How to Use the Python iter() Function
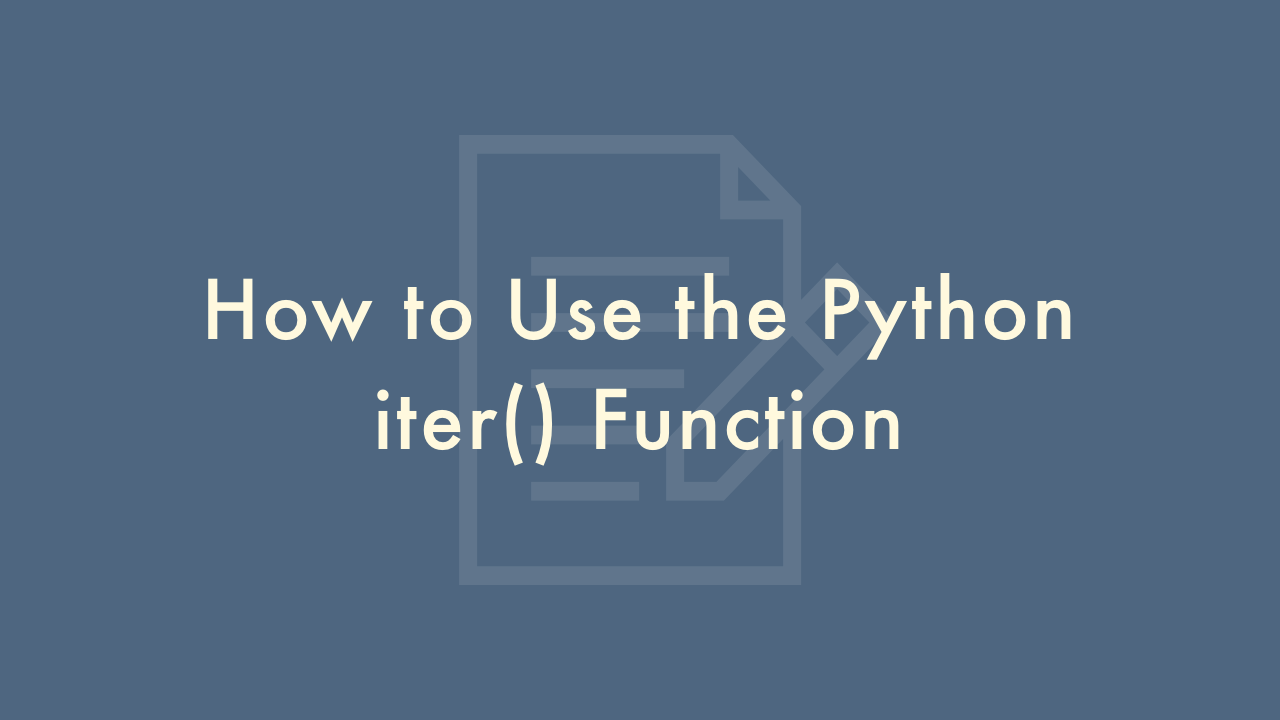
Contents
In this article, you will learn how to use the Python iter() function.
Python iter() Function
The iter() function in Python is a built-in function that returns an iterator object that can be used to iterate over an iterable object such as a list, tuple, string, dictionary, or set. The iter() function takes an iterable object as an argument and returns an iterator object that you can use to iterate over the iterable.
Here’s an example of how to use the iter() function:
my_list = [1, 2, 3, 4, 5]
my_iterator = iter(my_list)
for item in my_iterator:
print(item)
In this example, we create a list called my_list containing the integers from 1 to 5. We then create an iterator object called my_iterator by calling the iter() function with my_list as its argument. Finally, we use a for loop to iterate over the elements of my_iterator and print them one by one.
Here’s another example of how to use the iter() function with a custom iterable object:
class MyIterable:
def __init__(self, data):
self.data = data
def __iter__(self):
self.index = 0
return self
def __next__(self):
if self.index >= len(self.data):
raise StopIteration
result = self.data[self.index]
self.index += 1
return result
my_iterable = MyIterable([1, 2, 3, 4, 5])
my_iterator = iter(my_iterable)
for item in my_iterator:
print(item)
In this example, we define a custom iterable object called MyIterable that has a __iter__() method that returns itself as an iterator object and a __next__() method that returns the next element in the iterable. We then create an instance of MyIterable called my_iterable and create an iterator object called my_iterator by calling the iter() function with my_iterable as its argument. Finally, we use a for loop to iterate over the elements of my_iterator and print them one by one.