How to Solve a System of Equations in Python
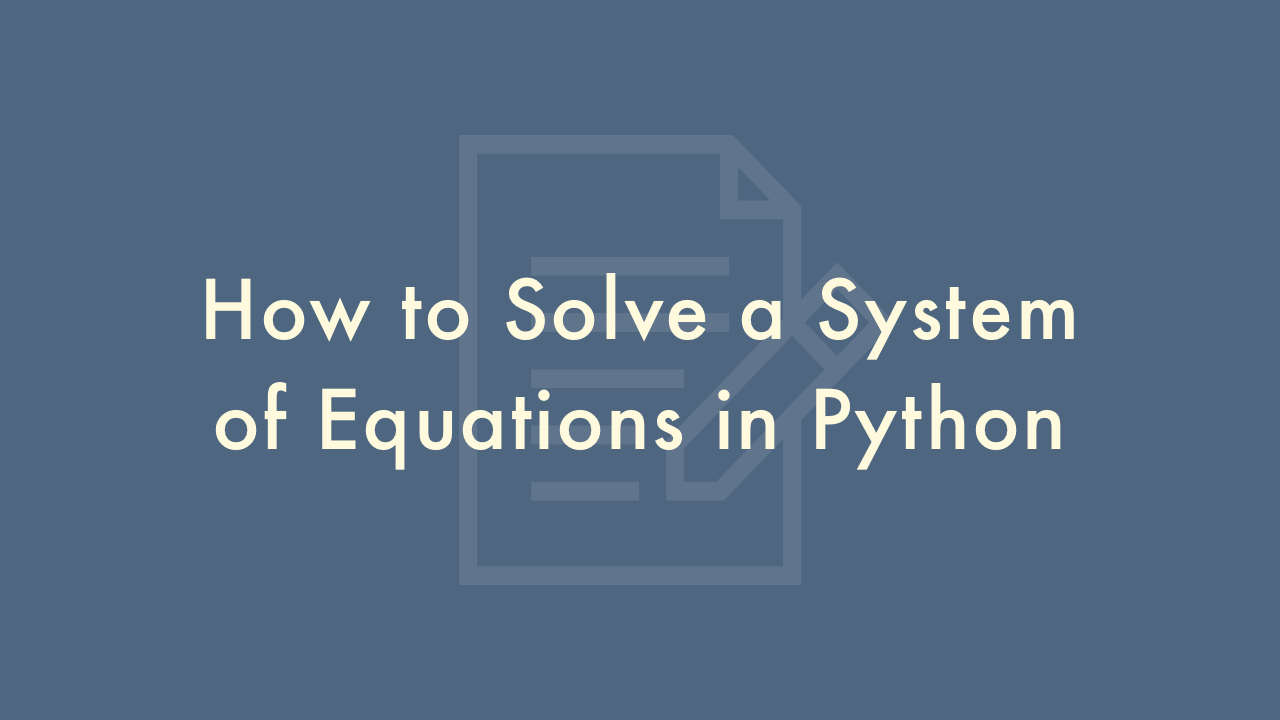
09/16/2021
Contents
In this article, you will learn how to solve a system of equations in Python.
How to solve a system of equations
To solve a system of equations in Python, you can use NumPy’s linalg.solve() function. Here are the steps:
Import the necessary libraries
You’ll need NumPy for the linalg.solve() function and any other necessary functions.
import numpy as np
Set up your system of equations
This could be any number of equations and variables, but for simplicity, let’s use a system of two equations with two variables:
3x + 2y = 5
2x - y = 2
We can represent this system of equations in matrix form as:
[3 2] [x] [5]
[2 -1] * [y] = [2]
So we have:
A = np.array([[3, 2], [2, -1]])
b = np.array([5, 2])
Solve the system of equations
Use linalg.solve() to solve the system of equations. This function takes in two arguments: the matrix A and the vector b. It returns the solution vector x:
x = np.linalg.solve(A, b)
Print the solution
print(x)
# Output:
# [1. 2.]
So the solution to the system of equations is x = 1 and y = 2.
Here’s the full code:
import numpy as np
# Set up the system of equations
A = np.array([[3, 2], [2, -1]])
b = np.array([5, 2])
# Solve the system of equations
x = np.linalg.solve(A, b)
# Print the solution
print(x)