How to Determine the Character Encoding of a File in Python
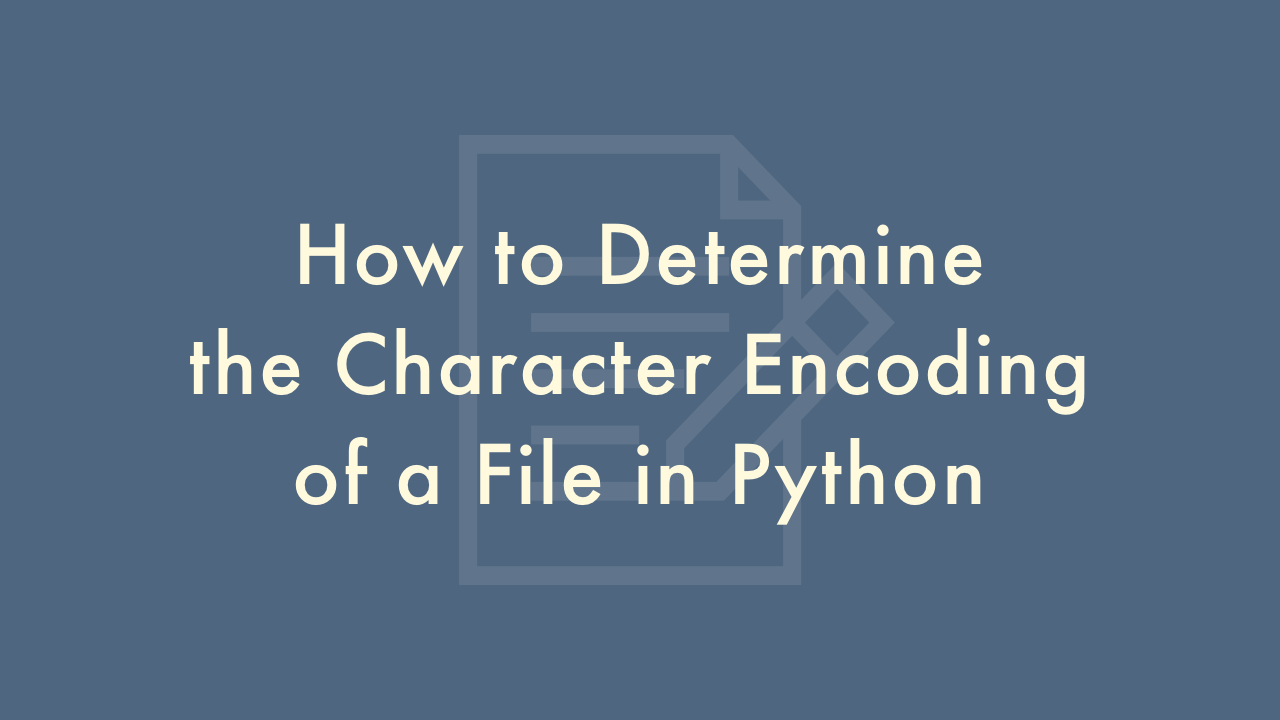
Contents
In this article, you will learn how to determine the character encoding of a file in Python.
Determine the character encoding of a file
To determine the character encoding of a file in Python, you can use the chardet library. This library uses statistical algorithms to determine the character encoding of a file.
Here’s an example on how to use chardet to determine the character encoding of a file:
import chardet
def get_encoding(file):
with open(file, 'rb') as f:
result = chardet.detect(f.read())
return result['encoding']
file = 'example.txt'
encoding = get_encoding(file)
print(f'The character encoding of {file} is {encoding}')
A character encoding is a system that maps characters in a character set (such as ASCII or Unicode) to unique numbers that can be used to represent the characters as bytes in a computer file or memory. When you open a file in Python, it’s important to know the character encoding of the file, because if you try to read the file using the wrong encoding, the characters in the file will be interpreted as incorrect or meaningless data.
In Python, you can use the open function to open a file and specify the encoding using the encoding parameter. For example:
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
print(content)
In this example, the open function is used to open the file example.txt in read mode (‘r’) and specify the encoding as utf-8. The with statement is used to ensure that the file is closed automatically when the block of code is finished. The contents of the file are then read into a string using the read method, and printed to the console.