How to Use the Twitter API in Python
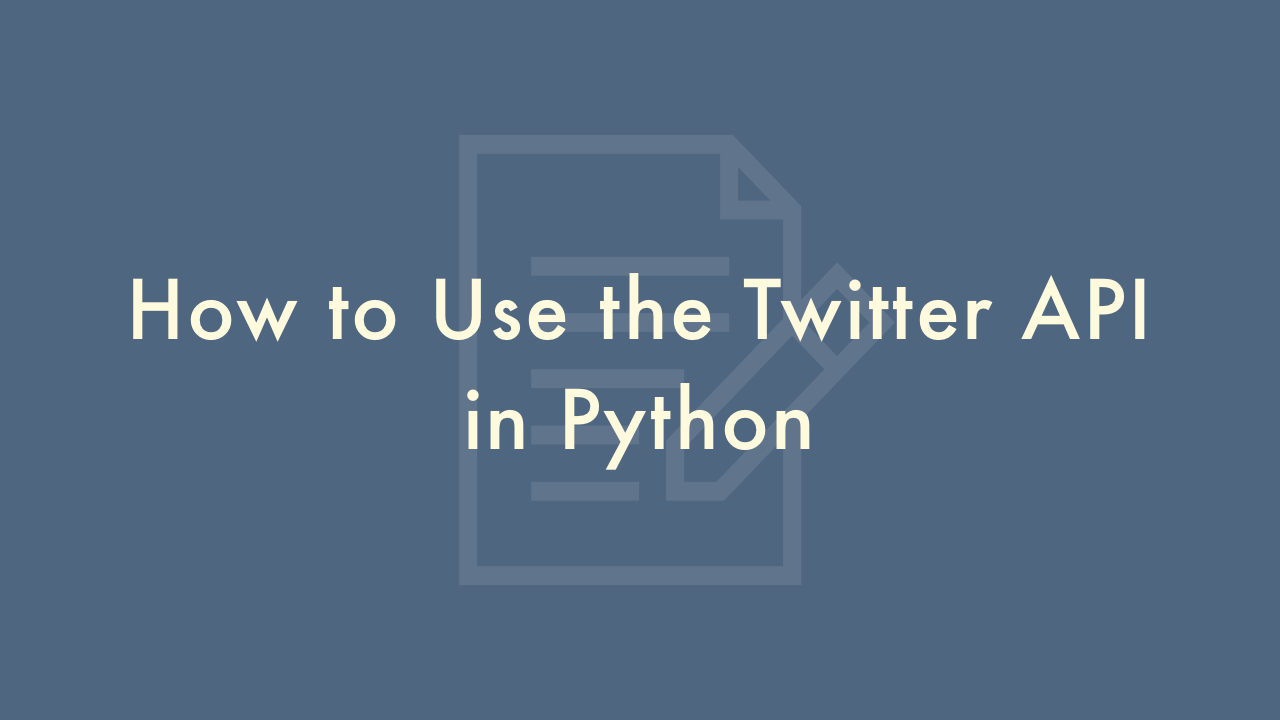
Contents
In this article, you will learn how to use the Twitter API in Python.
How to Use the Twitter API
To use the Twitter API in Python, you’ll need to follow these steps:
Create a Twitter Developer Account
Before you can use the Twitter API, you need to create a developer account. Go to the Twitter Developer Dashboard and sign in with your Twitter account. Follow the prompts to create a developer account.
Create a Twitter App
Once you have a developer account, you need to create a Twitter app. Click the “Create an App” button, give your app a name, and follow the prompts to create it.
Get your API keys
Once you have created your app, you need to generate your API keys. You will need the Consumer Key, Consumer Secret, Access Token, and Access Token Secret to connect to the Twitter API. You can find these keys under the “Keys and Tokens” tab of your app dashboard.
Install Tweepy
Tweepy is a Python package that provides a convenient way to use the Twitter API. You can install it using pip:
pip install tweepy
Connect to the API
Use Tweepy to connect to the Twitter API using your API keys. Here is an example of how to do it:
import tweepy
consumer_key = "your_consumer_key"
consumer_secret = "your_consumer_secret"
access_token = "your_access_token"
access_token_secret = "your_access_token_secret"
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
Use the API
Now that you’re connected to the API, you can use it to search for tweets, post tweets, and more. Here’s an example of how to search for tweets:
tweets = api.search(q="Python", count=10)
for tweet in tweets:
print(tweet.text)
This will search for tweets containing the word “Python” and print the text of the first 10 results.
You can now use the Twitter API in Python to do all sorts of cool things. Just be sure to follow Twitter’s API usage guidelines to avoid getting your account suspended.