How to Use Python Pillow
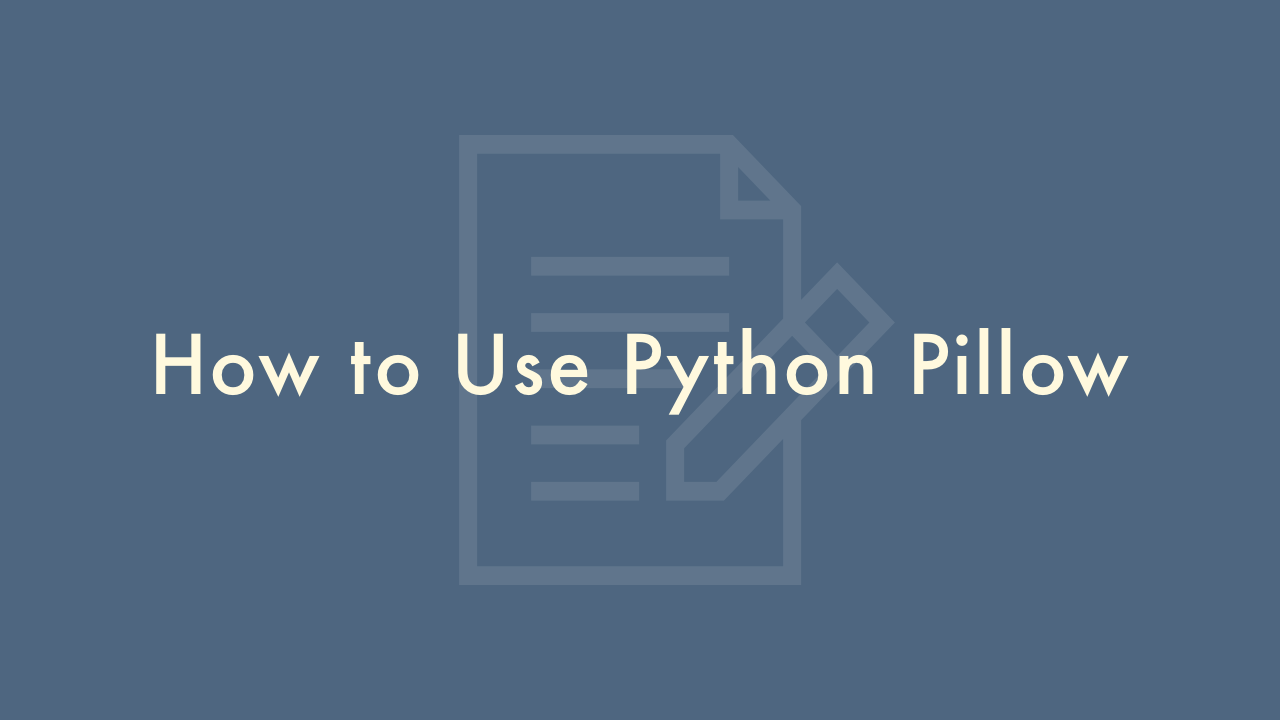
Contents
In this article, you will learn how to use Python Pillow.
How to Use Python Pillow
The Python Imaging Library (PIL) is now known as pillow and it is a library for handling and transforming image files. Here are some basic steps to get started with using pillow:
Install pillow:
To install the pillow library, you can use the pip package manager by running ‘pip install pillow’ in your command line or terminal.
pip install pillow
Importing the library:
Once you have installed the library, you can import it into your Python script by using the following code:
from PIL import Image
Opening an Image:
You can open an image by using the Image.open() method, like this:
from PIL import Image
img = Image.open("image.jpg")
Displaying an Image:
You can display an image using the img.show() method, which opens the image in your default image viewer.
Resizing an Image:
You can resize an image by using the img.resize() method, which takes a tuple of the new image size as an argument:
img_resized = img.resize((300, 200))
img_resized.show()
Rotating an Image:
You can rotate an image by using the img.rotate() method, which takes the angle of rotation in degrees as an argument:
img_rotated = img.rotate(45)
img_rotated.show()
Cropping an Image:
You can crop an image by using the img.crop() method, which takes a tuple of the left, upper, right, and lower pixel coordinates as an argument:
img_cropped = img.crop((0, 0, 150, 100))
img_cropped.show()
Saving an Image:
Finally, you can save the modified image by using the img.save() method, which takes the file name as an argument:
img_resized.save("resized_image.jpg")
Here are some more advanced operations you can perform using pillow:
Manipulating Image Pixels:
You can access and modify the pixels of an image using the img.load() method, which loads the image data into memory. You can then use the img.getpixel() and img.putpixel() methods to retrieve and set the color of individual pixels, respectively.
Image Filters:
pillow provides a variety of image filters that you can apply to your images, such as sharpen, blur, and contour. You can apply these filters by using the img.filter() method, which takes a filter object as an argument.
Image Transformation:
You can perform various transformations on an image, such as scaling, rotating, and flipping. For example, you can flip an image vertically using the img.transpose(method=Image.Transpose.FLIP_TOP_BOTTOM) method.
Image Channels:
You can manipulate the channels of an image, such as splitting and merging channels. For example, you can split an image into its red, green, and blue channels using the img.split() method.
Image Formats:
pillow supports a wide range of image formats, including BMP, JPEG, PNG, and TIFF. You can save an image in a specific format by passing the format string as an argument to the img.save() method, like this: img.save(“image.png”, format=”PNG”).
These are just a few examples of what you can do with pillow. It is a very powerful library for image manipulation, and there are many more methods and capabilities available. I would encourage you to explore the official pillow documentation for more information.