How to Read RSS Feed in Python
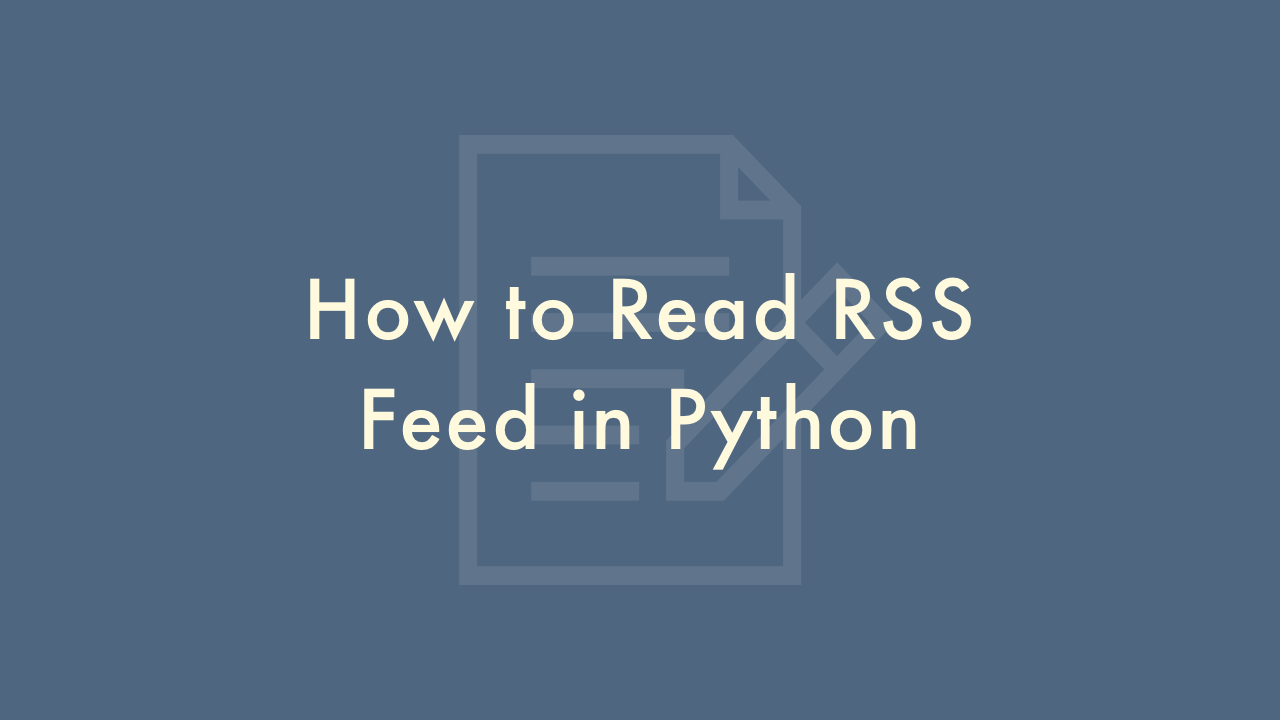
09/11/2021
Contents
In this article, you will learn how to read RSS feed in Python.
Read RSS Feed in Python
Reading RSS feeds in Python can be done using the built-in feedparser library. Here are the steps you can follow:
Install feedparser library by running the following command in your terminal:
pip install feedparser
Import the feedparser library in your Python script:
import feedparser
Use the feedparser.parse() function to parse the RSS feed:
This function returns a dictionary with the parsed feed data.
rss_url = "https://example.com/feed"
feed = feedparser.parse(rss_url)
Replace “https://example.com/feed” with the URL of the RSS feed you want to read.
Access the feed data:
Now, you can access the feed data using the dictionary keys. For example, to print the title and description of each feed item, you can use a for loop like this:
for item in feed["items"]:
print(item["title"])
print(item["description"])
print("\n")
The feed[“items”] key contains a list of all feed items.