How to Read and Write CSV Files in Python
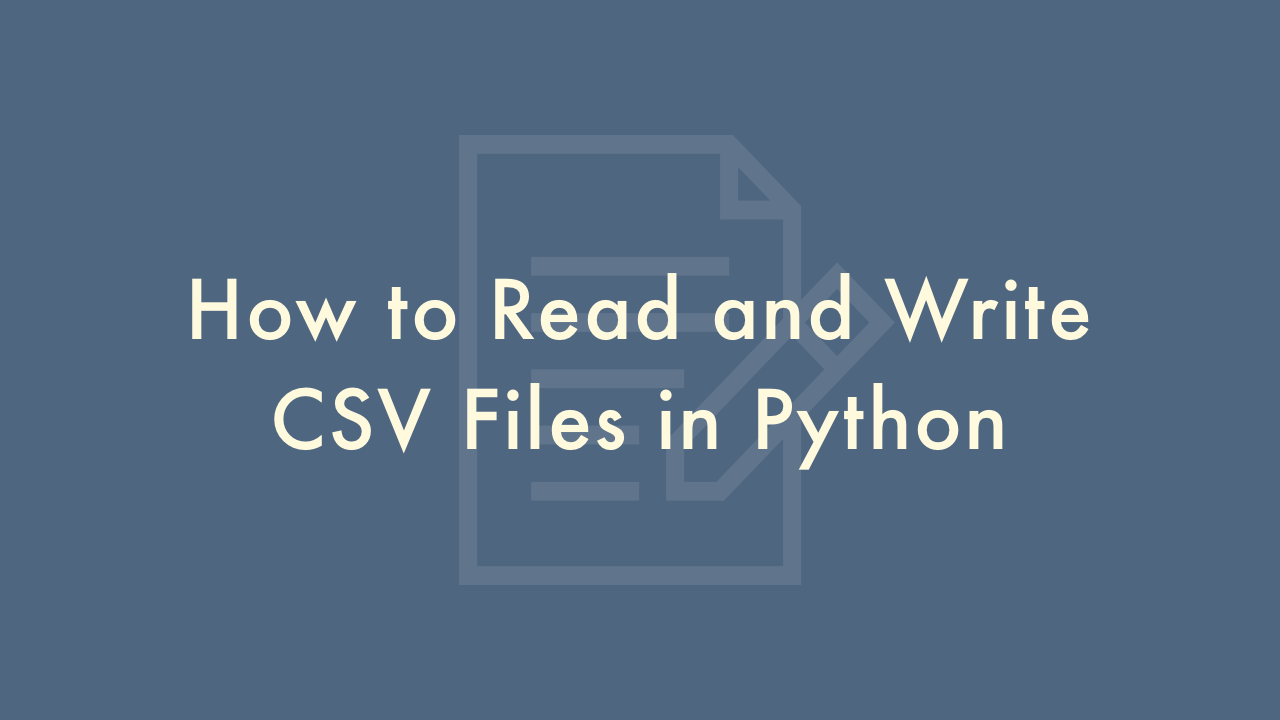
Contents
In this article, you will learn how to read and write CSV files in Python.
Reading and writing CSV files in Python
Reading and writing CSV files in Python can be done using the built-in “csv” module.
Here’s a brief example on how to do this:
Reading a CSV file
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
In this example, file.csv is the name of the CSV file you want to read. The with statement is used to ensure that the file is properly closed after reading it, even if an error occurs. The csv.reader function is used to create a reader object, which can be iterated over to access the rows of the CSV file. Each row is returned as a list of strings, where each string is a cell in the row.
Reading a CSV file with custom delimiter and quote character
By default, the csv.reader function uses a comma as the delimiter and double quotes as the quote character, but these can be changed using the delimiter and quotechar parameters, respectively.
For example:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file, delimiter='\t', quotechar="'")
for row in reader:
print(row)
In this example, the delimiter is changed to a tab character (‘\t’) and the quote character is changed to a single quote (“‘”).
Reading a CSV file with headers
If the CSV file has a header row, you can use the csv.DictReader class to read the file into a list of dictionaries, where each dictionary represents a row in the CSV file and the keys are the header names.
For example:
import csv
with open('file.csv', 'r') as file:
reader = csv.DictReader(file)
for row in reader:
print(row)
In this example, each row is returned as a dictionary where the keys are the header names and the values are the corresponding cell values.
Writing a CSV file
import csv
header = ['Column 1', 'Column 2', 'Column 3']
rows = [['Row 1, Column 1', 'Row 1, Column 2', 'Row 1, Column 3'],
['Row 2, Column 1', 'Row 2, Column 2', 'Row 2, Column 3'],
['Row 3, Column 1', 'Row 3, Column 2', 'Row 3, Column 3']]
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(header)
writer.writerows(rows)
In this example, header is a list of strings that represents the header row of the CSV file. rows is a list of lists, where each inner list represents a row in the CSV file. The with statement is used to ensure that the file is properly closed after writing to it, even if an error occurs. The csv.writer function is used to create a writer object, which can be used to write to the CSV file. The writerow method is used to write a single row to the CSV file, while the writerows method is used to write multiple rows at once.
Writing a CSV file with custom delimiter and quote character
The csv.writer class also has parameters delimiter and quotechar that can be used to specify the delimiter and quote character, respectively.
For example:
import csv
header = ['Column 1', 'Column 2', 'Column 3']
rows = [['Row 1, Column 1', 'Row 1, Column 2', 'Row 1, Column 3'],
['Row 2, Column 1', 'Row 2, Column 2', 'Row 2, Column 3'],
['Row 3, Column 1', 'Row 3, Column 2', 'Row 3, Column 3']]
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file, delimiter='\t', quotechar="'", quoting=csv.QUOTE_MINIMAL)
writer.writerow(header)
writer.writerows(rows)
In this example, the delimiter is changed to a tab character (‘\t’) and the quote character is changed to a single quote (“‘”). The quoting parameter is set to csv.QUOTE_MINIMAL, which means that only cells that contain the delimiter or quote character will be quoted.