How to Pack and Unpack Arguments in Python
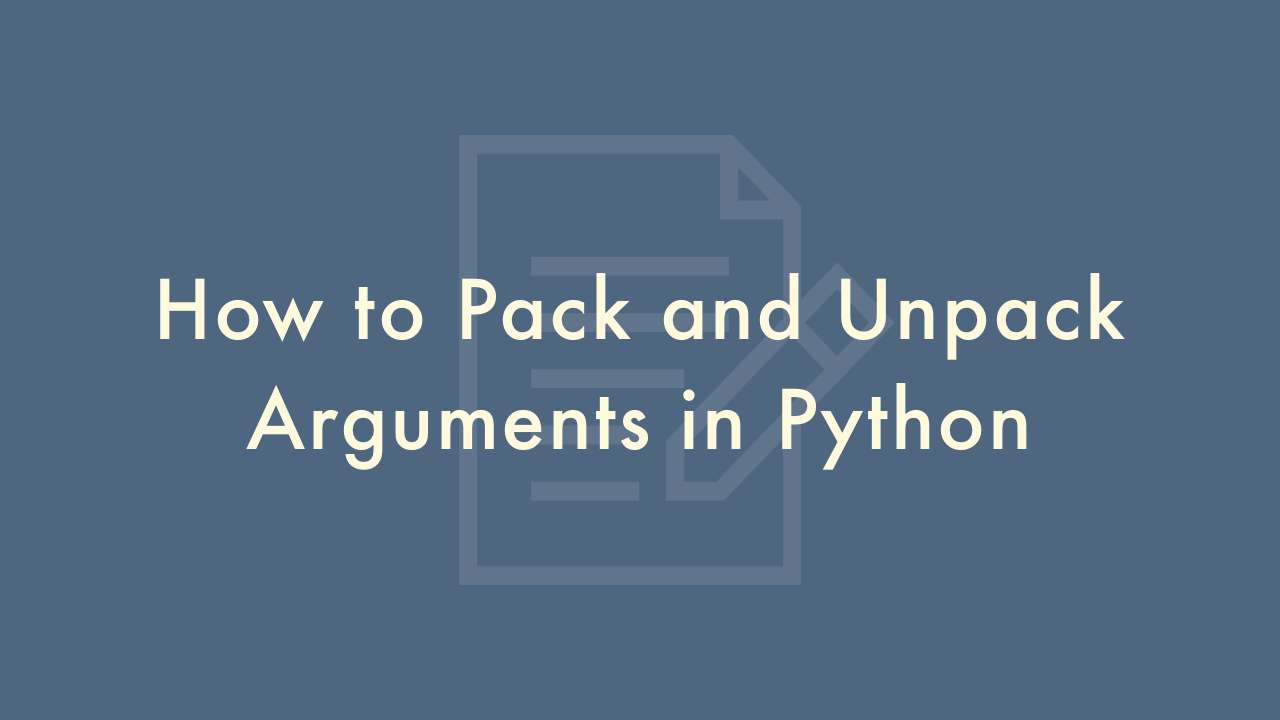
Contents
In this article, you will learn how to pack and unpack arguments in Python.
Pack and Unpack Arguments
In Python, packing and unpacking arguments can be achieved using the * (asterisk) operator. Here is an overview of how to use the * operator for packing and unpacking arguments:
Packing Arguments
Packing arguments means taking a list or tuple of values and packing them into a single variable. Here is an example of how to pack arguments using the * operator:
def my_function(*args):
for arg in args:
print(arg)
my_function(1, 2, 3)
In this example, the *args parameter in the my_function function indicates that any number of arguments can be passed to the function. When the function is called with my_function(1, 2, 3), the values 1, 2, and 3 are packed into a tuple and assigned to the args parameter.
Unpacking Arguments
Unpacking arguments means taking a sequence of values and unpacking them into individual variables. Here is an example of how to unpack arguments using the * operator:
my_list = [1, 2, 3]
def my_function(a, b, c):
print(a, b, c)
my_function(*my_list)
In this example, the *my_list expression in the function call unpacks the list [1, 2, 3] and assigns the first value to a, the second value to b, and the third value to c. The output of the function call is:
1 2 3
Note that the number of variables in the function parameter list must match the length of the sequence being unpacked, otherwise a TypeError will be raised.