How to Use the Numpy repeat() Function in Python
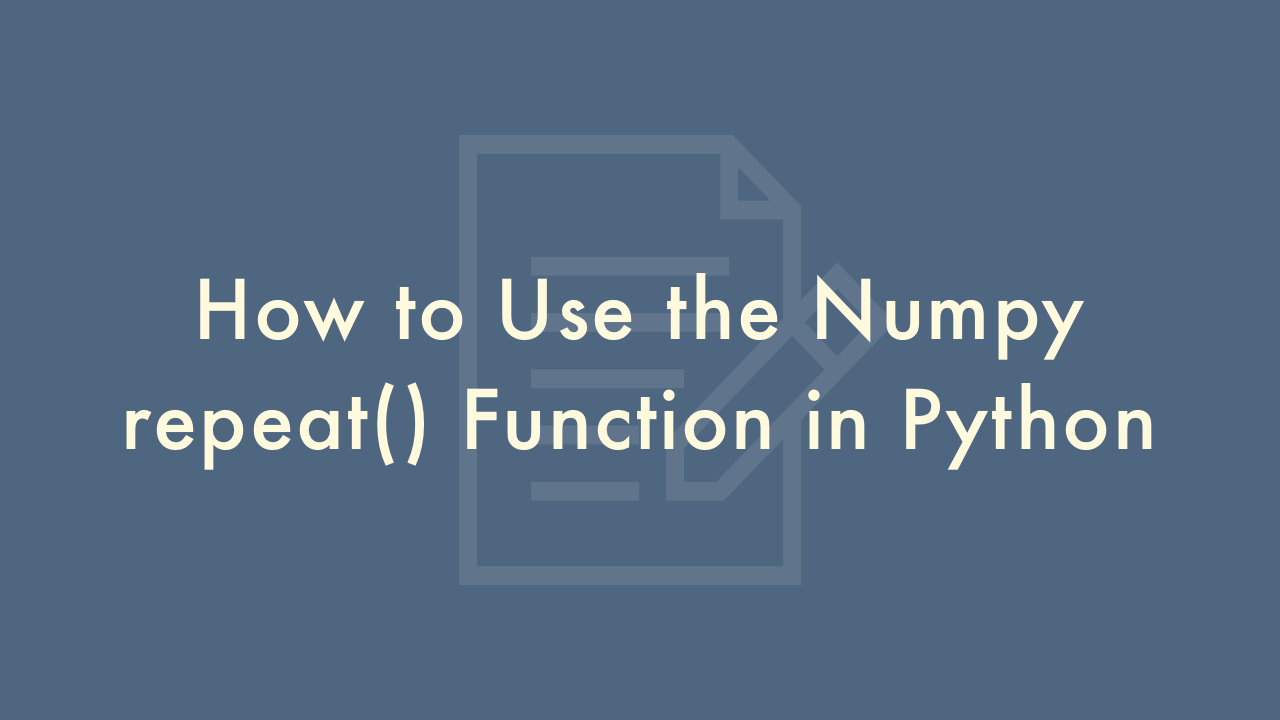
Contents
In this article, you will learn how to use the Numpy repeat() function in Python.
Numpy repeat() Function
The numpy.repeat() function is a powerful tool that allows you to repeat elements of an array along a specified axis. This function is useful when you want to replicate an element or a set of elements of an array a certain number of times, without having to use loops.
Here’s how you can use the numpy.repeat() function in Python:
First, you need to import the NumPy library:
import numpy as np
Next, create a NumPy array with the elements that you want to repeat:
arr = np.array([1, 2, 3])
To repeat each element of this array three times, you can use the repeat() function:
repeated_arr = np.repeat(arr, 3)
print(repeated_arr)
This will output the following:
array([1, 1, 1, 2, 2, 2, 3, 3, 3])
You can also specify the axis along which to repeat the elements. For example, to repeat each row of a 2D array three times, you can use the axis parameter:
arr_2d = np.array([[1, 2], [3, 4]])
repeated_arr_2d = np.repeat(arr_2d, 3, axis=0)
print(repeated_arr_2d)
This will output the following:
array([[1, 2],
[1, 2],
[1, 2],
[3, 4],
[3, 4],
[3, 4]])
In this case, the repeat() function repeats each row of the 2D array arr_2d three times along the first axis (axis=0). You can also use axis=1 to repeat each column of the 2D array three times.