How to Use Classes in Python
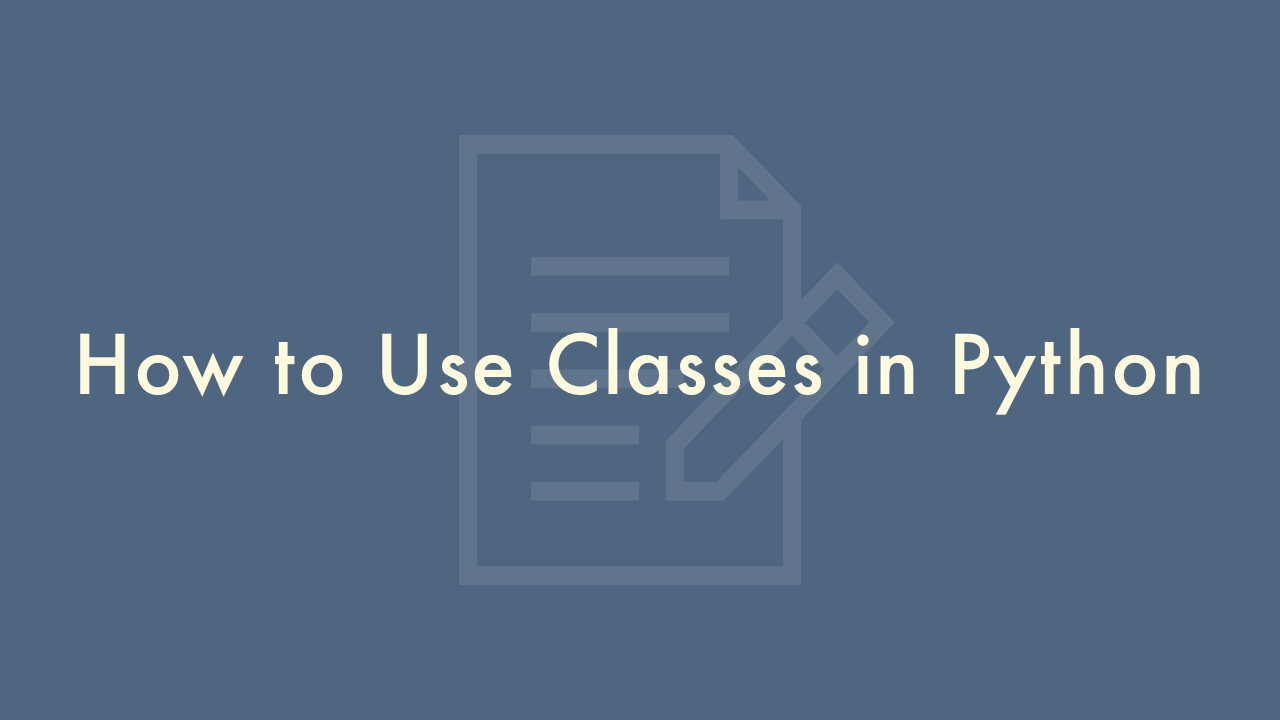
Contents
In this article, you will learn how to use classes in Python.
Using Classes in Python
Classes are a fundamental concept in object-oriented programming and are widely used in Python. They allow you to define custom data structures and encapsulate behavior that can be reused throughout your code.
Here is a basic example of how to create a class in Python:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def description(self):
return f'{self.year} {self.make} {self.model}'
In this example, the class Car has a constructor method __init__ that is called when you create a new instance of the class. The __init__ method takes three arguments: make, model, and year, which are used to initialize the attributes of the class.
The description method returns a string that describes the car. You can create an instance of the Car class by calling Car with the desired arguments:
my_car = Car("Tesla", "Model S", 2021)
print(my_car.description()) # Output: 2021 Tesla Model S
One of the key benefits of using classes in Python is that they allow you to encapsulate data and behavior into a single entity. This makes your code more organized and easier to maintain, as well as allowing you to write code that is more reusable.
In addition to the methods and variables that you define inside a class, Python provides several special methods that you can use to customize the behavior of your classes. For example, the __str__ method is called when you try to convert an instance of your class to a string, using the str() function or by using it in a print statement. Here’s an example:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def description(self):
return f'{self.year} {self.make} {self.model}'
def __str__(self):
return self.description()
my_car = Car("Tesla", "Model S", 2021)
print(my_car) # Output: 2021 Tesla Model S
You can also define methods like __eq__, __lt__, __add__, etc. to customize the behavior of the class when using comparison and mathematical operators.
Inheritance is another powerful feature of classes in Python. It allows you to create a new class based on an existing class, inheriting all its attributes and behaviors, and potentially adding or modifying them. This makes it easier to create new classes that are related to existing ones, and to reuse code across multiple classes. Here’s an example:
class ElectricCar(Car):
def __init__(self, make, model, year, battery_size):
super().__init__(make, model, year)
self.battery_size = battery_size
def description(self):
return f'{super().description()} with a {self.battery_size} kWh battery'
my_electric_car = ElectricCar("Tesla", "Model 3", 2022, 75)
print(my_electric_car.description()) # Output: 2022 Tesla Model 3 with a 75 kWh battery
In this example, the ElectricCar class inherits from the Car class, and adds a new attribute battery_size as well as a new method description that provides a more specific description of the car. The super() function allows you to call the method from the parent class, and is used to ensure that the description from the parent class is included in the description from the child class.