How to Compute Inner Product of Matrices in Python
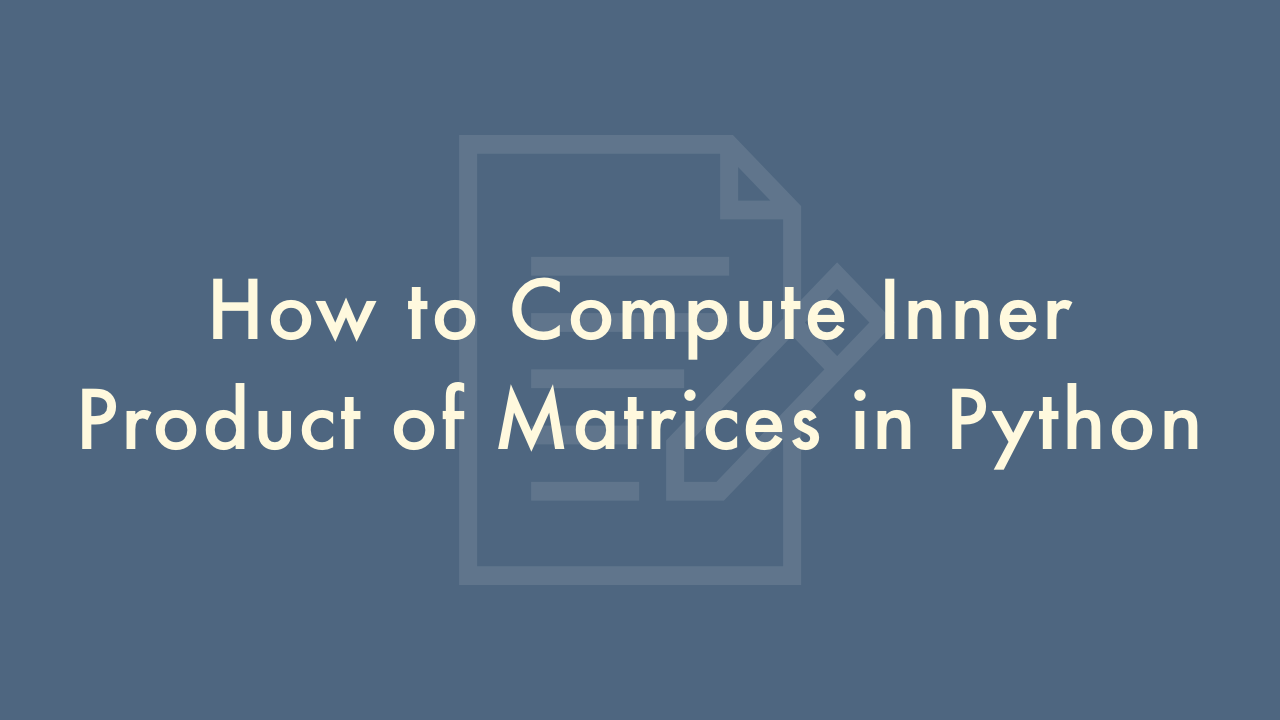
09/10/2021
Contents
In this article, you will learn how to compute inner product of matrices in Python.
Compute Inner Product of Matrices
The inner product of two matrices can be calculated in Python by using the dot product function from the NumPy library. The dot product function computes the dot product of two arrays and returns the scalar result. The arrays must have the same number of elements, and their shapes must be compatible for a dot product operation.
Here’s an example of how to compute the inner product of two matrices in Python:
import numpy as np
matrix_a = np.array([[1, 2], [3, 4]])
matrix_b = np.array([[5, 6], [7, 8]])
inner_product = np.dot(matrix_a, matrix_b)
print(inner_product)
This will output the following:
array([[19, 22],
[43, 50]])
Note that the dot product of two matrices is a matrix. If you want to calculate the inner product of two vectors (1-dimensional arrays), you can use the dot product function in the same way.