How to Use the Matplotlib.pyplot.plot() Function in Python
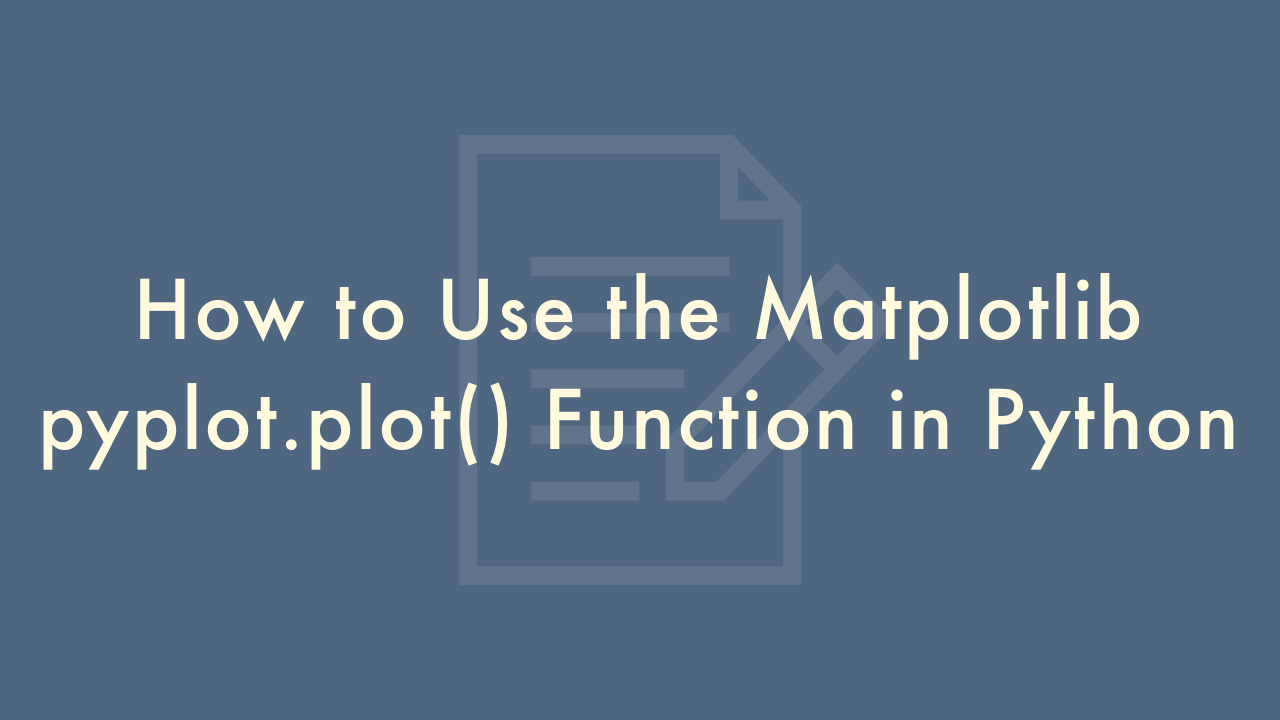
Contents
In this article, you will learn how to use the Matplotlib.pyplot.plot() function in Python.
The Matplotlib.pyplot.plot() Function
The plot() function is one of the most commonly used functions in the pyplot module, and it allows you to create line plots.
Syntax
The basic syntax of the plot() function is as follows:
plt.plot(x, y, format_string, **kwargs)
Parameters
x
andy
are the data sets to be plotted. They can be arrays, lists, or other sequences of data.format_string
is a string that specifies the color, line style, and marker style of the plot. For example, ‘ro’ specifies a red circle marker, and ‘b-‘ specifies a blue solid line. If format_string is not specified, the plot will be a blue solid line by default.**kwargs
are optional keyword arguments that can be used to customize the plot. For example, linewidth can be used to set the width of the line, and label can be used to provide a label for the plot.
Examples
Here’s a simple example that plots a line:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [1, 4, 9, 16]
plt.plot(x, y)
plt.show()
In this example, we first import the pyplot module from the matplotlib library. We then define two lists, x and y, that represent the data we want to plot. Finally, we call the plot() function with x and y as arguments, and then call the show() function to display the plot.
Here’s another example that demonstrates some of the formatting options:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [1, 4, 9, 16]
plt.plot(x, y, 'ro-', linewidth=2, label='Squares')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Square Numbers')
plt.legend()
plt.show()
In this example, we use the format_string argument to specify a red circle marker (‘ro’) and a solid line (‘-‘). We also set the linewidth to 2 and provide a label for the plot. We then use the xlabel(), ylabel(), and title() functions to add labels to the X and Y axes and a title to the plot. Finally, we use the legend() function to display the label.
The plot() function can also accept multiple data sets and plot them on the same graph. Here’s an example:
import matplotlib.pyplot as plt
x1 = [1, 2, 3, 4]
y1 = [1, 4, 9, 16]
x2 = [1, 2, 3, 4]
y2 = [2, 4, 6, 8]
plt.plot(x1, y1, 'ro-', label='Squares')
plt.plot(x2, y2, 'b^-', label='Doubles')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Square and Double Numbers')
plt.legend()
plt.show()
In this example, we define two sets of data, x1 and y1, and x2 and y2. We then call the plot() function twice with different data sets and format strings to plot both sets of data on the same graph. We also provide labels for each plot using the label argument. Finally, we call the legend() function to display the labels.
The plot() function can also accept a third argument, which specifies the type of plot to be created. For example, plt.plot(x, y, ‘o’) will create a scatter plot with circles as markers.
In addition to line plots and scatter plots, the plot() function can also create other types of plots such as bar plots, histogram plots, and pie charts.