How to Use the Pickle Module in Python
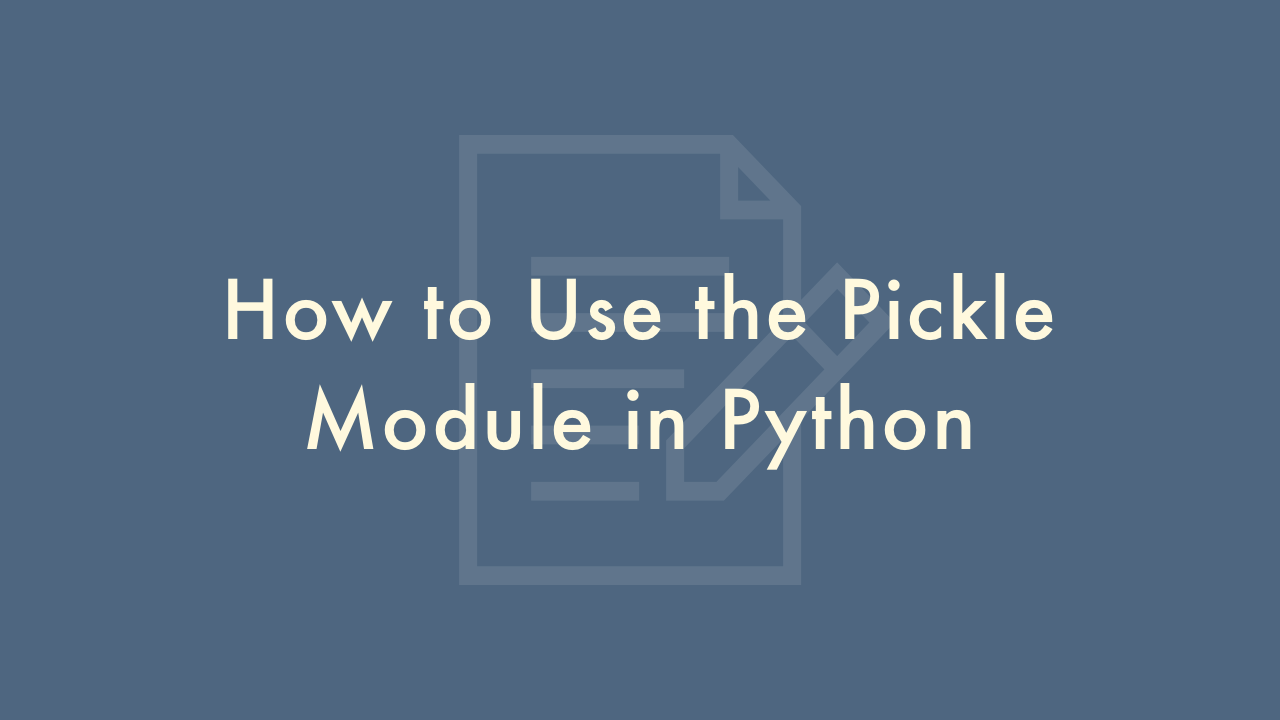
Contents
In this article, you will learn how to use the pickle module in Python.
The pickle module
The pickle module in Python allows you to serialize and deserialize Python objects to a byte stream. This means that you can save a Python object to disk and later load it back into memory, preserving its state.
Here’s how you can use the pickle module to save and load objects:
import pickle
# Save an object to disk
with open("object.pickle", "wb") as f:
pickle.dump(my_object, f)
# Load an object from disk
with open("object.pickle", "rb") as f:
my_loaded_object = pickle.load(f)
The dump function is used to write the object to disk, while the load function is used to read the object back into memory. You should always use the with statement when working with files in Python to ensure that the file is properly closed, even if an exception is raised.
Here’s some more information on using the pickle module in Python:
Protocols
The pickle module supports different protocols for serializing and deserializing objects. The default protocol (protocol 0) is a human-readable format, while the other protocols (protocol 1 to 5) are optimized for speed and size. You can specify the protocol to use when calling the dump and load functions by passing a protocol argument.
For example:
import pickle
# Save an object using protocol 2
with open("object.pickle", "wb") as f:
pickle.dump(my_object, f, protocol=2)
# Load an object using the highest protocol available
with open("object.pickle", "rb") as f:
my_loaded_object = pickle.load(f)
Compatibility
The pickle module is not guaranteed to be compatible across different Python versions. If you need to ensure that your pickled objects can be read by other systems, you should use a specific protocol (such as protocol 3) and ensure that the other systems are using the same version of Python.
Custom objects
By default, the pickle module can only serialize and deserialize objects that are built-in types or that are defined in modules that are part of the Python standard library. If you want to serialize and deserialize custom objects, you need to implement the __getstate__ and __setstate__ methods in your class. The __getstate__ method should return a representation of the object’s state, and the __setstate__ method should take that representation and restore the object’s state.
Here’s an example of how you can use these methods to serialize and deserialize a custom object:
import pickle
class MyClass:
def __init__(self, value):
self.value = value
def __getstate__(self):
return self.value
def __setstate__(self, value):
self.value = value
# Save an object of custom class
with open("object.pickle", "wb") as f:
pickle.dump(MyClass(42), f)
# Load an object of custom class
with open("object.pickle", "rb") as f:
my_loaded_object = pickle.load(f)