How to Use the Numpy argmin() Function in Python
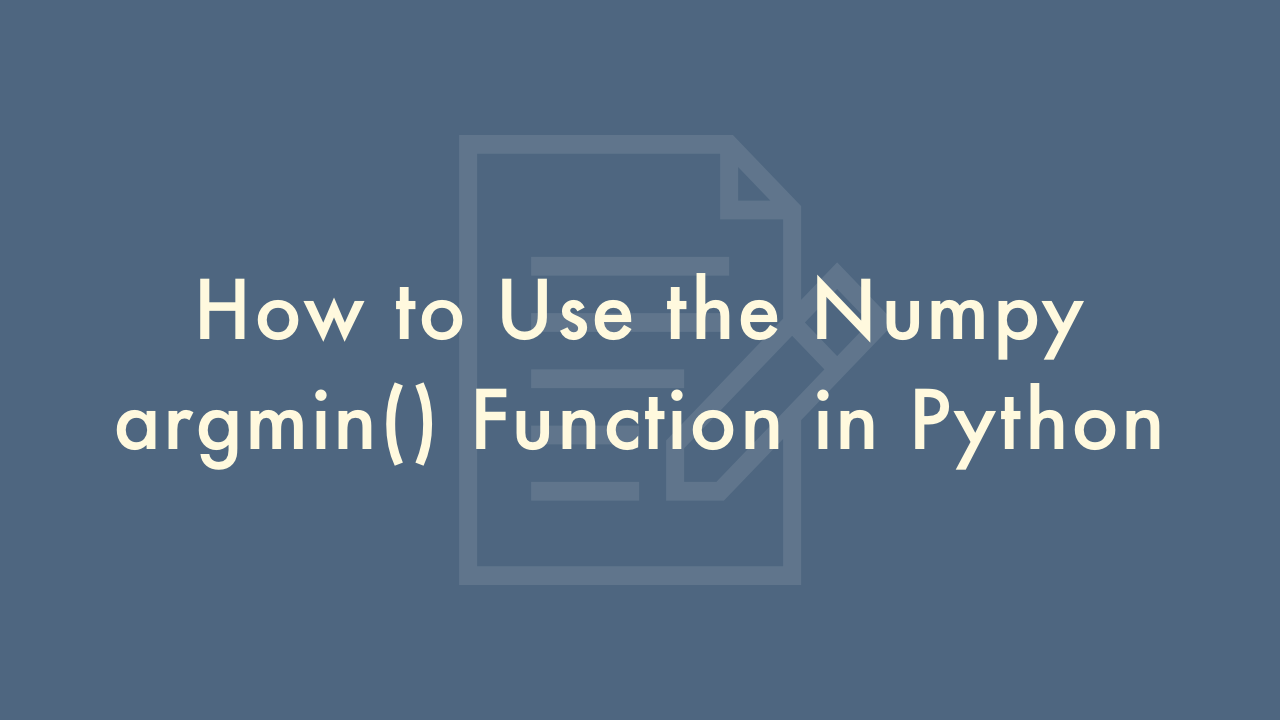
Contents
In this article, you will learn how to use the Numpy argmin() function in Python.
Numpy argmin() Function
The NumPy argmin() function is a built-in method in Python that returns the indices of the minimum value along a specified axis of an array.
Syntax
The syntax of the argmin() function is as follows:
numpy.argmin(arr, axis=None, out=None)
Parameters
arr
: The input array for which to find the index of the minimum value.axis
(optional): The axis along which to find the minimum value. If not specified, the function finds the index of the minimum value of the flattened array.out
(optional): An alternative output array in which to place the result. If not specified, the function returns a new array with the result.
Return Value
The argmin() function returns the index of the minimum value along the specified axis of the input array.
Example
import numpy as np
# Create a NumPy array
arr = np.array([10, 20, 30, 40, 50])
# Find the index of the minimum value in the array
min_index = np.argmin(arr)
# Print the index
print(min_index) # Output: 0
In the above code, we first import the NumPy module and create a NumPy array arr. Then, we use the argmin() function to find the index of the minimum value in the array. Finally, we print the index using the print() function.
You can also use the argmin() function to find the index of the minimum value along a specific axis of a multidimensional array. Here is an example:
import numpy as np
# Create a 2D NumPy array
arr = np.array([[10, 20, 30], [40, 50, 60]])
# Find the index of the minimum value along the rows (axis=1)
min_index = np.argmin(arr, axis=1)
# Print the index
print(min_index) # Output: [0 0]
In the above code, we create a 2D NumPy array arr. Then, we use the argmin() function with the axis=1 parameter to find the index of the minimum value along the rows of the array. Finally, we print the index using the print() function.