How to Copy a File and Folder in Python
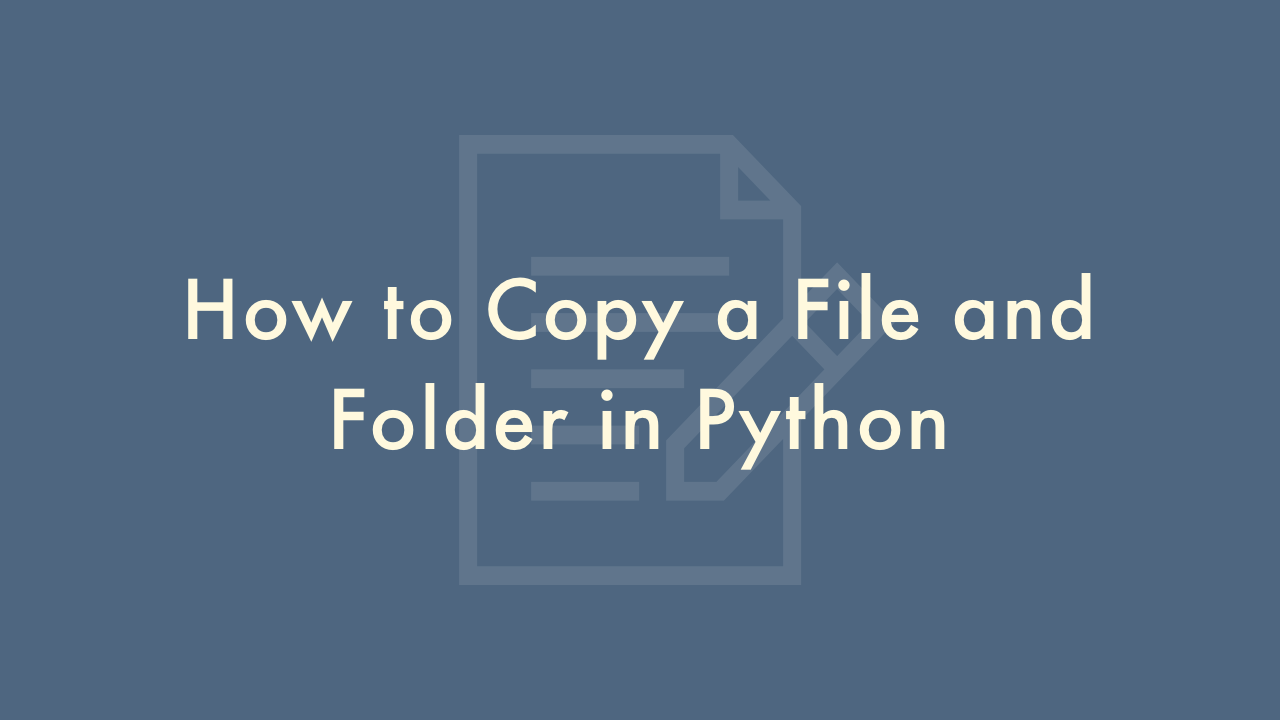
09/14/2021
Contents
In this article, you will learn how to copy a file and folder in Python.
Copy a file and folder
To copy a file or folder in Python, you can use the built-in shutil module, which provides functions for copying files and directories. Here are the basic steps for copying a file or folder in Python:
- Import the shutil module.
- Use the shutil.copy() function to copy a file from one location to another. The function takes two arguments: the source file path and the destination file path.
- Use the shutil.copytree() function to copy a folder and all its contents to a new location. The function takes two arguments: the source folder path and the destination folder path.
Here’s an example of how to copy a file in Python:
import shutil
# Copy a file from source to destination
src_file = 'path/to/source/file.txt'
dst_file = 'path/to/destination/file.txt'
shutil.copy(src_file, dst_file)
And here’s an example of how to copy a folder in Python:
import shutil
# Copy a folder from source to destination
src_folder = 'path/to/source/folder'
dst_folder = 'path/to/destination/folder'
shutil.copytree(src_folder, dst_folder)
Here are some more details about copying files and folders in Python using the shutil module:
shutil.copy(src, dst, *, follow_symlinks=True)
: This function is used to copy a file from the source location to the destination location. If the destination already exists, it will be replaced. By default, this function follows symbolic links, but you can disable this behavior by setting follow_symlinks to False.shutil.copy2(src, dst, *, follow_symlinks=True)
: This function is similar to shutil.copy(), but it also preserves the file’s metadata, such as its creation and modification times.shutil.copytree(src, dst, symlinks=False, ignore=None, copy_function=copy2, ignore_dangling_symlinks=False)
: This function is used to copy a folder and all its contents from the source location to the destination location. The symlinks argument controls whether symbolic links are copied as links or as the contents of the linked file. The ignore argument is a function that can be used to filter out files or directories that you don’t want to copy. The copy_function argument specifies the function used to copy individual files, and by default it is set to shutil.copy2(). The ignore_dangling_symlinks argument determines whether to ignore or raise an error for symbolic links that point to non-existent files.shutil.copytree() can raise exceptions
: If the destination already exists, shutil.copytree() will raise an FileExistsError exception. If the source folder doesn’t exist or is not a folder, it will raise a FileNotFoundError exception.
Here’s an example of how to copy a file and preserve its metadata:
import shutil
# Copy a file and preserve its metadata
src_file = 'path/to/source/file.txt'
dst_file = 'path/to/destination/file.txt'
shutil.copy2(src_file, dst_file)
And here’s an example of how to copy a folder and exclude files that end with ‘.log’:
import os
import shutil
# Copy a folder and exclude files that end with '.log'
def exclude_logs(dir, files):
return [f for f in files if f.endswith('.log')]
src_folder = 'path/to/source/folder'
dst_folder = 'path/to/destination/folder'
shutil.copytree(src_folder, dst_folder, ignore=exclude_logs)