How to Use the Python Image.fromarray() Function
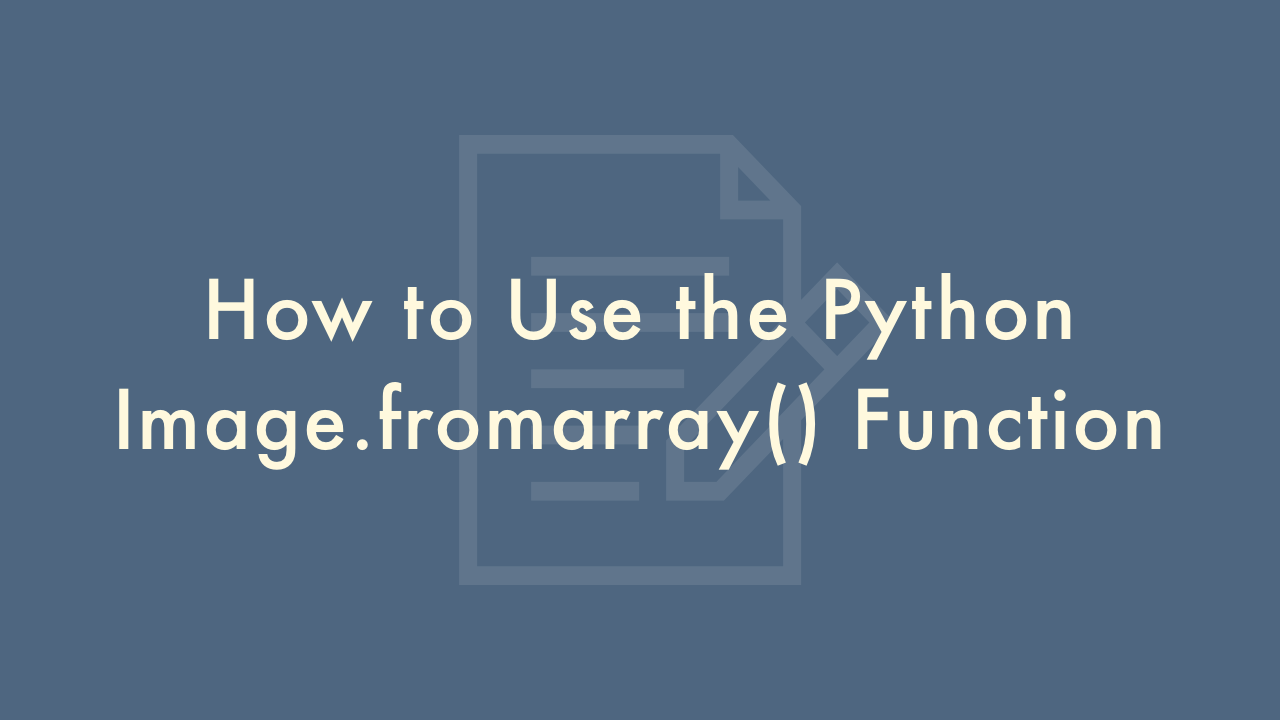
Contents
In this article, you will learn how to use the Image.fromarray() function in Python.
The Image.fromarray() Function
The Image.fromarray() function is a part of the Python Imaging Library (PIL) or the Pillow module, which is used for processing images in Python. This function is used to create an image object from a NumPy array.
The NumPy array can be a two-dimensional or three-dimensional array representing the image. A two-dimensional array represents a grayscale image, while a three-dimensional array represents a color image with three color channels (red, green, and blue).
Syntax
Here is the syntax of the Image.fromarray() function:
Image.fromarray(array, mode=None)
The array parameter is the NumPy array from which the image object is to be created. The mode parameter is an optional parameter that specifies the color mode of the image. If the mode parameter is not specified, the mode is inferred from the shape of the input array. If the input array is two-dimensional, the mode is ‘L’ (grayscale), and if it is three-dimensional, the mode is ‘RGB’.
Examples
Here is an example code that demonstrates the usage of the Image.fromarray() function:
import numpy as np
from PIL import Image
# Create a random grayscale image of size 256x256
array = np.random.randint(0, 256, size=(256, 256), dtype=np.uint8)
# Create an image object from the NumPy array
img = Image.fromarray(array)
# Display the image
img.show()
In this example, we first create a random grayscale image of size 256×256 using NumPy. Then, we create an image object from the NumPy array using the Image.fromarray() function. Finally, we display the image using the show() method of the image object.
Similarly, we can create a color image from a three-dimensional NumPy array representing the image. Here is an example code that demonstrates the usage of the Image.fromarray() function to create a color image:
import numpy as np
from PIL import Image
# Create a random color image of size 256x256
array = np.random.randint(0, 256, size=(256, 256, 3), dtype=np.uint8)
# Create an image object from the NumPy array
img = Image.fromarray(array)
# Display the image
img.show()
In this example, we first create a random color image of size 256×256 using NumPy. Then, we create an image object from the NumPy array using the Image.fromarray() function. Finally, we display the image using the show() method of the image object.