How to Use the Matplotlib.pyplot.show() Function in Python
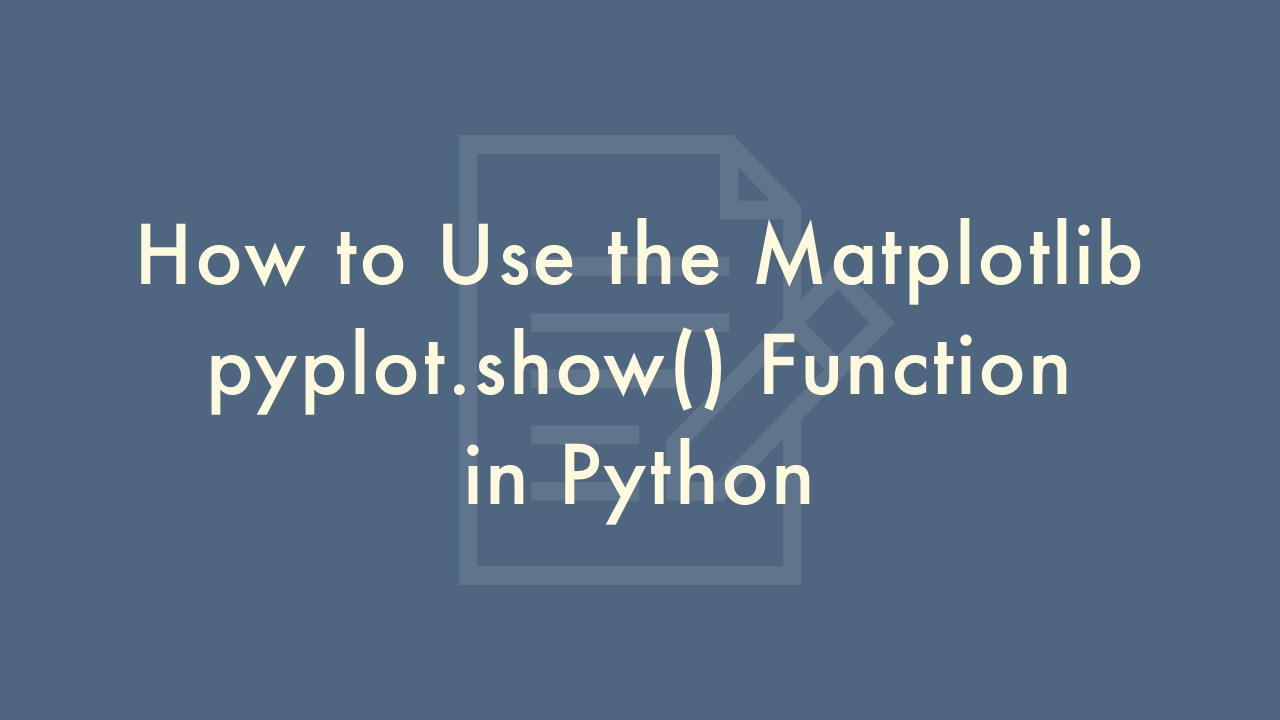
Contents
In this article, you will learn how to use the Matplotlib.pyplot.show() function in Python.
The Matplotlib.pyplot.show() Function
The show() function is a method of the pyplot module of the Matplotlib library. This function is used to display the plot created using Matplotlib. The show() function opens a new window and displays the plot in it. The plot can then be interacted with, such as zooming in and out, panning, and saving the plot as an image.
Syntax
The syntax of the show() function is straightforward. It doesn’t require any arguments.
import matplotlib.pyplot as plt
# create a plot
plt.plot(x, y)
# show the plot
plt.show()
In this code snippet, we first import the pyplot module of the Matplotlib library and create a plot using the plot() function. Then we use the show() function to display the plot.
Examples
Here is an example of creating and displaying a simple line plot using Matplotlib and the show() function.
import matplotlib.pyplot as plt
# create some data
x = [1, 2, 3, 4]
y = [10, 20, 30, 40]
# create a line plot
plt.plot(x, y)
# show the plot
plt.show()
When you run this code, it will display a window containing a line plot with four data points. You can interact with the plot by zooming in and out, panning, and saving the plot as an image.
Closing the plot window
After displaying the plot, you can close the window using the close() function. This function is a method of the pyplot module of the Matplotlib library.
import matplotlib.pyplot as plt
# create some data
x = [1, 2, 3, 4]
y = [10, 20, 30, 40]
# create a line plot
plt.plot(x, y)
# show the plot
plt.show()
# close the plot window
plt.close()
In this code snippet, we first create and display a line plot using the show() function. Then we close the plot window using the close() function.