How to Use the Python zip_longest() Function
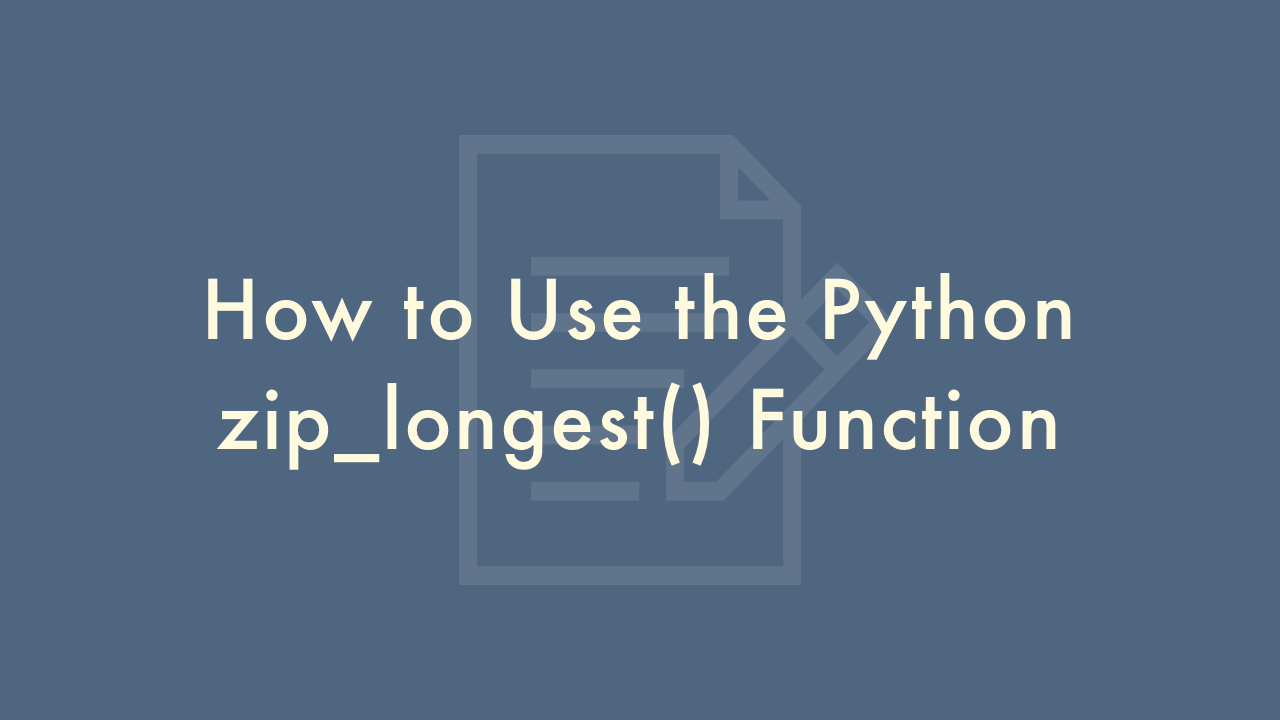
Contents
In this article, you will learn how to use the Python zip_longest() function.
Python zip_longest() Function
The zip_longest() function in Python’s itertools module is used to combine multiple iterables, with unequal lengths, into a single iterator of tuples. This function works similarly to the zip() function, but instead of stopping when the shortest iterable is exhausted, it continues until the longest iterable is exhausted and fills in missing values with a specified fillvalue (by default, None).
Here’s how you can use the zip_longest() function:
Import the zip_longest() function from the itertools module:
from itertools import zip_longest
Create the iterables that you want to combine. For example:
list1 = [1, 2, 3]
list2 = ['a', 'b']
Call the zip_longest() function and pass in the iterables as arguments. For example:
zipped = zip_longest(list1, list2)
Iterate over the zipped iterator to access the tuples. For example:
for item in zipped:
print(item)
This would output:
(1, 'a')
(2, 'b')
(3, None)
Note that the third tuple has None as the second element because list2 was shorter than list1, and so zip_longest() filled in the missing value with the default fillvalue of None.
You can also specify a different fillvalue by passing it as a keyword argument to the zip_longest() function. For example:
zipped = zip_longest(list1, list2, fillvalue='NA')
This would output:
(1, 'a')
(2, 'b')
(3, 'NA')
Note that the missing value in the third tuple is now ‘NA’ instead of None.