How to Get All Combinations of a List in Python
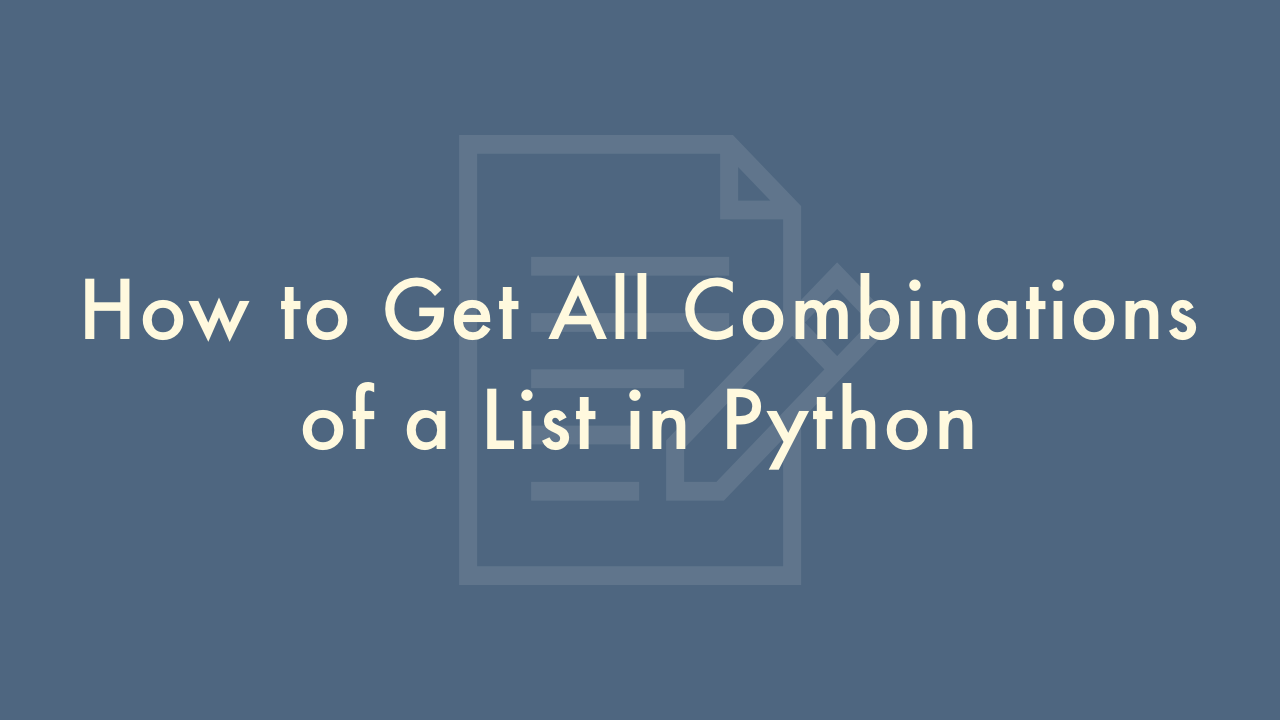
Contents
In this article, you will learn how to get all combinations of a list in Python.
Get All Combinations of a List
There are several ways to get all combinations of a list in Python. Here are two common methods:
Using the itertools module
The itertools
module in Python provides a function called combinations
that takes two arguments: an iterable objects and an integer n, and returns an iterator over n-length tuples of elements from the input iterable. To get all combinations, you can use a for loop and generate all combinations for each length starting from 1 to the length of the list.
import itertools
def all_combinations(lst):
result = []
for i in range(1, len(lst)+1):
combinations = list(itertools.combinations(lst, i))
result.extend(combinations)
return result
Using Recursion
Another way to get all combinations of a list is to use recursion. The basic idea is to generate all combinations for a sublist, and then add each element in the list to the sublist one by one.
def all_combinations(lst):
if len(lst) == 0:
return [[]]
result = []
for i in range(len(lst)):
m = lst[i]
rem_list = lst[:i] + lst[i+1:]
for p in all_combinations(rem_list):
result.append([m] + p)
return result
Both of these methods return a list of tuples, where each tuple represents a combination of elements from the original list.