How to Use the Python strftime() Function
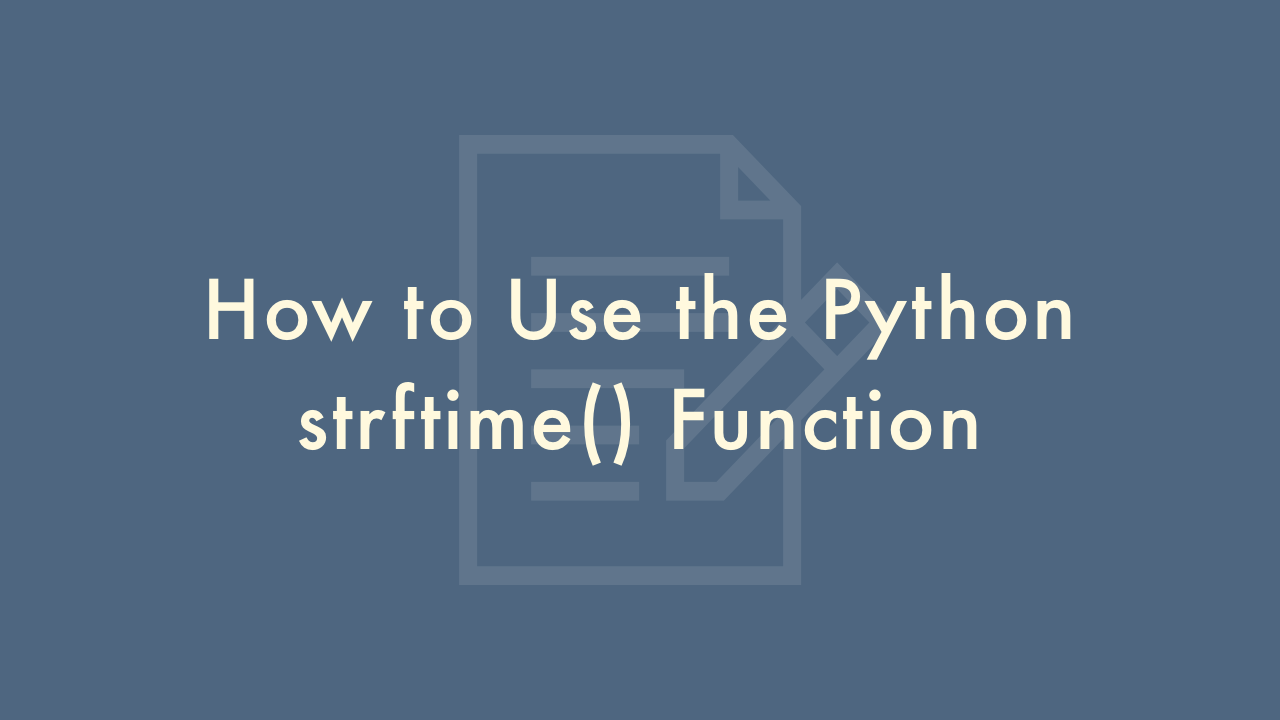
Contents
In this article, you will learn how to use the Python strftime() function.
Python strftime() Function
The Python strftime() function is used to format date and time values as strings. It takes a date or time object and returns a string representing that object in a specific format. Here’s how you can use strftime() in Python:
Import the datetime module:
import datetime
Create a datetime object:
date_obj = datetime.datetime(2023, 2, 16, 10, 30, 15)
This creates a datetime object representing the date and time February 16th, 2023 at 10:30:15.
Call strftime() on the datetime object and pass a format string as an argument:
formatted_date = date_obj.strftime("%Y-%m-%d %H:%M:%S")
The format string specifies the desired format of the string. In this case, %Y represents the year, %m represents the month, %d represents the day, %H represents the hour, %M represents the minute, and %S represents the second. The resulting string will be “2023-02-16 10:30:15”.
Here are some other common format codes you can use with strftime():
- %a: abbreviated weekday name (e.g. “Mon”)
- %A: full weekday name (e.g. “Monday”)
- %b: abbreviated month name (e.g. “Jan”)
- %B: full month name (e.g. “January”)
- %c: preferred date and time representation for the current locale
- %d: day of the month as a zero-padded decimal number (e.g. “01”-“31”)
- %H: hour (24-hour clock) as a zero-padded decimal number (e.g. “00”-“23”)
- %I: hour (12-hour clock) as a zero-padded decimal number (e.g. “01”-“12”)
- %j: day of the year as a zero-padded decimal number (e.g. “001”-“366”)
- %m: month as a zero-padded decimal number (e.g. “01”-“12”)
- %M: minute as a zero-padded decimal number (e.g. “00”-“59”)
- %p: either “AM” or “PM”
- %S: second as a zero-padded decimal number (e.g. “00”-“59”)
- %U: week number of the year (Sunday as the first day of the week) as a zero-padded decimal number (e.g. “00”-“53”)
- %w: weekday as a decimal number (0-Sunday, 6-Saturday)
- %W: week number of the year (Monday as the first day of the week) as a zero-padded decimal number (e.g. “00”-“53”)
- %x: preferred date representation for the current locale
- %X: preferred time representation for the current locale
- %y: year without century as a zero-padded decimal number (e.g. “00”-“99”)
- %Y: year with century as a decimal number
- %z: UTC offset in the form “+HHMM” or “-HHMM”
- %Z: time zone name (empty string if the object is naive)
- %%: a literal “%” character
By using these format codes, you can create custom date and time string representations using strftime().