How to Implement Asynchronous Programming in Python
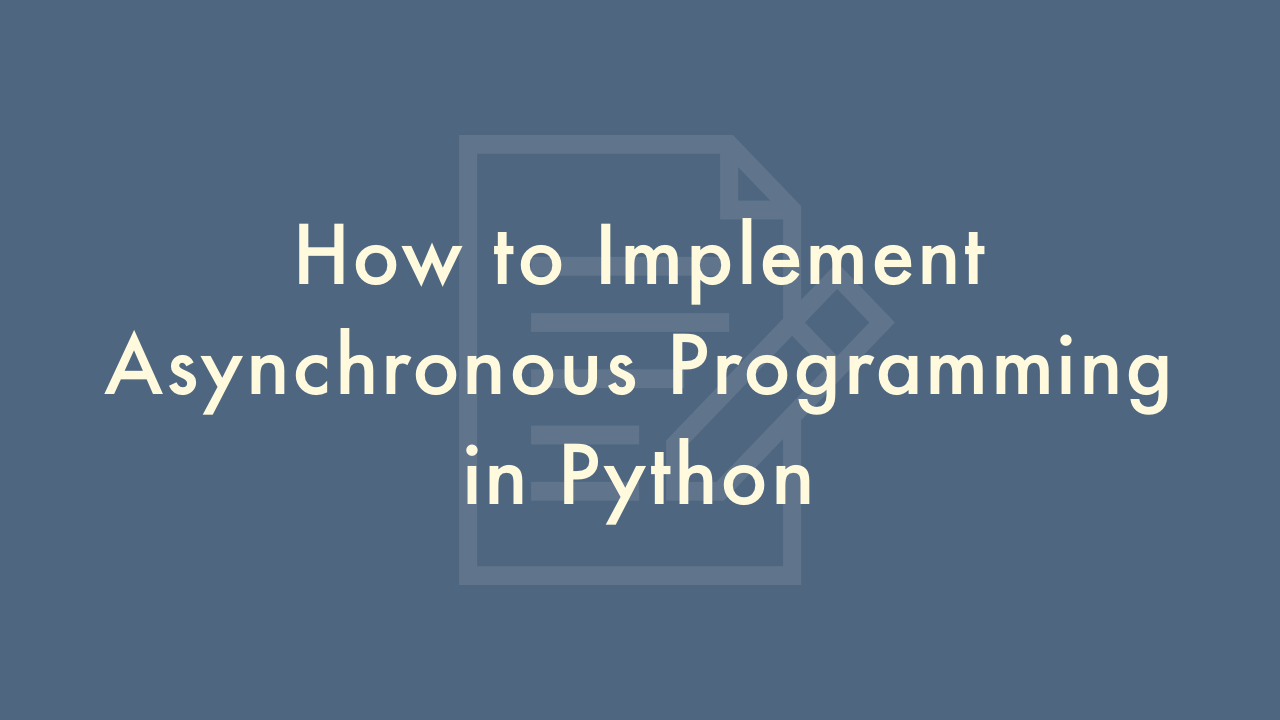
Contents
In this article, you will learn how to implement asynchronous programming in Python.
Asynchronous Programming in Python
Asynchronous programming is a style of programming where tasks can run concurrently without blocking each other. In Python, you can implement asynchronous programming using the asyncio library. Here’s an overview of how to do it:
Import the asyncio library:
import asyncio
Define your asynchronous function using the async def syntax:
async def my_coroutine():
# do something asynchronous
Create an event loop:
loop = asyncio.get_event_loop()
Add your coroutine to the event loop using the create_task() method:
task = loop.create_task(my_coroutine())
Run the event loop using the run_until_complete() method:
loop.run_until_complete(task)
This will run your asynchronous function and wait for it to complete. You can also run multiple coroutines concurrently by adding them to the event loop using create_task() and then running the event loop using run_until_complete().
Here’s an example of running two coroutines concurrently:
import asyncio
async def coroutine_one():
print("Coroutine One started")
await asyncio.sleep(1)
print("Coroutine One finished")
async def coroutine_two():
print("Coroutine Two started")
await asyncio.sleep(2)
print("Coroutine Two finished")
loop = asyncio.get_event_loop()
task_one = loop.create_task(coroutine_one())
task_two = loop.create_task(coroutine_two())
loop.run_until_complete(asyncio.gather(task_one, task_two))
This will output:
Coroutine One started
Coroutine Two started
Coroutine One finished
Coroutine Two finished
Note that in this example, we used the asyncio.gather() method to run both coroutines concurrently. This method takes a list of tasks and runs them concurrently, waiting for all of them to complete before returning.