How to Find Items in a Python List
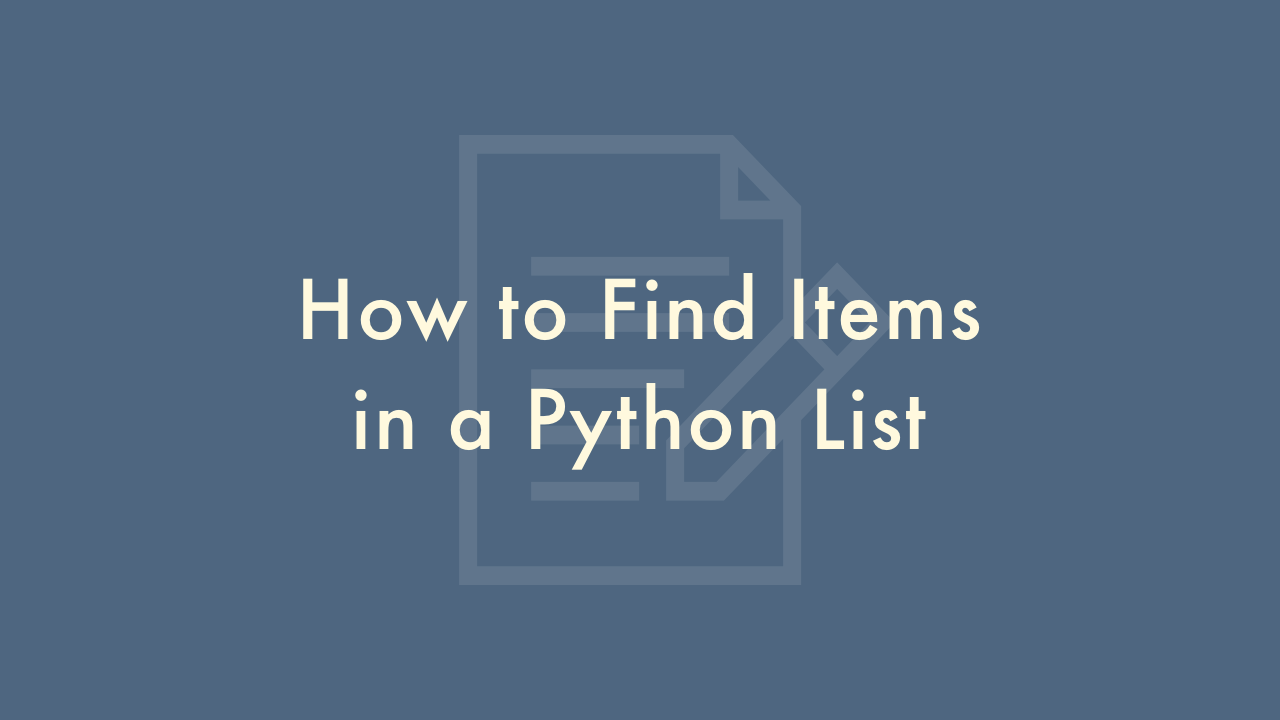
Contents
In this article, you will learn how to find items in a Python list.
How to find items
Using the in operator
You can check whether an item exists in a list using the in operator. For example:
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("3 is in the list")
Using the index() method
The index() method returns the index of the first occurrence of an item in a list. For example:
my_list = ["apple", "banana", "orange", "grape"]
index = my_list.index("orange")
print(index) # Output: 2
Note that if the item is not in the list, the index() method will raise a ValueError exception.
Using a loop
You can loop through the list and check each item until you find the one you’re looking for. For example:
my_list = ["dog", "cat", "rabbit", "hamster"]
for item in my_list:
if item == "cat":
print("Found cat!")
break
This will print “Found cat!” and exit the loop as soon as it finds “cat” in the list.
Using a list comprehension
You can also use a list comprehension to filter a list and return only the items that match a certain criteria. For example:
my_list = [1, 2, 3, 4, 5]
filtered_list = [x for x in my_list if x > 3]
print(filtered_list) # Output: [4, 5]
This will create a new list containing only the items from my_list that are greater than 3.
Using the count() method
The count() method returns the number of occurrences of an item in a list. For example:
my_list = ["red", "green", "blue", "green", "red"]
count = my_list.count("green")
print(count) # Output: 2
Using the any() function
The any() function returns True if any item in an iterable (including a list) is True. You can use this to check if a list contains any items that meet a certain criteria. For example:
my_list = [0, False, "", None, 5]
if any(my_list):
print("The list contains at least one non-falsey value")
This will print the message because 5 is a non-falsey value.
Using the filter() function
The filter() function returns an iterator that contains only the items from an iterable (including a list) that meet a certain criteria. For example:
my_list = [1, 2, 3, 4, 5]
filtered = filter(lambda x: x > 3, my_list)
print(list(filtered)) # Output: [4, 5]
This will create a new list containing only the items from my_list that are greater than 3.
Using the enumerate() function
The enumerate() function returns an iterator that produces pairs of the form (index, item) for each item in an iterable (including a list). You can use this to loop over a list and keep track of the index of each item. For example:
my_list = ["apple", "banana", "orange", "grape"]
for i, item in enumerate(my_list):
if item == "orange":
print(f"Found 'orange' at index {i}")
break
This will print “Found ‘orange’ at index 2”.