How to Use the Python enumerate() Function
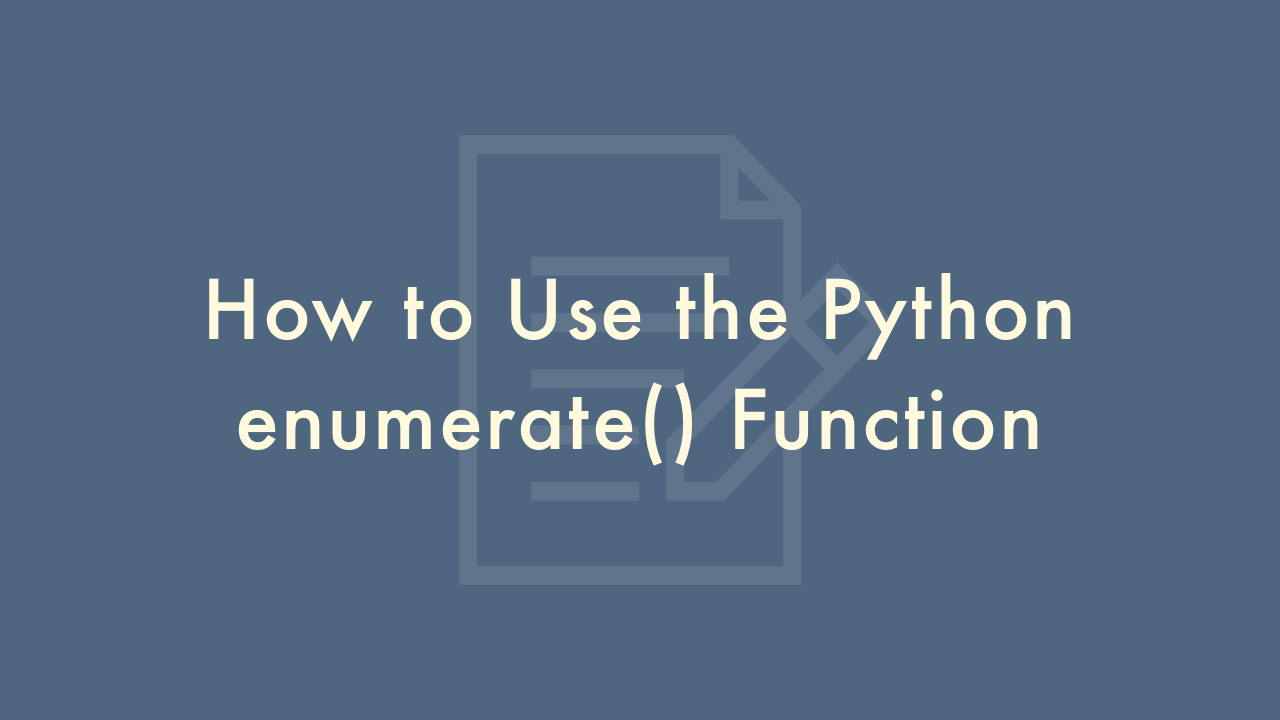
Contents
In this article, you will learn how to use the Python enumerate() function.
Python enumerate() Function
The enumerate() function in Python is used to add a counter to an iterable object, such as a list, tuple, or string. The function takes two arguments: an iterable object, and an optional starting value for the counter.
Syntax
Here’s the general syntax of the enumerate() function:
enumerate(iterable, start=0)
Parameters
iterable
: The object that you want to iterate over. It can be a list, tuple, string, or any other iterable object.start
: An optional argument that specifies the starting value for the counter. By default, the counter starts at 0, but you can specify a different starting value if you need to.
Example
Here’s an example that shows how to use enumerate() to loop over a list and print out each item along with its index:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(i, fruit)
//Output
//0 apple
//1 banana
//2 cherry
In this example, the enumerate() function is used to loop over the fruits list. The for loop is used to iterate over the result of enumerate(fruits), and i and fruit are used to capture the index and the item at each iteration.
You can also specify a different starting value for the counter by including the start argument in the call to enumerate():
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits, start=1):
print(i, fruit)
//Output
//1 apple
//2 banana
//3 cherry
In this example, the counter starts at 1 instead of 0.