How to Read and Write Files in Python
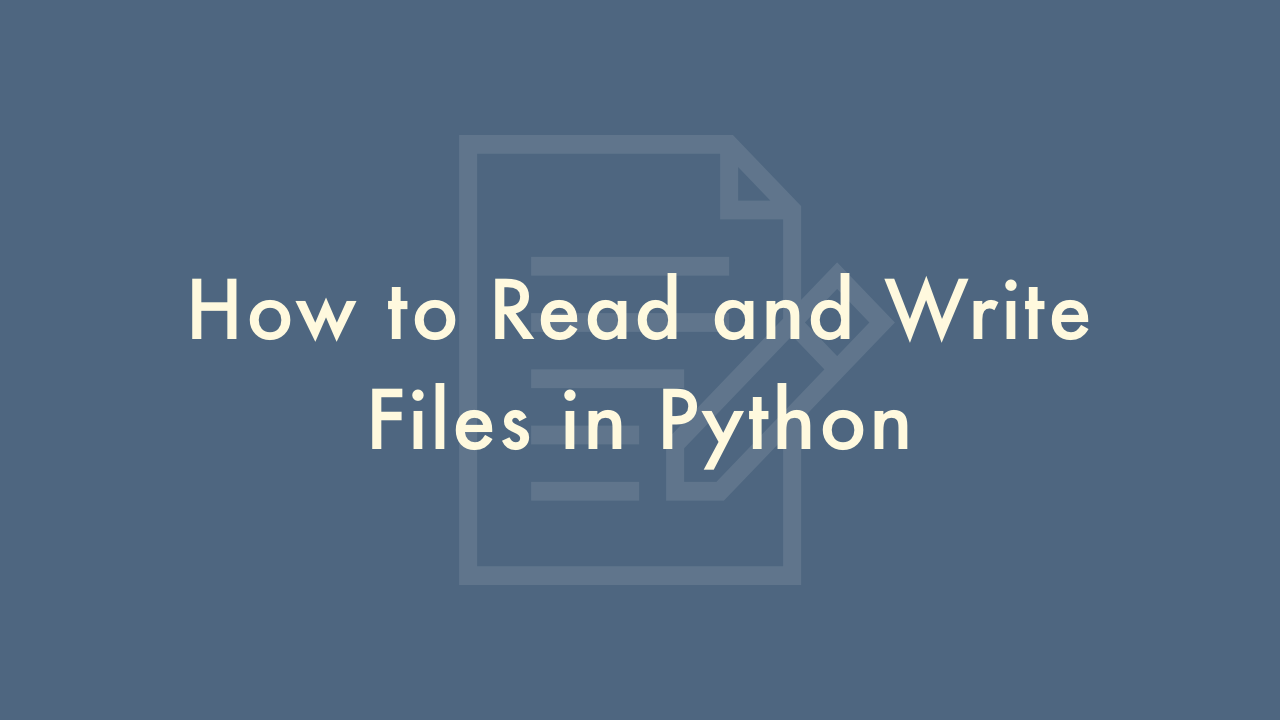
Contents
In this article, you will learn how to read and write Files in Python.
reading and writing files in Python
Python provides several methods for reading and writing files. Here are some of the most commonly used methods:
open
This method is used to open a file in Python. By default, it opens the file in text mode. You can specify the mode in which you want to open the file (read, write, append, etc.).
f = open("filename.txt", "mode")
write
This method is used to write data to a file. The write method takes a string as an argument and writes it to the file.
f = open("filename.txt", "w")
f.write("This is some text.")
f.close()
read
This method is used to read the contents of a file. By default, it reads the entire file, but you can specify how many characters you want to read.
f = open("filename.txt", "r")
content = f.read()
print(content)
f.close()
readline
This method reads a single line from the file.
f = open("filename.txt", "r")
print(f.readline())
f.close()
readlines
This method reads all the lines of a file and returns them as a list.
f = open("filename.txt", "r")
lines = f.readlines()
for line in lines:
print(line)
f.close()
close
This method is used to close a file. It is a good practice to close a file after you are done with it, so that resources can be freed up.
f = open("filename.txt", "r")
content = f.read()
f.close()
It’s worth noting that you can use the with statement to automatically close a file, even if an error occurs, without having to explicitly call the close method.
with open("filename.txt", "r") as f:
content = f.read()
Here’s a bit more information about the modes in which you can open a file in Python:
- “r” (read mode): This mode is used to read an existing file. If the file does not exist, an error is raised.
- “w” (write mode): This mode is used to write to a file. If the file already exists, its contents are truncated and overwritten. If the file does not exist, it is created.
- “a” (append mode): This mode is used to write to a file. If the file already exists, the new data is appended to the end of the file. If the file does not exist, it is created.
- “x” (exclusive creation mode): This mode is used to write to a file, but only if the file does not already exist. If the file already exists, an error is raised.
- “b” (binary mode): This mode is used to open a binary file, such as an image or audio file.
You can combine these modes as follows:
- “rb” (read binary mode)
- “wb” (write binary mode)
- “ab” (append binary mode)
- “xb” (exclusive creation binary mode)
It’s important to use the correct mode when opening a file, as using the wrong mode can lead to unexpected results, such as truncating the contents of a file or overwriting it.