How to Connect Bluetooth in Python
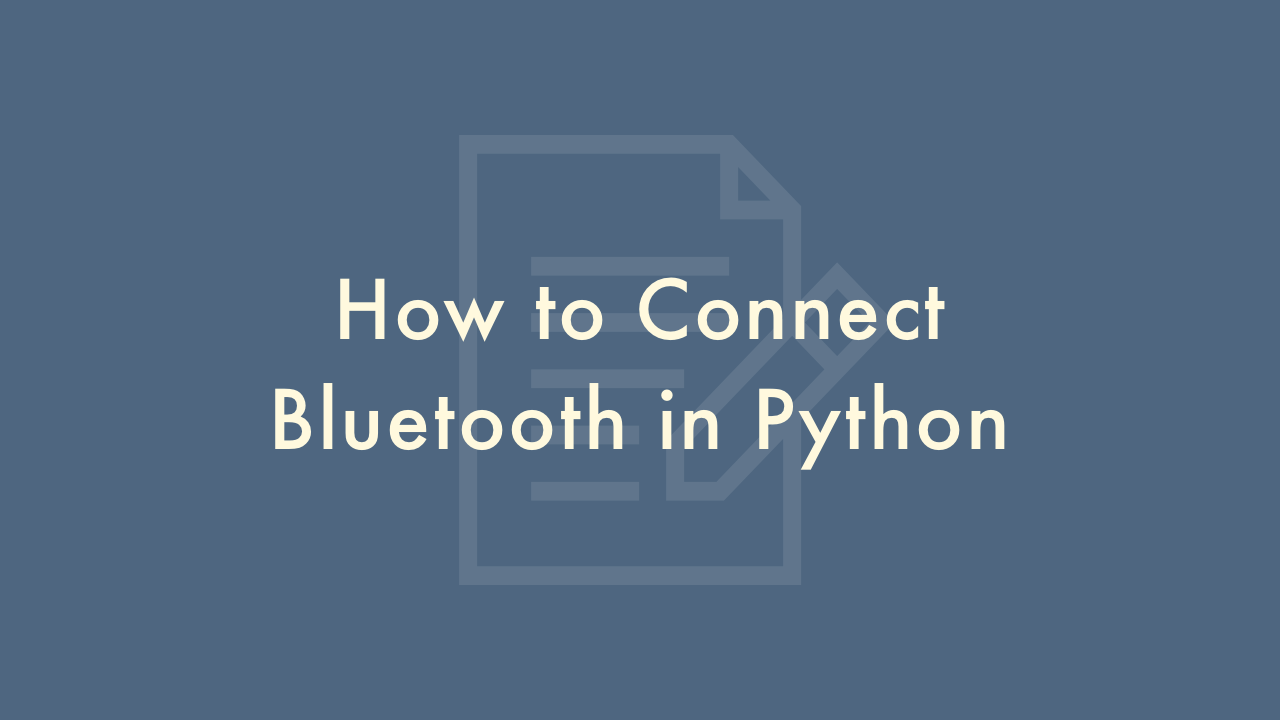
Contents
In this article, you will learn how to connect bluetooth in Python.
How to connect bluetooth
To connect Bluetooth in Python, you can use the PyBluez library which provides a Python interface to the Bluetooth protocol stack.
Here’s an example code that demonstrates how to connect to a Bluetooth device using PyBluez:
import bluetooth
# Set the MAC address of the Bluetooth device you want to connect to
target_address = 'XX:XX:XX:XX:XX:XX'
# Set the Bluetooth service UUID you want to connect to
# This will depend on the type of device you're connecting to
target_service = 'XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX'
# Search for nearby Bluetooth devices
nearby_devices = bluetooth.discover_devices()
# Loop through the nearby devices and try to connect to the target device
for address in nearby_devices:
if address == target_address:
# Try to connect to the target device
print(f"Connecting to {target_address}...")
try:
# Create a Bluetooth socket and connect to the target device
sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
sock.connect((target_address, 1))
# Send some data to the target device
sock.send("Hello, world!")
# Receive some data from the target device
data = sock.recv(1024)
print(f"Received: {data}")
# Close the socket
sock.close()
except:
# Failed to connect to the target device
print(f"Failed to connect to {target_address}")
pass
In the code above, replace target_address with the MAC address of the Bluetooth device you want to connect to, and replace target_service with the UUID of the Bluetooth service you want to connect to. You can find the UUID of the service in the device’s documentation.
Note that you’ll need to pair the target device with your computer before you can connect to it. You can do this using the operating system’s Bluetooth settings or using the PyBluez library.