How to Use Self in Python
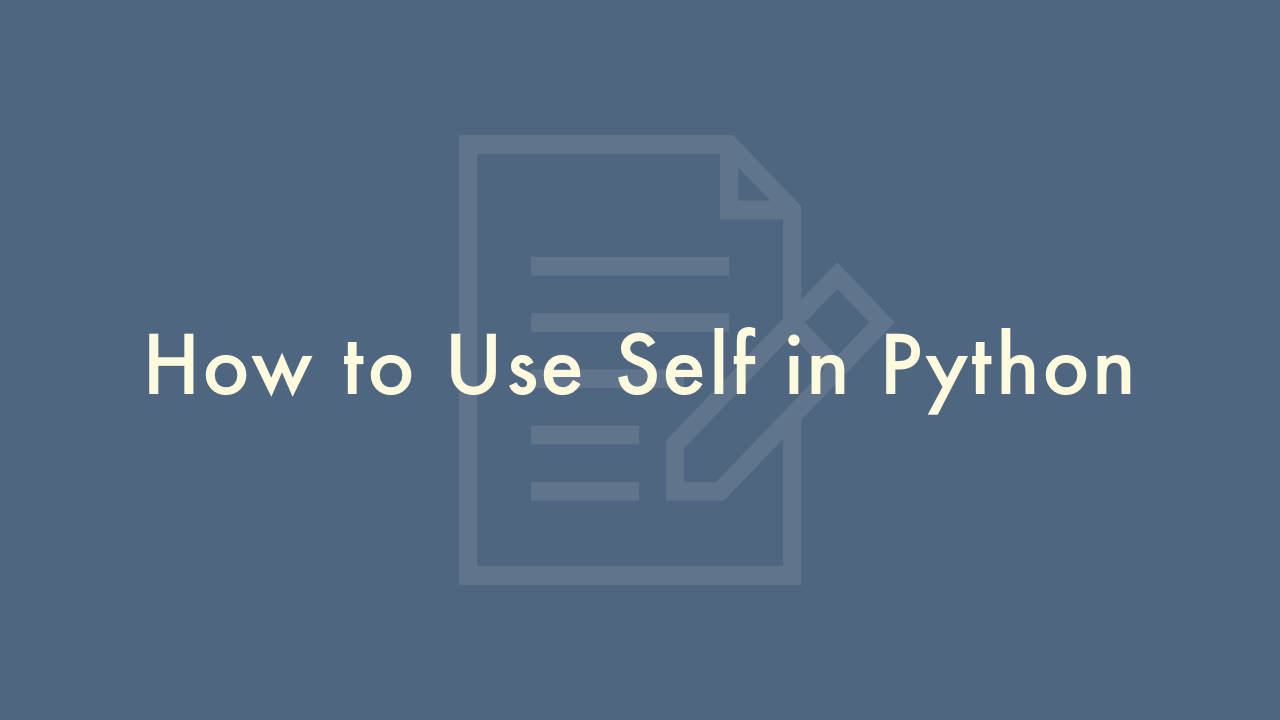
Contents
In this article, you will learn how to use self in Python.
Use Self in Python
In Python, the self keyword is used to refer to the instance of an object that is calling a method. It is a convention that is used in object-oriented programming to refer to the instance of an object from within that object’s own methods.
Here’s an example of how you might use self
in a class:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def get_description(self):
return f'{self.make} {self.model}'
my_car = Car('Toyota', 'Camry')
print(my_car.get_description())
In this example, the Car class has an __init__ method that takes two parameters make
and model
and sets them as instance variables using self
. The get_description method uses self
to refer to the instance of the Car object that is calling the method, and returns a string that includes the make and model of the car.
When we create an instance of the Car class and call the get_description method, self
refers to the instance of the object, which is my_car. The call to my_car.get_description() will return the string ‘Toyota Camry’.
It’s important to note that the name self is just a convention, and you can use any other name you like instead. However, using self is widely accepted as the standard, and using a different name could make your code harder to understand for others.
You can also use self
to access other methods of the same class. For example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print("Hello, my name is", self.name)
def introduce_yourself(self):
self.say_hello()
print("I am", self.age, "years old.")
p = Person("John Doe", 30)
p.introduce_yourself()
In this example, the introduce_yourself method uses self to call the say_hello method of the same class, which then prints a message that includes the person’s name.