How to Delete a File or Folder in Python
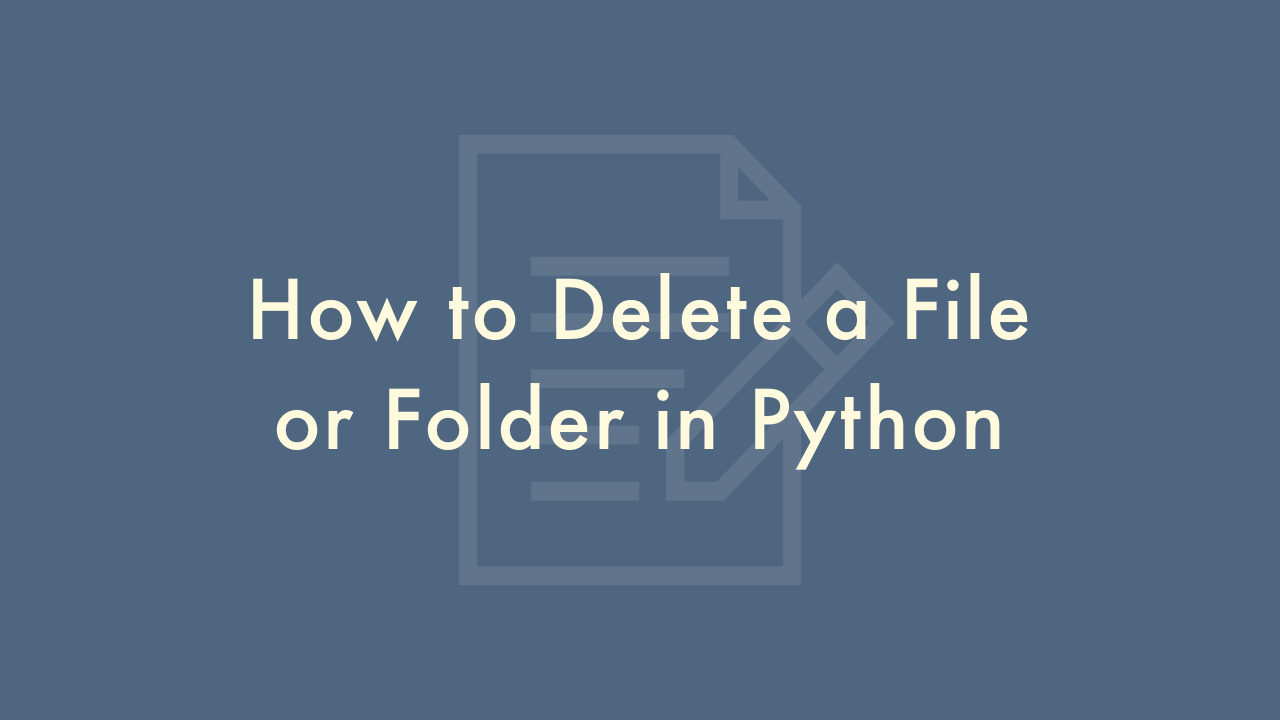
Contents
In this article, you will learn how to delete a file or folder in Python.
Deleting a File or Folder in Python
Deleting a File
To delete a file using the os.remove() function, you need to provide the full path to the file you want to delete as a string. For example, to delete a file named “example.txt” in the current directory, you can use:
import os
os.remove("example.txt")
If the file is in a different directory, you need to provide the full path to the file. For example, to delete a file named “example.txt” in a directory called “mydir” on the desktop, you can use:
import os
os.remove("/Users/username/Desktop/mydir/example.txt")
Note that if the file does not exist, you will get a FileNotFoundError.
Deleting an Empty Folder
To delete an empty folder using the os.rmdir() function, you need to provide the full path to the folder you want to delete as a string. For example, to delete an empty folder called “myfolder” in the current directory, you can use:
import os
os.rmdir("myfolder")
If the folder is in a different directory, you need to provide the full path to the folder. For example, to delete an empty folder called “myfolder” in a directory called “mydir” on the desktop, you can use:
import os
os.rmdir("/Users/username/Desktop/mydir/myfolder")
Note that if the folder is not empty, you will get a OSError.
Deleting a Folder and Its Contents
To delete a folder and its contents using the shutil.rmtree() function, you need to provide the full path to the folder you want to delete as a string. For example, to delete a folder called “myfolder” in the current directory, you can use:
import shutil
shutil.rmtree("myfolder")
If the folder is in a different directory, you need to provide the full path to the folder. For example, to delete a folder called “myfolder” in a directory called “mydir” on the desktop, you can use:
import shutil
shutil.rmtree("/Users/username/Desktop/mydir/myfolder")
Note that shutil.rmtree() will delete everything inside the folder, including any subfolders and their contents. Use this function with caution and make sure you specify the correct path to the folder you want to delete.