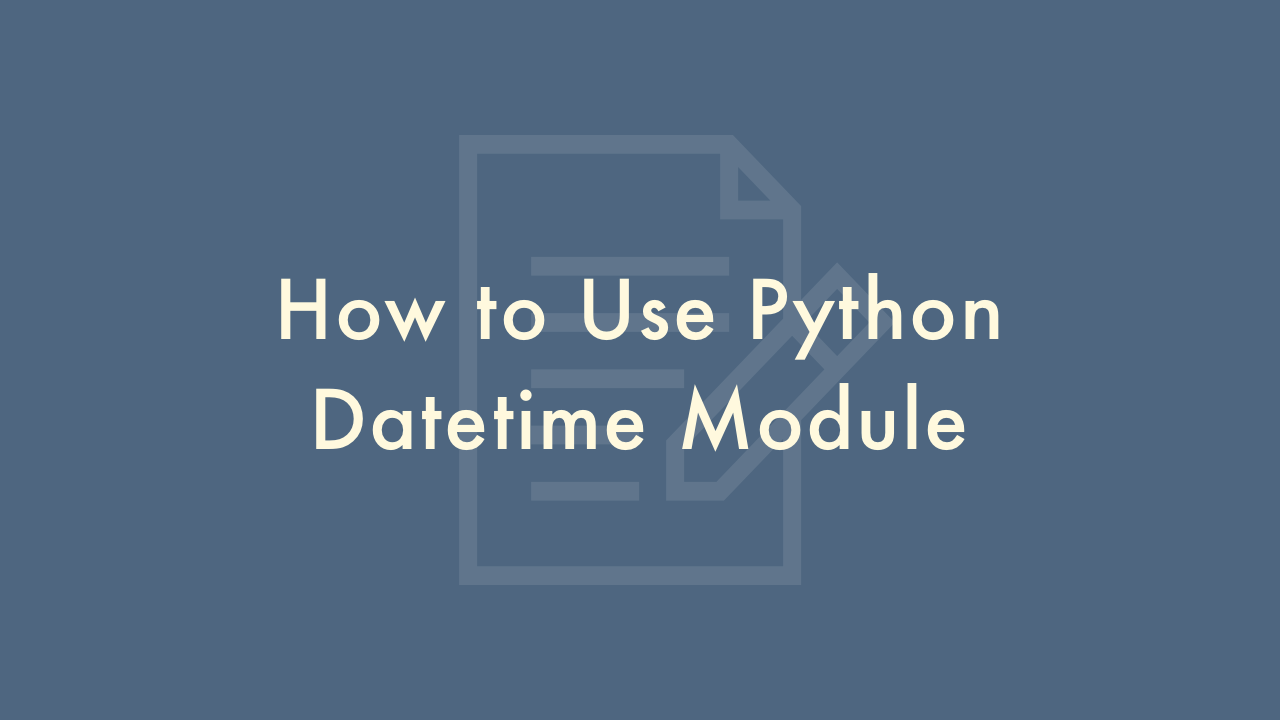
Contents
In this article, you will learn how to use Python datetime module.
Python Datetime Module
The datetime module in Python is part of the standard library and provides classes for working with dates and times. Here’s an overview of how you can use some of the most commonly used classes in the datetime module:
date:
Represents a date (year, month, day). You can create a date object by calling the date constructor and passing in the year, month, and day as arguments. For example:
from datetime import date
d = date(2021, 1, 1)
print(d) # Output: 2021-01-01
time:
Represents a time of day (hours, minutes, seconds, milliseconds). You can create a time object by calling the time constructor and passing in the hour, minute, second, and microsecond as arguments. For example:
from datetime import time
t = time(12, 30, 45)
print(t) # Output: 12:30:45
datetime:
Represents a date and time. You can create a datetime object by calling the datetime constructor and passing in the year, month, day, hour, minute, second, and microsecond as arguments. For example:
from datetime import datetime
dt = datetime(2021, 1, 1, 12, 30, 45)
print(dt) # Output: 2021-01-01 12:30:45
timedelta:
Represents a duration or a difference between two dates or times. You can create a timedelta object by calling the timedelta constructor and passing in the number of days, seconds, microseconds, milliseconds, minutes, hours, or weeks as arguments. For example:
from datetime import timedelta
delta = timedelta(days=7)
print(delta) # Output: 7 days, 0:00:00
tzinfo:
Represents a time zone. The datetime module does not include a time zone database, but you can use the pytz library to get time zone information.
Formatting dates and times:
You can format dates and times using the strftime method. This method takes a format string as an argument and returns a string representing the date or time in the specified format. For example:
from datetime import datetime
dt = datetime(2021, 1, 1, 12, 30, 45)
print(dt.strftime('%Y-%m-%d %H:%M:%S')) # Output: 2021-01-01 12:30:45
These are some of the most commonly used classes and methods in the datetime module. You can find more information and examples in the official Python documentation: https://docs.python.org/3/library/datetime.html