How to Remove a Specific Row in a CSV file with Python Pandas
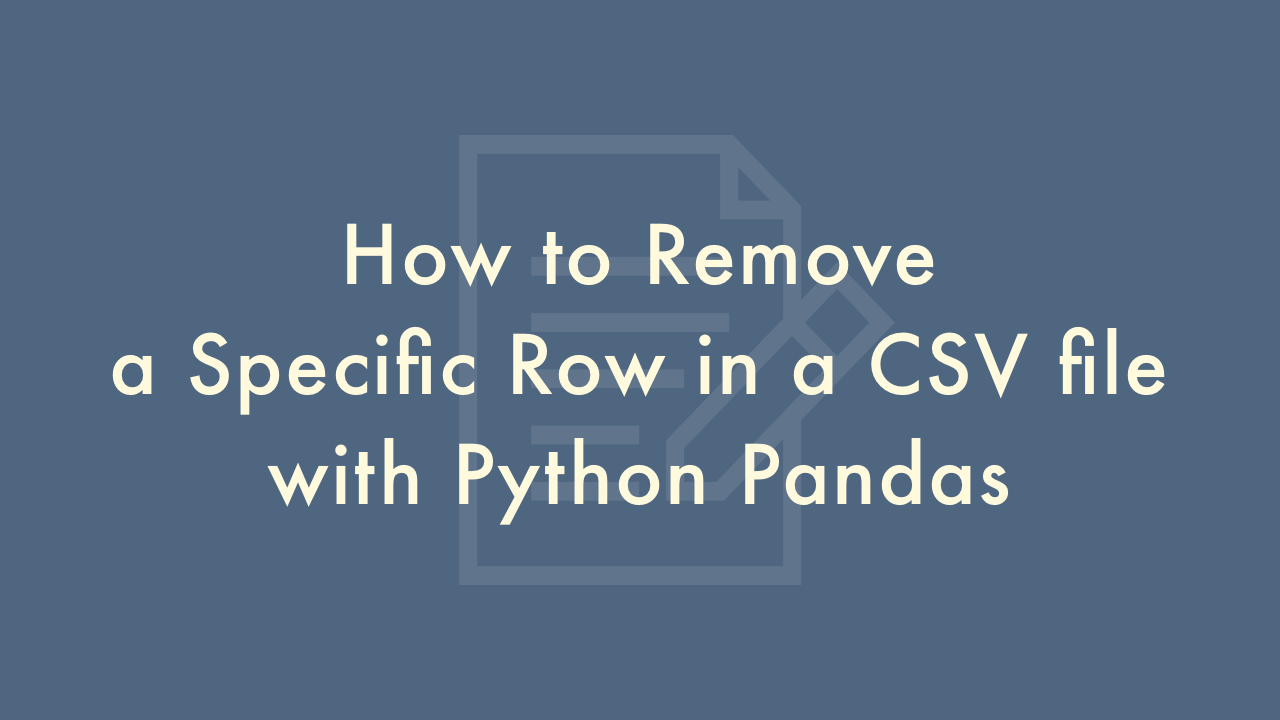
Contents
In this article, you will learn how to remove a specific row in a CSV file with Python Pandas.
Remove a specific row in a CSV file with Python Pandas
To remove a specific row from a CSV file using Python Pandas, you can follow these steps:
Import the pandas library:
The pandas library is used to handle data manipulation and analysis tasks. You need to import the library at the beginning of your Python script to access its functionality.
import pandas as pd
Read the CSV file into a Pandas DataFrame:
A DataFrame is a two-dimensional table-like data structure that can store data of different types in columns. You can create a DataFrame by reading a CSV file using the read_csv() method from pandas. This method reads the data from the CSV file and returns a DataFrame object.
df = pd.read_csv('filename.csv')
Here, filename.csv is the name of the CSV file you want to read.
Identify the specific row you want to remove:
You can identify the row you want to remove by using the index or a column value. In the example above, we used the index to identify the row. If you want to remove a row based on a column value, you can use a condition to select the row. For example, if you want to remove the row where the ‘Name’ column has the value ‘John’, you can use the following code:
df = df[df['Name'] != 'John']
This code selects all rows where the ‘Name’ column is not equal to ‘John’ and updates the DataFrame with the selected rows.
Use the drop() method to remove the row from the DataFrame:
The drop() method is used to remove rows or columns from a DataFrame. You can specify the row or column you want to remove using its index or label. To remove a specific row, you need to specify its index using the drop() method.
df = df.drop(row_to_remove)
Here, row_to_remove is the index of the row you want to remove.
Save the updated DataFrame to a new CSV file:
Once you have removed the specific row, you can save the updated DataFrame to a new CSV file using the to_csv() method from pandas. You need to specify the name of the new CSV file and set the index parameter to False to exclude the index column from the new CSV file.
df.to_csv('new_filename.csv', index=False)
Here, new_filename.csv is the name of the new CSV file you want to create.
These are the basic steps you need to follow to remove a specific row from a CSV file using Python Pandas.