How to Replace Strings with Regex in Python
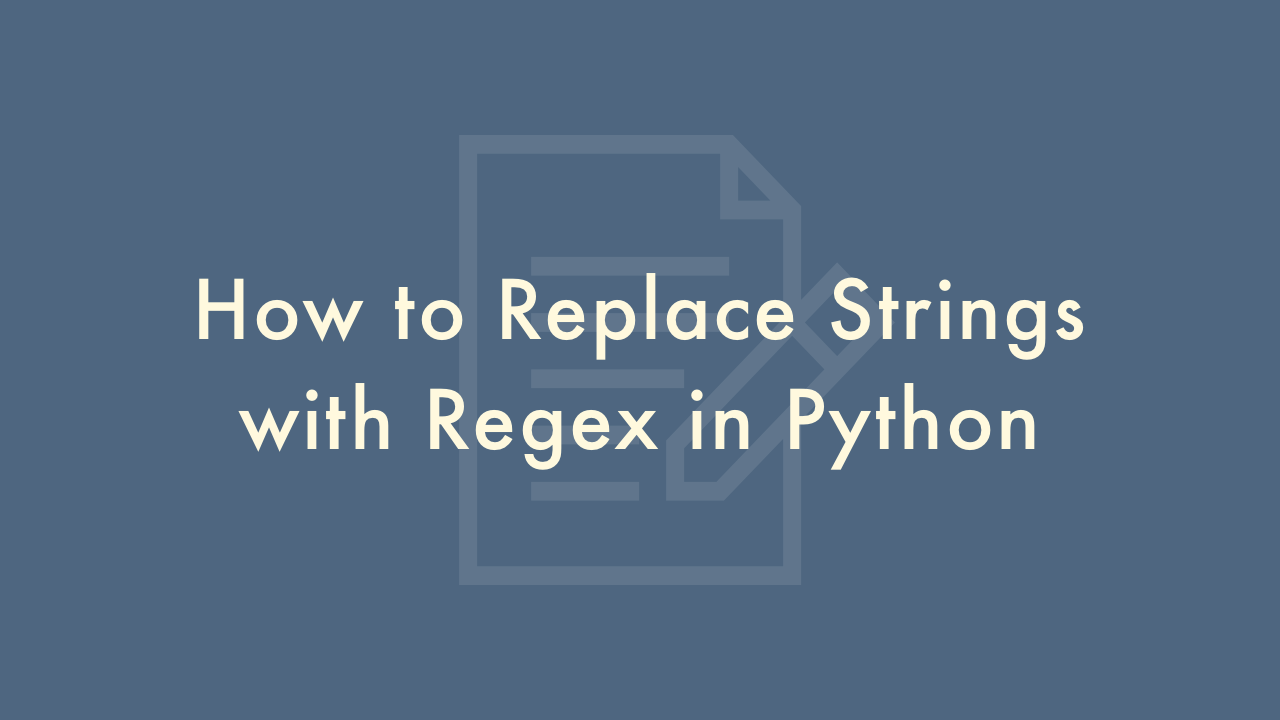
Contents
In this article, you will learn how to replace strings with regex in Python.
Replace Strings with Regex
You can replace strings in Python using the re
module, which provides functions for working with regular expressions.
Here is an example of how you can use the re.sub() function to replace a pattern in a string:
import re
text = "The cat is black."
new_text = re.sub("cat", "dog", text)
print(new_text)
# Output
# The dog is black.
The first argument to re.sub() is the pattern you want to replace, which in this case is the word “cat”. The second argument is the string to replace the pattern with, which is “dog”. The third argument is the input string.
You can also use regular expressions to define the pattern. For example:
text = "The number is 123."
new_text = re.sub("\d+", "999", text)
print(new_text)
# Output
# The number is 999.
In this case, the pattern \d+ matches one or more digits, so it replaces the number “123” with “999”.
Here are some additional details and tips for using the re.sub() function:
-
You can use capture groups to only replace a portion of the match. For example:
text = "The number is 123 and the date is 2020-01-01." new_text = re.sub("(\d+)-(\d+)-(\d+)", "\\2/\\3/\\1", text) print(new_text) # Output # The number is 123 and the date is 01/01/2020.
In this case, the pattern (\d+)-(\d+)-(\d+) matches dates in the format “YYYY-MM-DD”. The \\1, \\2, and \\3 in the replacement string refer to the captured groups, so the date is transformed to the format “MM/DD/YYYY”.
-
The re.sub() function replaces all occurrences of the pattern by default. If you only want to replace the first occurrence, you can specify the count parameter. For example:
text = "The cat is black and the cat is sleepy." new_text = re.sub("cat", "dog", text, count=1) print(new_text) # Output # The dog is black and the cat is sleepy.
-
The re.sub() function returns a new string with the replacements. If you want to modify the original string in-place, you need to assign the result back to the original string. For example:
text = "The cat is black." text = re.sub("cat", "dog", text) print(text) # Output # The dog is black.