How to Convert a String List to an Integer List in Python
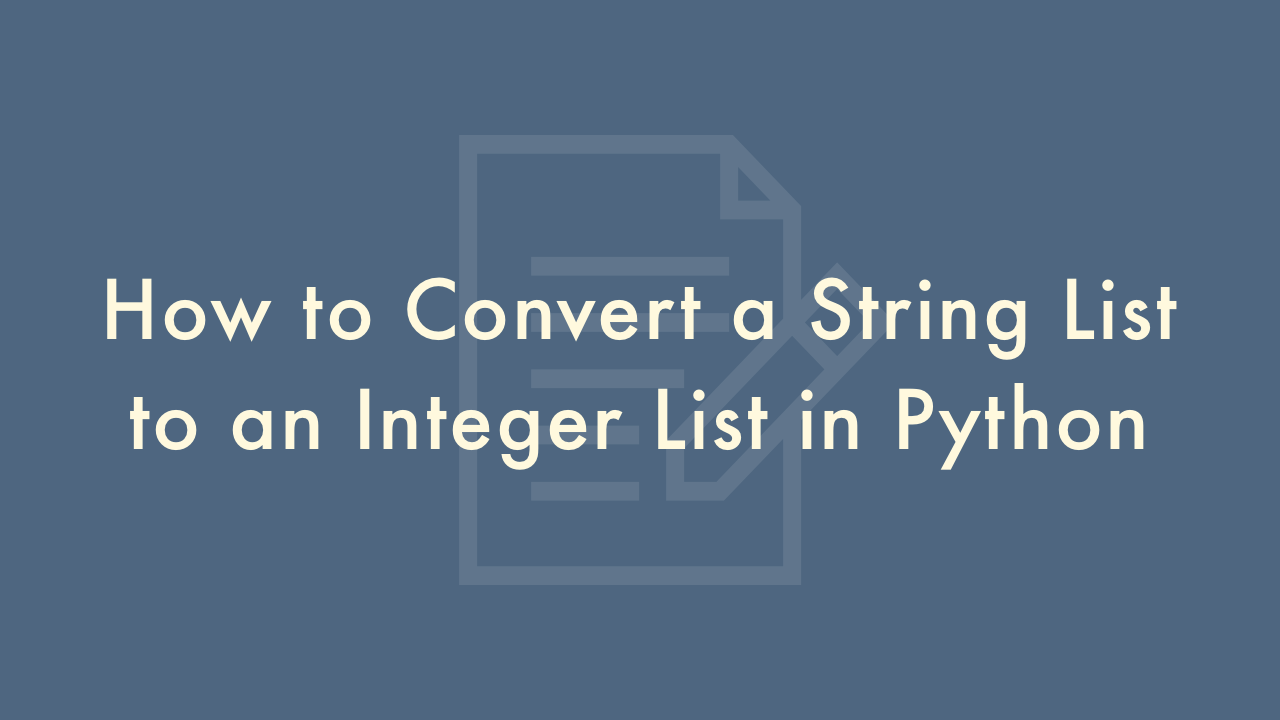
Contents
In this article, you will learn how to convert a string list to an integer list in Python.
Converting a String List to an Integer List
Using a list comprehension and the int() function
To convert a string list to an integer list in Python, you can use a list comprehension and the int() function to convert each string element into an integer. Here is an example:
string_list = ['1', '2', '3', '4', '5']
integer_list = [int(element) for element in string_list]
print(integer_list)
# Output:
# [1, 2, 3, 4, 5]
In the example, we create a list of strings called string_list. We then use a list comprehension to loop through each element in the list and convert it to an integer using the int() function. The resulting list of integers is stored in the variable integer_list. Finally, we print the contents of integer_list.
Using the map() function
string_list = ['1', '2', '3', '4', '5']
integer_list = list(map(int, string_list))
print(integer_list)
In this example, we use the map() function to apply the int() function to each element in the string_list. The resulting values are then passed to the list() function to create a new list of integers.
Using a loop:
string_list = ['1', '2', '3', '4', '5']
integer_list = []
for element in string_list:
integer_list.append(int(element))
print(integer_list)
Here, we use a for loop to iterate over each element in the string_list, and use the int() function to convert it to an integer. The resulting values are appended to a new list called integer_list.
No matter which method you choose, be sure to check that your string list contains only valid integers, otherwise the conversion will fail and result in a ValueError.