How to Check If a File Exists in Python
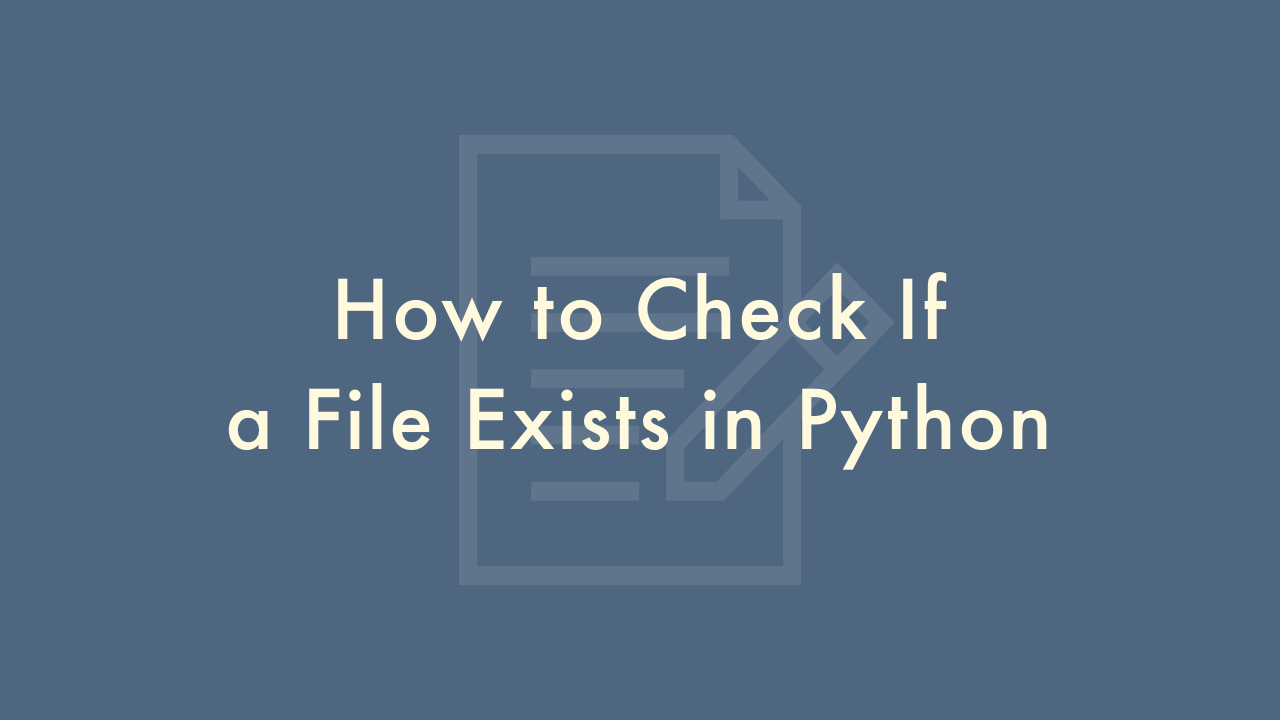
Contents
In this article, you will learn how to check if a file exists in Python.
Check If a File Exists in Python
In Python, you can use the os module to check if a file exists using the os.path.exists() function.
Here’s an example:
import os
filename = "example.txt"
if os.path.exists(filename):
print(f"{filename} exists.")
else:
print(f"{filename} does not exist.")
In this example, the function os.path.exists() returns True if the file exists, and False if it does not. You can use this information to determine whether to perform further actions on the file.
Here are a few more details on how you can use the os.path module to check for the existence of files in Python:
os.path.isfile(path)
: This function returns True if the specified path is an existing regular file (i.e. not a directory or a symbolic link). You can use it to check if a file exists and is a regular file.os.path.isdir(path)
: This function returns True if the specified path is an existing directory. You can use it to check if a path is a directory.os.path.lexists(path)
: This function returns True if the specified path is an existing file, including broken symbolic links. You can use it to check for the existence of any type of file.os.path.getsize(path)
: This function returns the size of the file specified by path, in bytes. If the file does not exist, it will raise a FileNotFoundError.
Here’s an example of how you can use these functions:
import os
filename = "example.txt"
if os.path.isfile(filename):
print(f"{filename} is a regular file.")
print(f"The size of {filename} is {os.path.getsize(filename)} bytes.")
elif os.path.isdir(filename):
print(f"{filename} is a directory.")
else:
print(f"{filename} does not exist or is a symbolic link.")
This example first checks if the specified filename is a regular file using os.path.isfile(), then it prints the size of the file using os.path.getsize(). If the file is not a regular file, it checks if it’s a directory using os.path.isdir(). If it’s neither a file nor a directory, it prints a message indicating that it does not exist or is a symbolic link.