How to Calculate Derivatives in Python
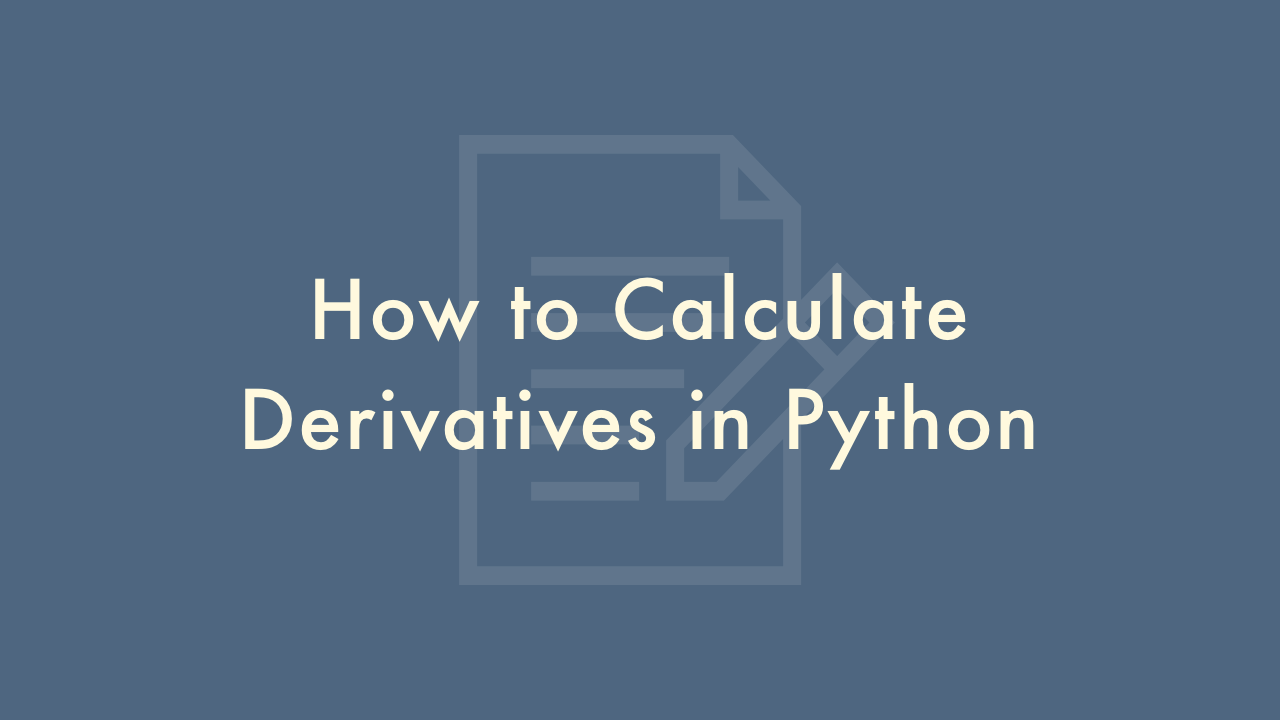
Contents
In this article, you will learn how to calculate derivatives in Python.
How to calculate derivatives
To calculate derivatives in Python, you can use the SymPy library. SymPy is a Python library for symbolic mathematics that can be used to perform various mathematical operations, including differentiation.
Here is an example of how to calculate the derivative of a function using SymPy:
from sympy import Symbol, diff
# Define the function
x = Symbol('x')
f = x**2 + 3*x + 1
# Calculate the derivative
dfdx = diff(f, x)
print(dfdx)
In this example, we first define the function f using the Symbol function from SymPy. We then use the diff function to calculate the derivative of f with respect to x. Finally, we print the result.
The output of the above code will be:
2*x + 3
This means that the derivative of f with respect to x is 2*x + 3.
Here are some additional details about calculating derivatives in Python using the SymPy library:
- SymPy supports both symbolic and numerical differentiation. Symbolic differentiation involves working with expressions in terms of variables, while numerical differentiation involves approximating derivatives using numerical methods. In this example, we used symbolic differentiation.
-
You can also calculate higher-order derivatives using SymPy. To calculate the second derivative of f with respect to x, for example, you can use the following code:
d2fdx2 = diff(f, x, 2) print(d2fdx2) # Output: # 2
This means that the second derivative of f with respect to x is a constant value of 2.