How to Calculate Standard Deviation in Python
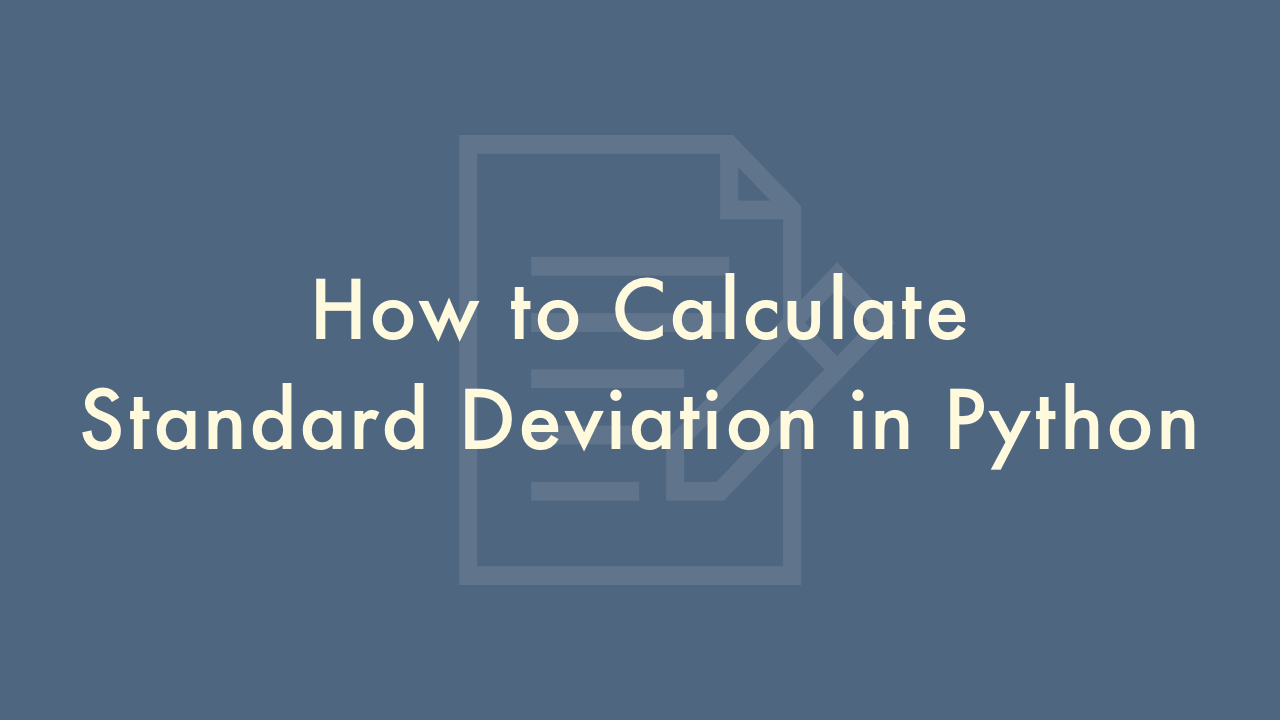
Contents
In this article, you will learn how to calculate standard deviation in Python.
Calculate standard deviation
To calculate the standard deviation in Python, you can use the statistics module or the numpy library. Here are the steps to calculate the standard deviation using both approaches:
Using the statistics module
Import the statistics module:
import statistics
Create a list of numbers for which you want to calculate the standard deviation.
data = [1, 2, 3, 4, 5]
Call the stdev() function from the statistics module and pass the list of numbers as the argument.
standard_deviation = statistics.stdev(data)
Print the calculated standard deviation.
print("Standard Deviation:", standard_deviation)
The complete code:
import statistics
data = [1, 2, 3, 4, 5]
standard_deviation = statistics.stdev(data)
print("Standard Deviation:", standard_deviation)
# Output:
# Standard Deviation: 1.5811388300841898
Using the numpy library
Import the numpy library:
import numpy as np
Create a list of numbers for which you want to calculate the standard deviation.
data = [1, 2, 3, 4, 5]
Call the std() function from the numpy library and pass the list of numbers as the argument.
standard_deviation = np.std(data)
Print the calculated standard deviation.
print("Standard Deviation:", standard_deviation)
The complete code:
import numpy as np
data = [1, 2, 3, 4, 5]
standard_deviation = np.std(data)
print("Standard Deviation:", standard_deviation)
# Output:
# Standard Deviation: 1.5811388300841898
Both approaches will give you the same result.