How to Use the re.sub() Function in Python
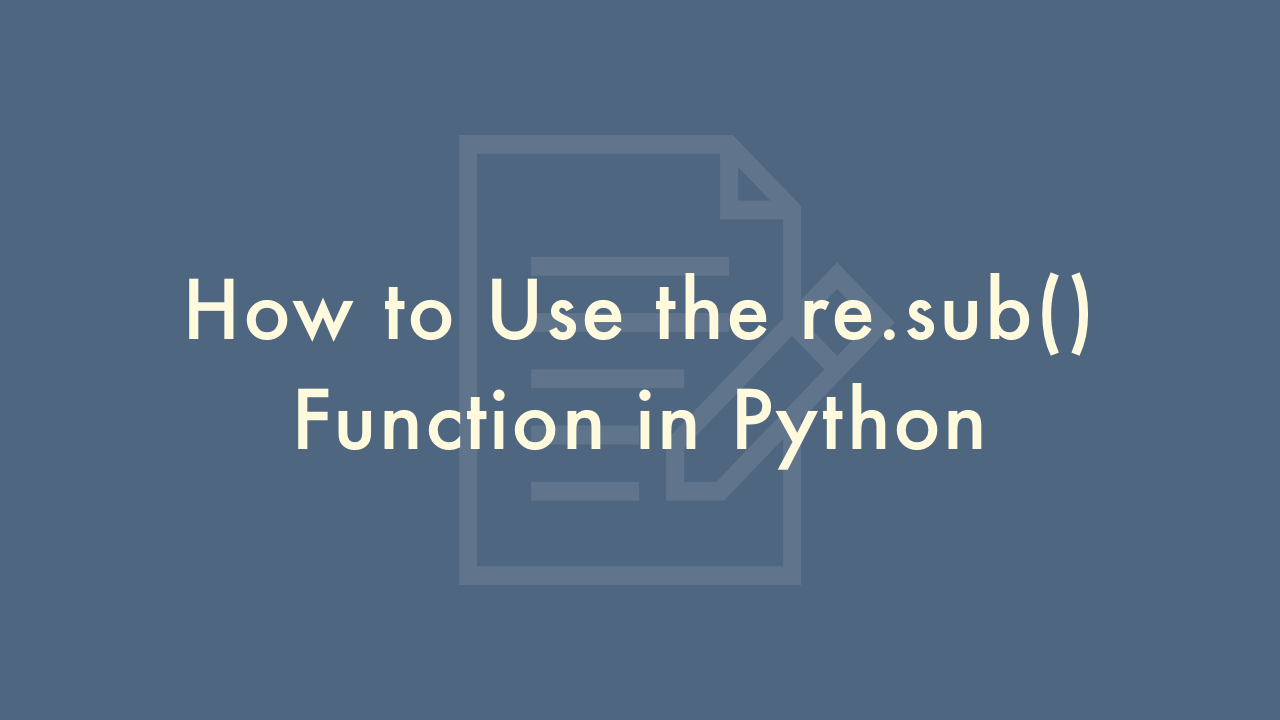
Contents
In this article, you will learn how to use the re.sub() function in Python.
Python re.sub() Function
The re.sub() function in Python is a powerful tool for string manipulation. It allows you to replace substrings in a string using regular expressions.
Here’s how to use the re.sub() function in Python:
Import the re module:
import re
Define the pattern you want to search for using regular expressions. For example, to search for all occurrences of the word “dog” in a string, you could use the following pattern:
pattern = r"dog"
Note that the r in front of the pattern string indicates that it is a raw string, which allows backslashes to be treated literally.
Define the replacement string. This is the string that will replace the matched substring. For example, if you want to replace “dog” with “cat”, you could use the following replacement string:
replacement = "cat"
Call the re.sub() function with the pattern, replacement string, and the string you want to search and replace on. For example:
original_string = "I have a dog and a cat"
new_string = re.sub(pattern, replacement, original_string)
print(new_string)
# Output:
# I have a cat and a cat
The re.sub() function will replace all occurrences of the pattern in the original string with the replacement string and return a new string. Note that you can also use the optional count parameter to specify the maximum number of replacements to make. For example, if you only want to replace the first occurrence of the pattern, you can use count=1.