How to Use For Loops in Python
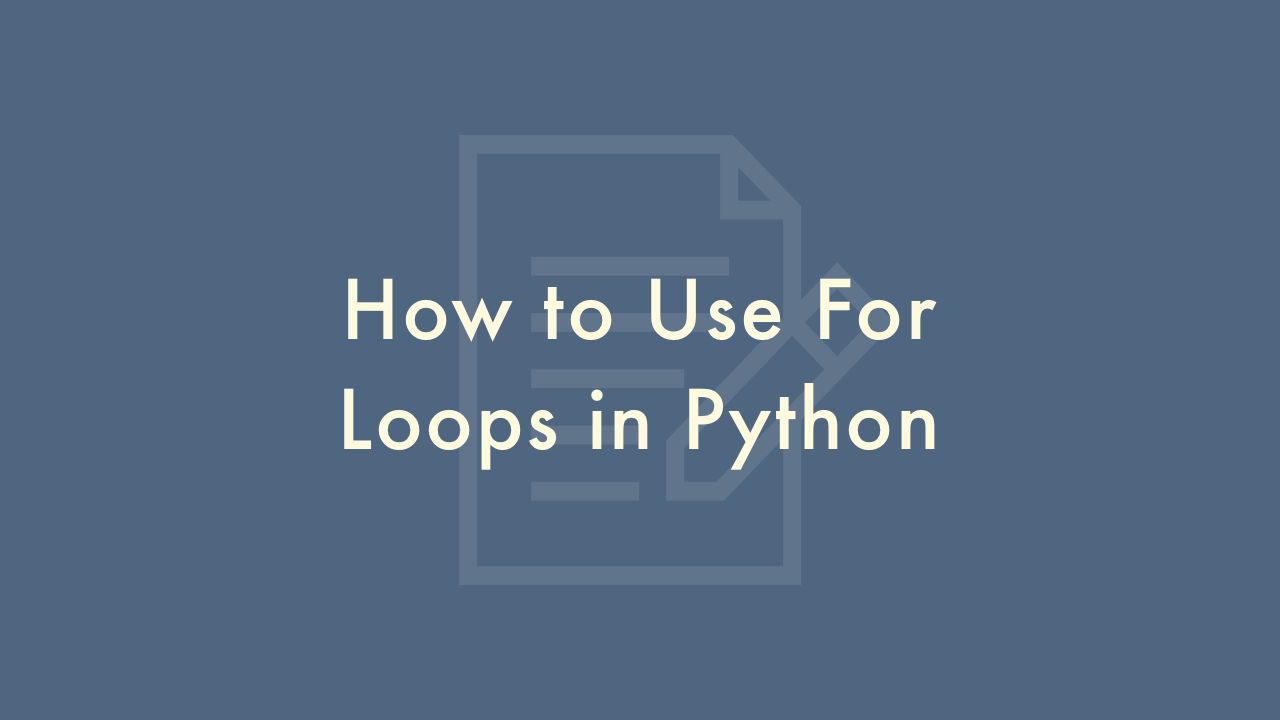
Contents
In this article, you will learn how to use for loops in Python.
Python For Loops
A for
loop in Python is used to iterate over a sequence (such as a list, tuple, dictionary, set, or string) and execute a block of code for each item in the sequence.
The syntax for a for loop is:
for variable in sequence:
# code to be executed
The variable in the for loop is a temporary placeholder for the current item in the sequence. On each iteration of the loop, the next item in the sequence is assigned to the loop variable.
For example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
The range() function is a built-in function in Python that generates a sequence of numbers. It can be used with the for loop to execute a block of code a specific number of times.
For example:
for i in range(5):
print(i)
Output:
0
1
2
3
4
To loop through a dictionary, you can use the items method, which returns a list of tuples, each containing a key-value pair.
For example:
person = {'name': 'John', 'age': 30, 'city': 'New York'}
for key, value in person.items():
print(f"{key}: {value}")
Output:
name: John
age: 30
city: New York
The enumerate() function can be used to loop over a sequence and keep track of the index of the current item in the sequence.
For example:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(f"{index}: {fruit}")
Output:
0: apple
1: banana
2: cherry
The zip() function can be used to iterate over multiple sequences in parallel.
For example:
fruits = ['apple', 'banana', 'cherry']
colors = ['red', 'yellow', 'red']
for fruit, color in zip(fruits, colors):
print(f"{fruit} is {color}")
Output:
apple is red
banana is yellow
cherry is red