How to Inverse a Matrix in NumPy
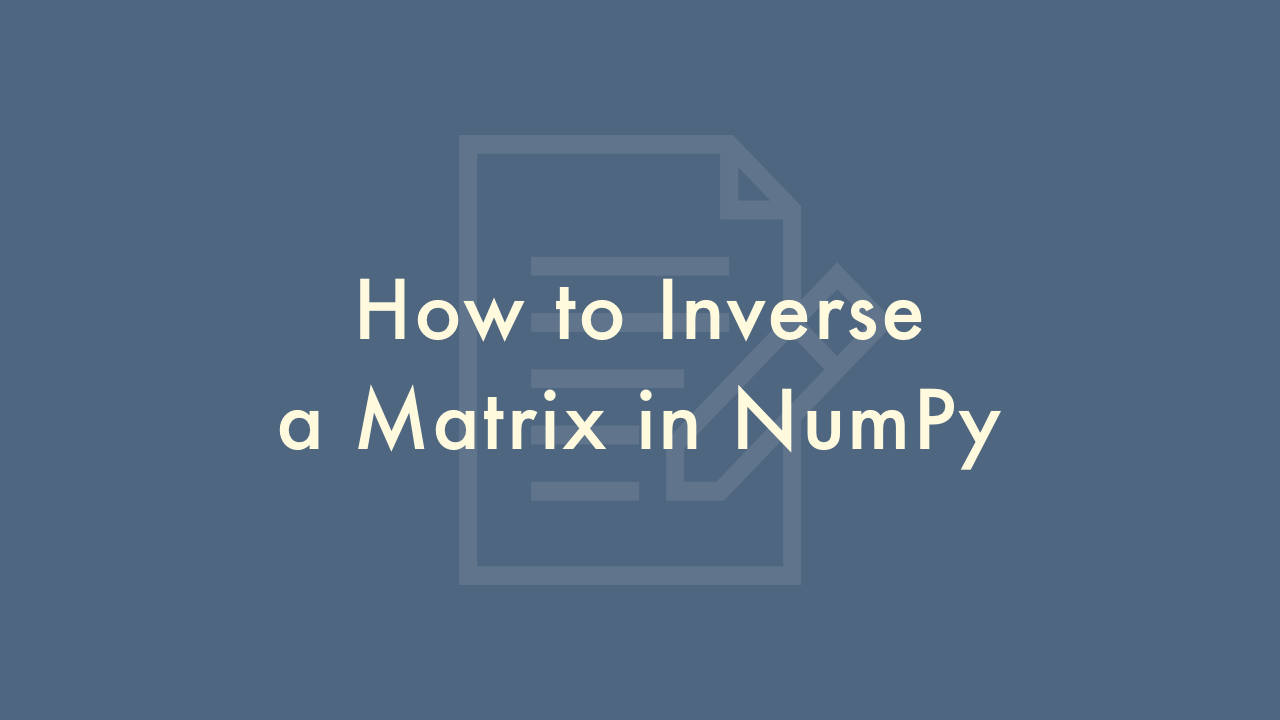
Contents
In this article, you will learn how to inverse a matrix in NumPy.
The numpy.linalg.inv function
The NumPy library provides the numpy.linalg.inv function to calculate the inverse of a matrix.
Here is an example of how to use it:
import numpy as np
A = np.array([[1, 2], [3, 4]])
A_inv = np.linalg.inv(A)
print("A: \n", A)
print("A_inv: \n", A_inv)
The output will be:
A:
[[1 2]
[3 4]]
A_inv:
[[-2. 1. ]
[ 1.5 -0.5]]
In this example, A is a 2×2 matrix and A_inv is its inverse. Note that A_inv is also a 2×2 matrix.
It’s important to keep in mind that not all matrices have an inverse. A matrix is only invertible if its determinant is not equal to zero. If a matrix is not invertible, the numpy.linalg.inv function will raise a LinAlgError.
import numpy as np
A = np.array([[1, 1], [1, 1]])
try:
A_inv = np.linalg.inv(A)
print("A_inv: \n", A_inv)
except np.linalg.LinAlgError as e:
print("Error: Matrix is not invertible.")
In mathematics, the inverse of a square matrix A is a matrix A^-1 such that AA^-1 = A^-1A = I, where I is the identity matrix. The identity matrix is a square matrix with ones on the main diagonal and zeros elsewhere. The identity matrix has the property that it doesn’t change any vector when multiplied by it.
In NumPy, the numpy.linalg.inv function calculates the inverse of a matrix using various methods based on the properties of the input matrix. In general, the function uses an LU decomposition-based method for finding the inverse of a matrix.
If the input matrix is singular (i.e., its determinant is equal to zero), then it does not have an inverse and the numpy.linalg.inv function will raise a LinAlgError exception.
It’s worth mentioning that the inverse of a matrix is not always unique, but for most matrices in practical applications, the inverse exists and is unique.