How to Use Python Quandl Package
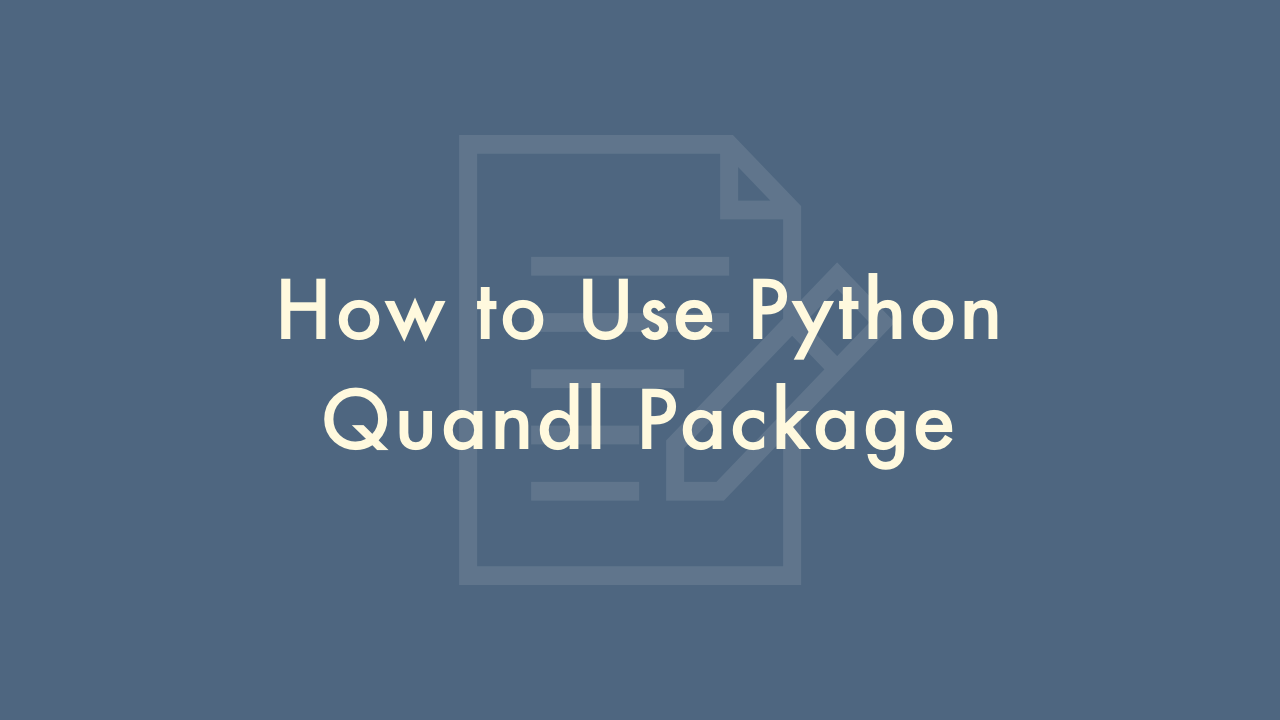
Contents
In this article, you will learn how to Use Python Quandl package.
Python Quandl Package
Quandl is a Python package that provides access to millions of financial and economic datasets. Here are the steps to use Quandl in Python:
Install the Quandl package
You can use pip to install the Quandl package in Python. Open your terminal or command prompt and run the following command:
pip install quandl
Import the Quandl package
After installing the Quandl package, import it in your Python script or notebook by running the following command:
import quandl
Get an API key
To access Quandl data, you need to sign up for a free API key. You can get an API key by creating a free account on the Quandl website.
Set the API key
After getting your API key, set it in your Python script or notebook by running the following command:
quandl.ApiConfig.api_key = "YOUR_API_KEY"
Access Quandl data
You can access Quandl data using the quandl.get() function. This function takes the dataset code as the parameter and returns a Pandas DataFrame object.
data = quandl.get("WIKI/AAPL")
print(data.head())
In the above example, we are retrieving the Apple stock price data from the WIKI dataset.
Plot the data
You can use various Python plotting libraries such as Matplotlib, Seaborn, or Plotly to visualize the data.
Here is a complete example of how to use Quandl in Python:
import quandl
import matplotlib.pyplot as plt
quandl.ApiConfig.api_key = "YOUR_API_KEY"
data = quandl.get("WIKI/AAPL")
plt.plot(data.index, data['Adj. Close'])
plt.title('Apple Stock Price')
plt.xlabel('Date')
plt.ylabel('Price ($)')
plt.show()
In the above example, we are plotting the Apple stock price data retrieved from the WIKI dataset using Matplotlib.