How to Overload a Constructor in Python
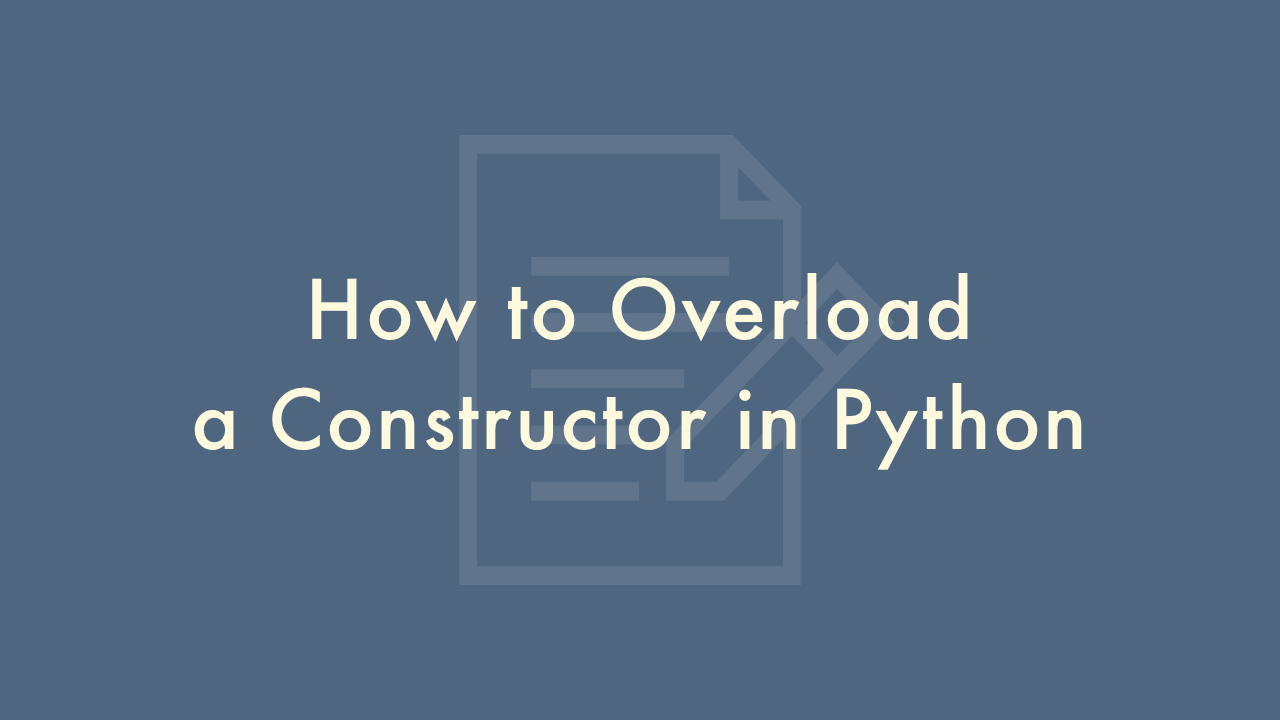
Contents
In this article, you will learn how to overload a constructor in Python.
Overload a constructor in Python
In Python, you can overload a constructor by defining multiple constructors with different numbers or types of parameters.
Python does not have true method overloading like some other programming languages. Instead, you can define multiple constructors with different arguments using default parameters or by using the *args and **kwargs syntax.
Here’s an example of how to overload a constructor in Python:
class MyClass:
def __init__(self):
# Constructor with no arguments
self.my_var = 0
def __init__(self, arg1):
# Constructor with one argument
self.my_var = arg1
def __init__(self, arg1, arg2):
# Constructor with two arguments
self.my_var = arg1 + arg2
In this example, we define the MyClass with three constructors, each with a different number of arguments. The first constructor takes no arguments and sets the my_var attribute to 0. The second constructor takes one argument and sets the my_var attribute to the value of the argument. The third constructor takes two arguments and sets the my_var attribute to the sum of the arguments.
When you create an instance of the class, Python will automatically select the appropriate constructor based on the number and types of arguments you pass. For example:
obj1 = MyClass() # Calls the first constructor with no arguments
obj2 = MyClass(10) # Calls the second constructor with one argument
obj3 = MyClass(5, 10) # Calls the third constructor with two arguments
Note that in Python, only the last constructor defined will be used, as previous constructors will be overwritten. Therefore, it’s recommended to use default parameters or *args and **kwargs to create multiple constructors.