How to Use the Python Numpy convolve() Function
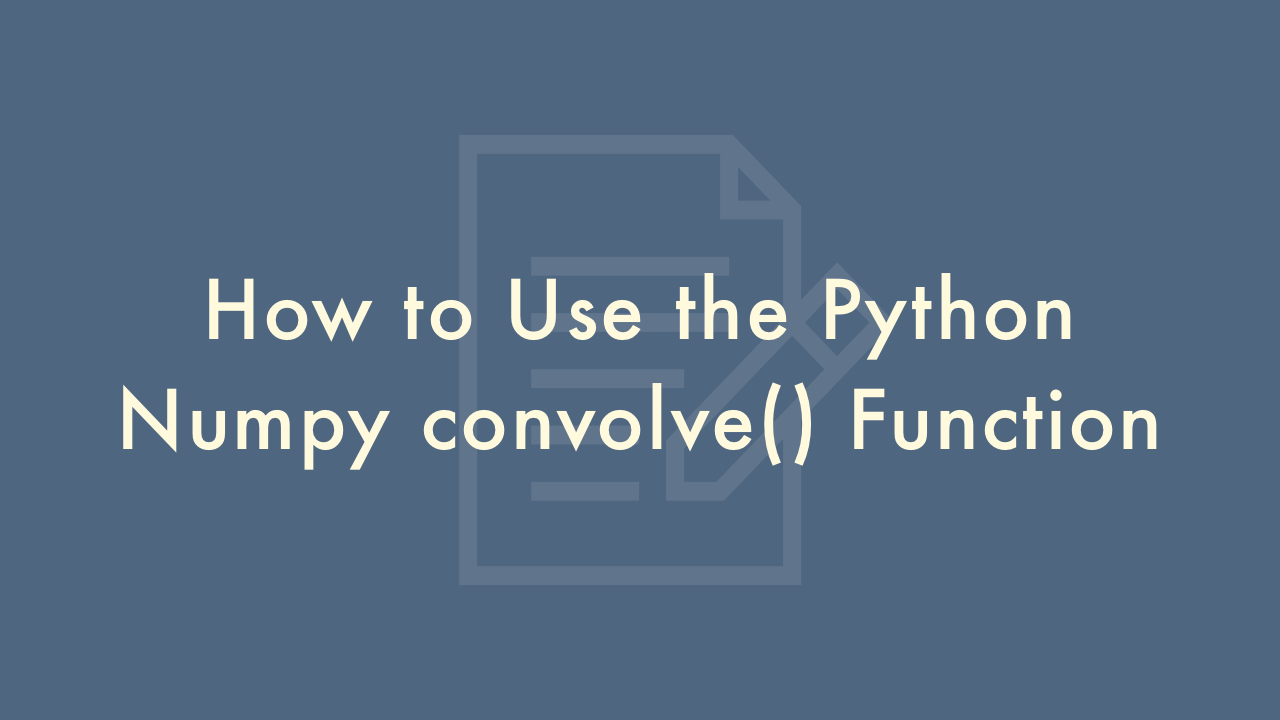
Contents
In this article, you will learn how to use the Python numpy convolve() function.
Numpy convolve() Function
The numpy.convolve() function in Python is used for signal processing and provides a way to perform linear convolution of two 1-dimensional arrays.
Syntax
The basic syntax for using the numpy.convolve() function is:
numpy.convolve(a, v, mode='full')
Parameters
a
,v
: The 1-dimensional input arraysmode
: Specifies the type of output
The a array is the input signal, and the v array is the filter or kernel.
The mode parameter specifies the size of the output array. The available options are:
mode='full'
: This is the default mode, and it returns the full convolution of the inputs. The output size is N+M-1, where N is the size of the input array a, and M is the size of the input array v.mode='same'
: This mode returns the central part of the convolution that is the same size as the input array a. The output size is max(N, M).mode='valid
: This mode returns only the part of the convolution that is computed without the zero-padded edges. The output size is abs(N-M)+1.
Example
Here is an example of using the numpy.convolve() function:
import numpy as np
# Create the input arrays
a = np.array([1, 2, 3])
v = np.array([0, 1, 0.5])
# Perform convolution with mode='full'
result_full = np.convolve(a, v, mode='full')
print(result_full)
# Perform convolution with mode='same'
result_same = np.convolve(a, v, mode='same')
print(result_same)
# Perform convolution with mode='valid'
result_valid = np.convolve(a, v, mode='valid')
print(result_valid)
In this example, we created two input arrays a and v and performed convolution using the numpy.convolve() function with all three available modes. The resulting output arrays for each mode are printed to the console.
Here is some more information about the numpy.convolve() function in Python:
- The numpy.convolve() function can also be used to perform 2-dimensional convolution on images by passing 2-dimensional input arrays. In this case, the v array represents the convolution kernel or filter, and the a array represents the input image.
- The numpy.convolve() function uses a straightforward implementation of the convolution operation, which is computationally expensive for larger input arrays. If you need to perform convolution on large arrays, it may be more efficient to use the scipy.signal.convolve2d() function, which provides a more optimized implementation.
- When performing convolution, the numpy.convolve() function performs zero-padding of the input arrays by default. This means that the output array may contain values that are not part of the original input data. You can control the amount of padding by specifying the mode parameter.
- The numpy.convolve() function is a general-purpose convolution function and can be used for a variety of signal processing tasks, including filtering, smoothing, and feature detection.
- The numpy.convolve() function can also be used for cross-correlation, which is similar to convolution but involves flipping the kernel before performing the operation.
Here is an example of using the numpy.convolve() function for cross-correlation:
import numpy as np
# Create the input arrays
a = np.array([1, 2, 3])
v = np.array([0, 1, 0.5])
# Flip the kernel
v_flipped = np.flip(v)
# Perform cross-correlation
result_corr = np.convolve(a, v_flipped, mode='valid')
print(result_corr)
In this example, we flipped the kernel v using the numpy.flip() function and performed cross-correlation by passing the flipped kernel to the numpy.convolve() function. The resulting output array is printed to the console.