How to Use the Numpy linspace() Function in Python
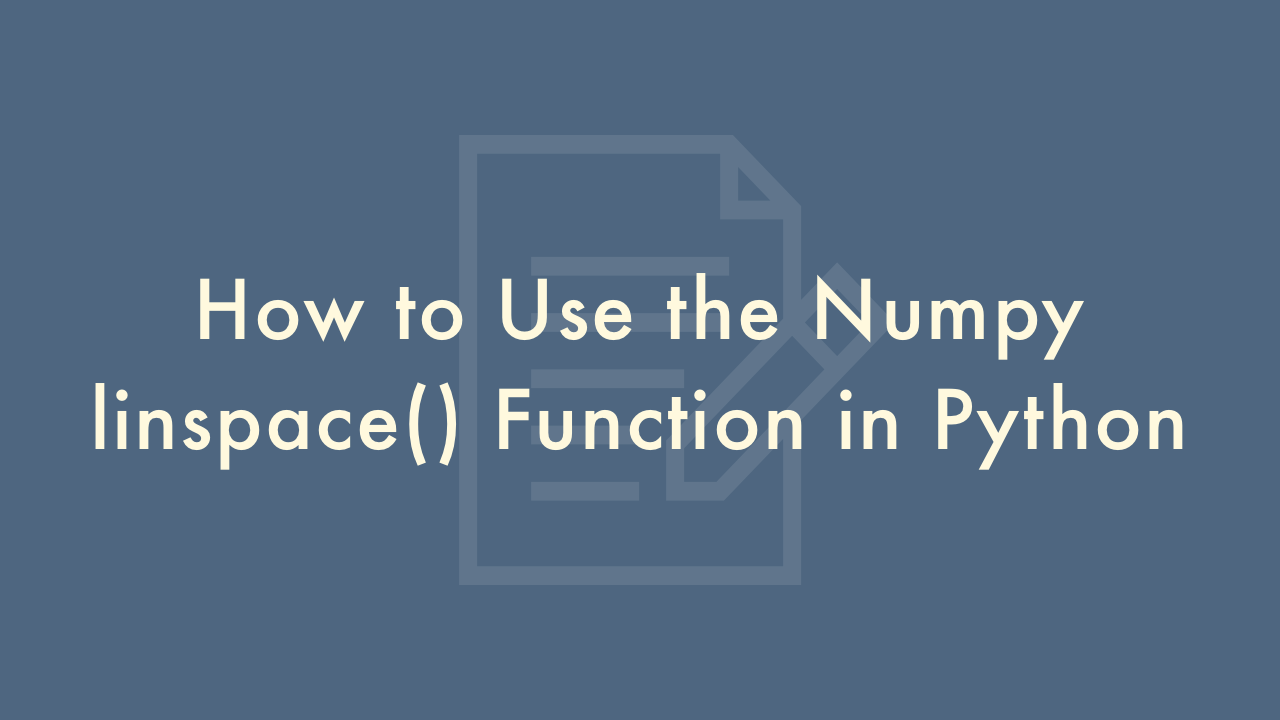
Contents
In this article, you will learn how to use the Numpy linspace() function in Python.
Numpy linspace() Function
The linspace() function is a part of the numpy library in Python that allows you to create a sequence of equally spaced numbers over a specified interval. The function takes three arguments: the start value of the sequence, the end value of the sequence, and the number of elements in the sequence. Here’s how you can use the linspace() function in Python:
import numpy as np
# Creating an array of 10 equally spaced numbers between 0 and 1 (both inclusive)
arr = np.linspace(0, 1, 10)
print(arr)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556
0.66666667 0.77777778 0.88888889 1. ]
In this example, we imported the numpy library and used the linspace() function to create an array of 10 equally spaced numbers between 0 and 1 (both inclusive). The resulting array contains the values [0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ].
You can use the linspace() function to create arrays of any length with any start and end values. The linspace() function can be useful for generating input values for mathematical functions, plotting graphs, and many other applications.
Here are a few more things to keep in mind when using the numpy linspace() function:
- The start and end values of the sequence are included in the output array by default. In the example above, we created a sequence of 10 equally spaced numbers between 0 and 1 (both inclusive), so the resulting array contained 10 elements, including 0 and 1.
- The number of elements in the output sequence is determined by the third argument to the linspace() function. In the example above, we specified 10 as the number of elements, so the resulting array contained 10 equally spaced values.
-
You can use the dtype argument to specify the data type of the output array. By default, the linspace() function creates an array of floating-point numbers. For example, if you want to create an array of integers, you can specify the data type as int:
# Creating an array of 5 equally spaced integers between 1 and 10 (both inclusive) arr = np.linspace(1, 10, 5, dtype=int) print(arr) # Output: # [ 1 3 5 7 10]
In this example, we created an array of 5 equally spaced integers between 1 and 10 (both inclusive) by specifying the dtype argument as int. The resulting array contains the values [1 3 5 7 10].
-
You can use the endpoint argument to specify whether the end value of the sequence should be included in the output array. By default, endpoint is set to True, so the end value is included in the output array. If you set endpoint to False, the end value is not included:
# Creating an array of 5 equally spaced numbers between 1 and 10 (excluding 10) arr = np.linspace(1, 10, 5, endpoint=False) print(arr) # Output: # [1. 3.8 6.6 9.4]
In this example, we created an array of 5 equally spaced numbers between 1 and 10 (excluding 10) by setting endpoint to False. The resulting array contains the values [1. 3.8 6.6 9.4].