How to Use the Matplotlib.pyplot.title() Function in Python
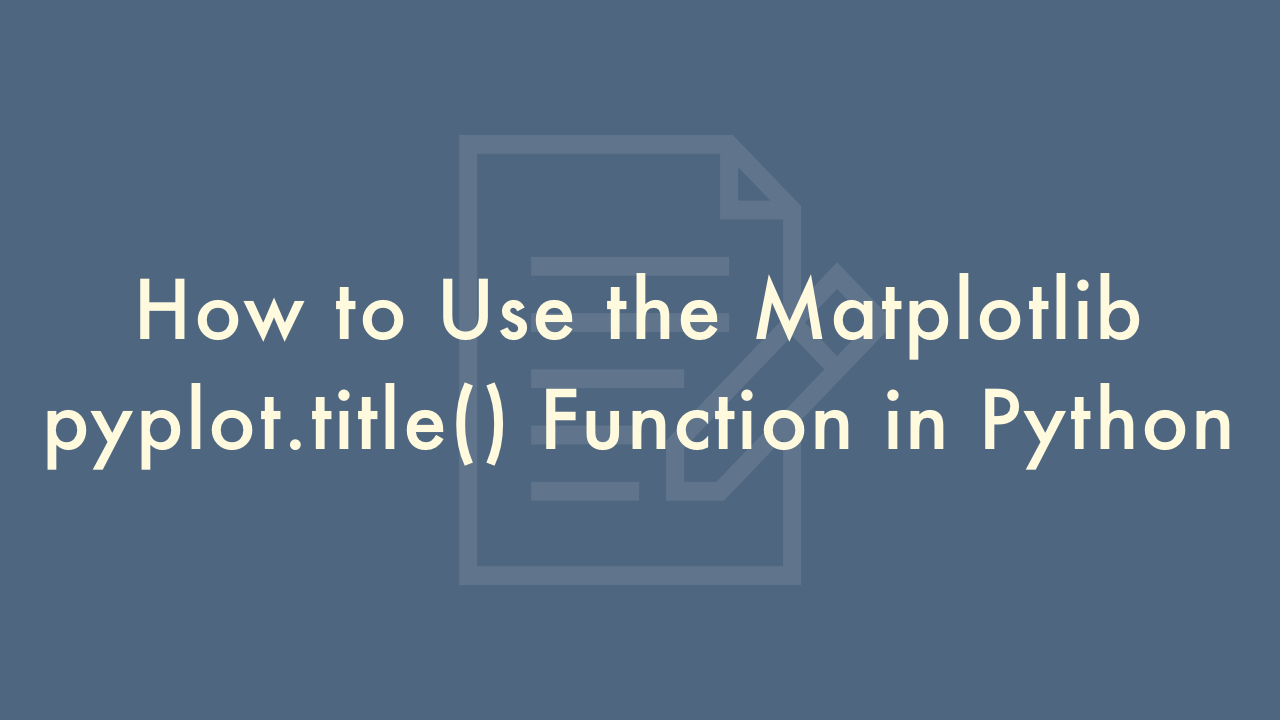
Contents
In this article, you will learn how to use the Matplotlib.pyplot.title() function in Python.
The Matplotlib.pyplot.title() Function
Matplotlib is a popular Python library for creating data visualizations. It provides a wide range of functions to create different types of plots such as bar, line, scatter, and many more. One of the functions provided by Matplotlib is title(), which is used to add a title to a plot.
The title() function in Matplotlib is used to add a title to a plot. The title is added to the top of the plot and provides a brief description of the data being visualized. The function takes a string as an argument, which is the text that will be displayed as the plot’s title.
Here is the syntax for using title() function:
import matplotlib.pyplot as plt
plt.title('Plot Title')
In this example, we have imported the pyplot module of the Matplotlib library and used the title() function to set the title of the plot to “Plot Title”.
The title() function can also take additional arguments to control the appearance of the title, such as the font size, font weight, and font color. Here is an example:
import matplotlib.pyplot as plt
plt.title('Plot Title', fontsize=16, fontweight='bold', color='blue')
In this example, we have set the font size to 16, the font weight to bold, and the font color to blue.
It is important to note that the title() function should be called after all other plot functions (such as plot(), scatter(), etc.) have been called, but before the plot is displayed with the show() function.
Here is an example of how to use the title() function to add a title to a plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.arange(0, 10, 0.1)
y = np.sin(x)
plt.plot(x, y)
plt.title('Sin(x) Function')
plt.xlabel('x')
plt.ylabel('y')
plt.show()
In this example, we have created a plot of the sine function using plot() function, then used title() function to set the title of the plot to “Sin(x) Function”. We also added labels to the x and y axes using the xlabel() and ylabel() functions.