How to Work with JSON Files in Python
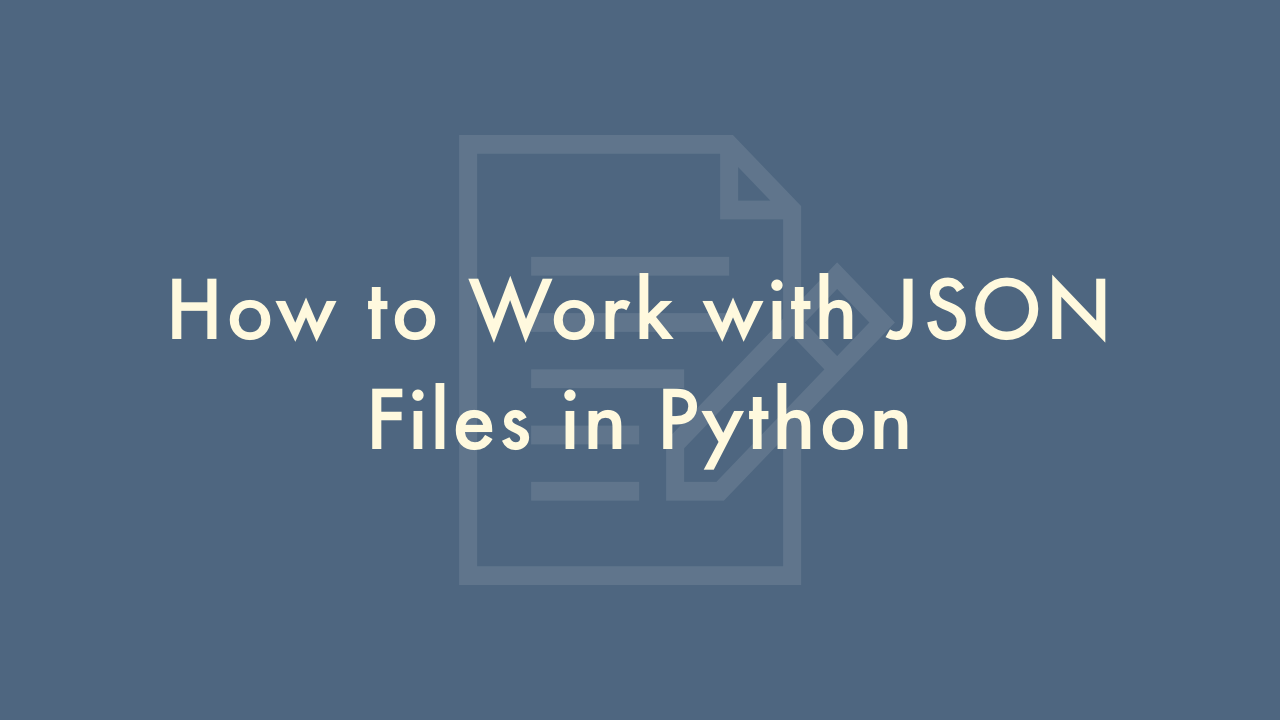
Contents
In this article, you will learn how to work with JSON files in Python.
Working with JSON Files
In Python, you can work with JSON files using the json module. The json module provides methods to read and write JSON data and provides an easy-to-use interface to interact with JSON-encoded data.
The json module provides several methods for working with JSON data in Python, including:
json.loads(json_string)
: This method deserializes a JSON-encoded string into a Python object. The argument json_string should be a string containing a valid JSON representation.json.dumps(python_object)
: This method serializes a Python object into a JSON-encoded string. The argument python_object can be any object that can be serialized into a JSON representation, such as a dictionary, list, or scalar value.json.load(file)
: This method reads a JSON-encoded file and returns a Python object. The argument file should be a file-like object (such as a file opened using open()) that is opened in read mode.json.dump(python_object, file)
: This method serializes a Python object into a JSON representation and writes it to a file. The argument python_object can be any object that can be serialized into a JSON representation, and the argument file should be a file-like object (such as a file opened using open()) that is opened in write mode.
When serializing data to a JSON representation, the json module will try to convert all Python objects into a JSON-encodable form. Some objects, such as complex numbers or custom objects, cannot be serialized into a JSON representation and will raise a TypeError.
Here’s a simple example that demonstrates how to read a JSON file in Python:
import json
# Open the file
with open('file.json') as json_file:
data = json.load(json_file)
# Access data in the JSON file
print(data)
In this example, we first open the file using the with statement, which ensures that the file will be closed automatically after we’re done working with it. We then use the json.load() method to read the contents of the file into a Python object (in this case, a dictionary).
To write a JSON file, you can use the json.dump() method:
import json
data = {
"name": "John Doe",
"age": 32,
"city": "New York"
}
# Open the file
with open('file.json', 'w') as json_file:
json.dump(data, json_file)
In this example, we first create a dictionary with some data, and then open the file in write mode (using the ‘w’ mode) and use the json.dump() method to write the data to the file.
By default, the json module uses a compact encoding for JSON data, but you can use the indent argument of the json.dump() method to specify the number of spaces to use for indentation, which can make the resulting JSON data more readable:
import json
data = {
"name": "John Doe",
"age": 32,
"city": "New York"
}
with open('file.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
In this example, the resulting JSON data will be indented using four spaces for each level of nesting.