Python String Interpolation
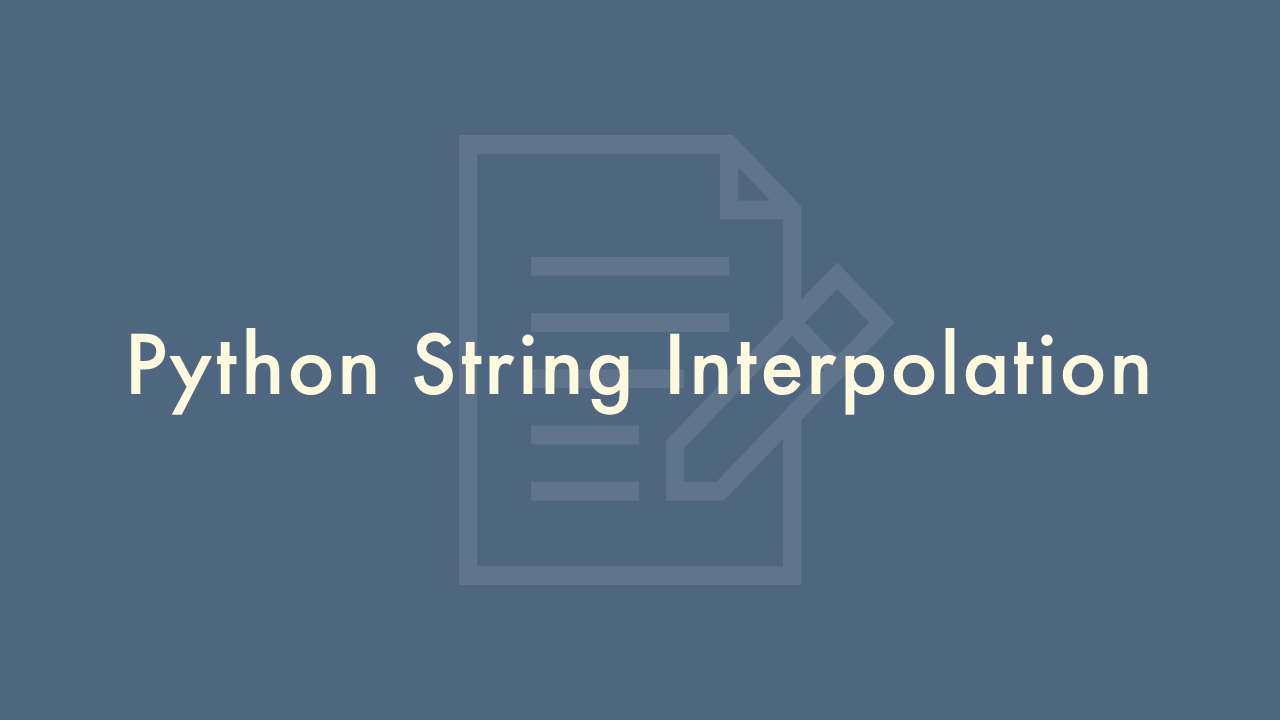
Contents
In this article, you will learn about string interpolation in Python.
Python String Interpolation
String interpolation is a process of evaluating expressions and substituting their values into a string. In Python, this can be achieved in several ways, including:
Concatenation
You can concatenate strings using the “+” operator. This is the simplest method to interpolate values into a string. You simply use the “+” operator to join different strings and variables.
For example:
name = "John"
age = 30
print("My name is " + name + " and I am " + str(age) + " years old.")
However, this method can become tedious if you have many variables to substitute into a string, as you need to manually convert them to strings using the str() function.
Format Method
You can use the format() method to substitute values into a string.
For example:
name = "John"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
The curly braces {} are placeholders that are replaced with the values passed as arguments to the format() method.
f-strings (Formatted string literals)
In Python 3.6 and later, you can use f-strings to embed expressions inside string literals, prefixed with “f”. f-strings are more readable and allow you to embed expressions directly within the string, without having to use the str() function to convert values to strings.
For example:
name = "John"
age = 30
print(f"My name is {name} and I am {age} years old.")
All of these methods are used to insert values into strings. The choice of which one to use depends on your personal preference, but f-strings are considered to be the most readable and are generally recommended for new projects.