How to Use Python netaddr Module
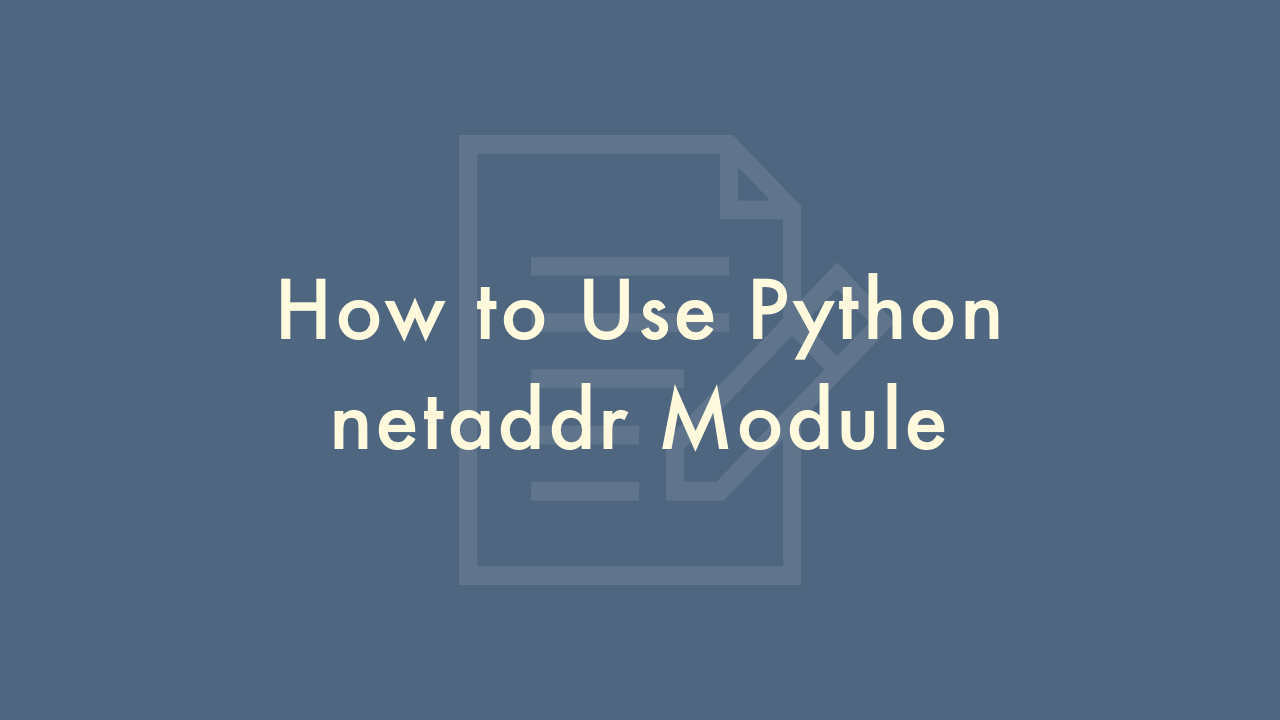
Contents
In this article, you will learn how to use Python netaddr module.
Python netaddr Module
The netaddr module is a powerful Python library that provides tools for working with network addresses and IP addresses. It can be used to perform common networking tasks such as subnetting, IP address manipulation, and network testing. Here’s how to use netaddr module in Python:
Installing netaddr:
To use netaddr, you first need to install it. You can do this using pip, the Python package manager, by running the following command in your terminal or command prompt:
pip install netaddr
Importing the module:
Once you have installed the netaddr module, you can start using it in your Python code. To do this, you need to import the module using the import statement:
import netaddr
Creating IP addresses:
The netaddr module provides a class called IPAddress that can be used to create IP addresses. You can create an IPAddress object by passing a string containing an IP address to the class constructor:
ip = netaddr.IPAddress('192.0.2.1')
Creating network addresses:
In addition to IP addresses, the netaddr module also provides a class called IPNetwork that can be used to create network addresses. You can create an IPNetwork object by passing a string containing a CIDR notation to the class constructor:
network = netaddr.IPNetwork('192.0.2.0/24')
Checking if an IP address is in a network:
You can use the in operator to check if an IPAddress object is in an IPNetwork object:
if ip in network:
print('IP address is in network')
else:
print('IP address is not in network')
Performing subnetting:
The IPNetwork class provides several methods for performing subnetting. For example, you can use the subnet method to split a network into smaller subnets:
subnets = network.subnet(28)
for subnet in subnets:
print(subnet)
Converting IP addresses to different formats:
The IPAddress class provides several methods for converting IP addresses to different formats. For example, you can use the packed method to get the IP address as a packed binary string:
packed_ip = ip.packed
Converting network addresses to different formats:
The IPNetwork class also provides several methods for converting network addresses to different formats. For example, you can use the cidr method to get the network address in CIDR notation:
cidr_network = network.cidr