How to Use the Numpy zeros() Function in Python
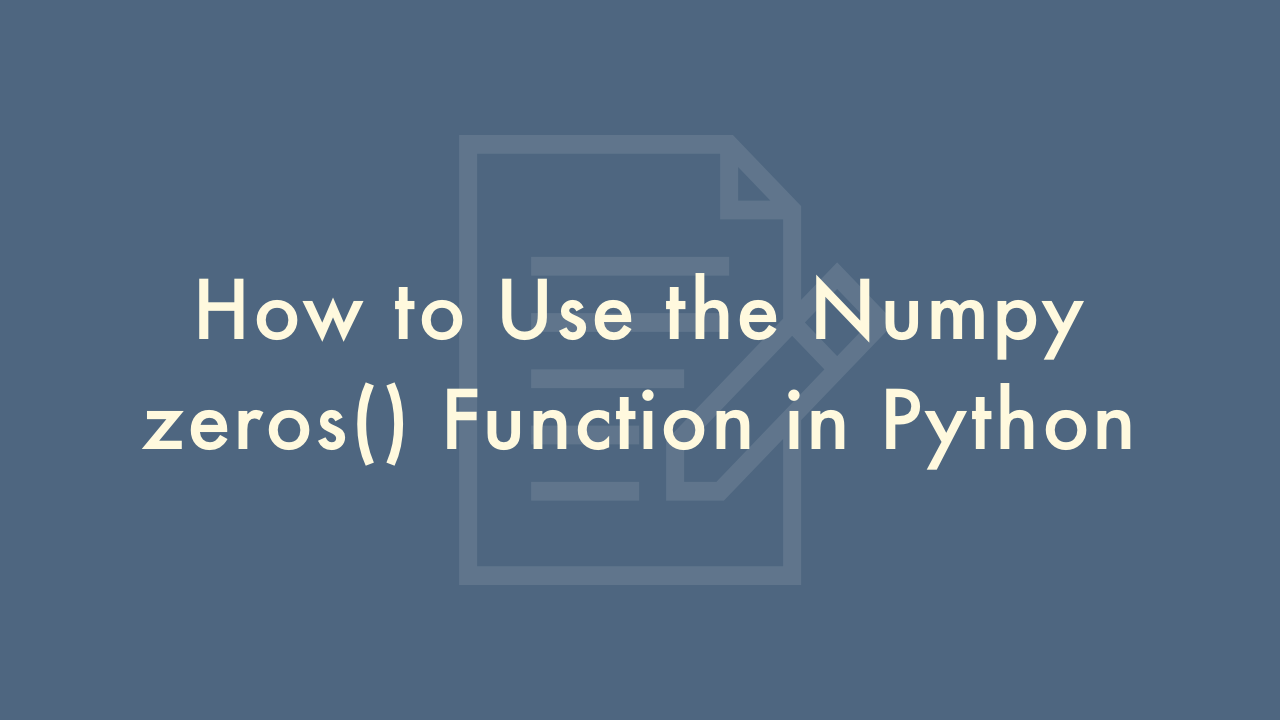
Contents
In this article, you will learn how to use the Numpy zeros() function in Python.
Numpy zeros() Function
The numpy.zeros() function is used to create a new array filled with zeros. This function is particularly useful when you need to create an array with a specific shape and initialize it with zeros. Here’s how to use the numpy.zeros() function in Python:
First, you need to import the numpy library:
import numpy as np
Then, you can use the numpy.zeros() function to create an array with a specific shape and data type:
a = np.zeros((3,4), dtype=int)
In this example, we create a 2-dimensional array with 3 rows and 4 columns, and set the data type to int. The resulting array a will look like this:
array([[0, 0, 0, 0],
[0, 0, 0, 0],
[0, 0, 0, 0]])
You can also create a 1-dimensional array by specifying the length of the array:
b = np.zeros(5)
This will create a 1-dimensional array of length 5 with default data type of float:
array([0., 0., 0., 0., 0.])
You can specify a different data type using the dtype parameter, like this:
c = np.zeros(5, dtype=int)
This will create a 1-dimensional array of length 5 with data type of int:
array([0, 0, 0, 0, 0])
That’s how you can use the numpy.zeros() function in Python to create arrays filled with zeros of a specified shape and data type.