How to Use the Python “with” Statement
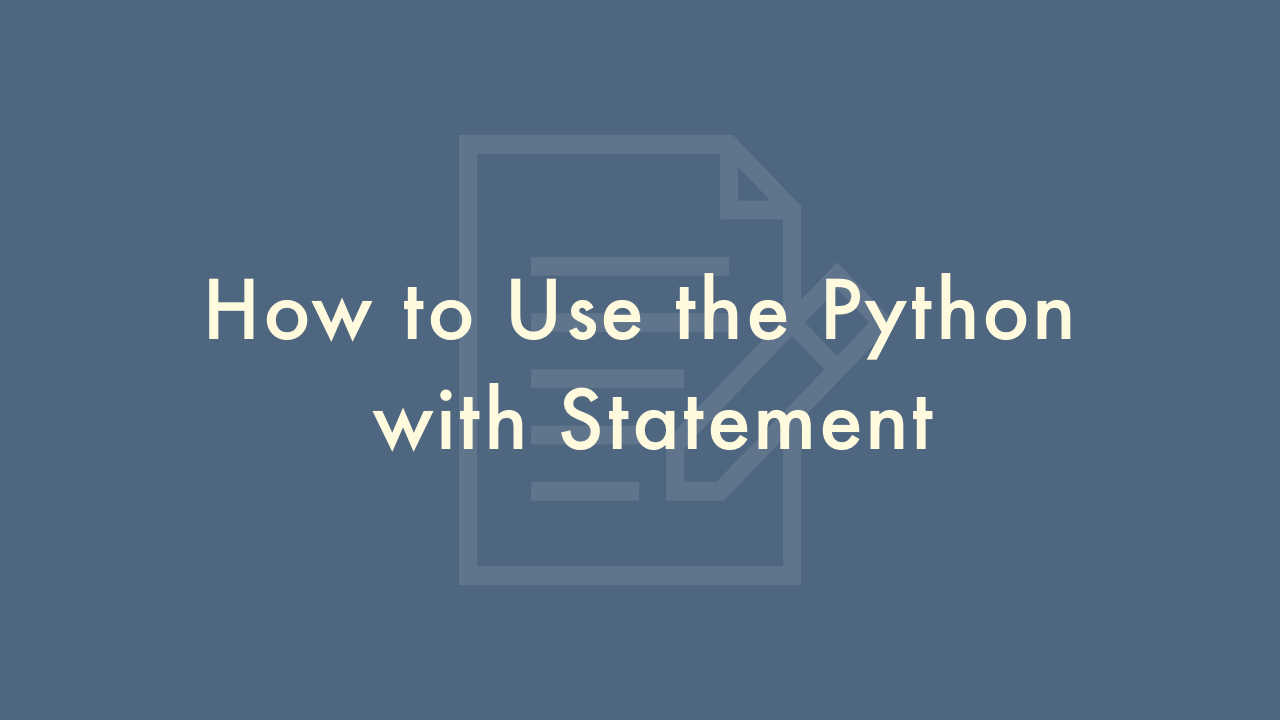
Contents
In this article, you will learn how to use the Python “with” Statement.
Python “with” Statement
The “with” statement in Python is used to wrap the execution of a block of code with methods defined by a context manager. A context manager is an object that defines the methods enter and exit. The purpose of these methods is to manage a context in which the code within the “with” statement is executed.
The “with” statement provides a convenient way to manage resources, such as file objects, sockets, and database connections, in a clean and efficient manner. The “with” statement ensures that the resources are properly acquired and released, even if an exception occurs within the block of code.
Here’s a simple example of how to use the “with” statement to open a file:
with open("file.txt", "r") as f:
contents = f.read()
print(contents)
In this example, the “with” statement creates a context in which the file “file.txt” is opened for reading. The file object is assigned to the variable “f”, which is used within the block of code to read the contents of the file. When the block of code is finished executing, the file is automatically closed, even if an exception was raised within the block. This ensures that the file is properly released and the resources it uses are freed.
Here’s some more information on the “with” statement and context managers in Python.
Advantages of Using the “with” Statement
Resource management
The “with” statement takes care of resource management, such as acquiring and releasing resources. This makes your code more readable and eliminates the need for explicit resource management, such as closing files or releasing locks.
Exception handling
If an exception occurs within the block of code executed by the “with” statement, the “with” statement ensures that the resources used by the block are properly released, even if the block raises an exception.
Cleanup actions
The “exit” method of a context manager can perform cleanup actions, such as closing a file or rolling back a transaction. This helps you ensure that resources are properly released, even if an exception occurs within the block of code.
Creating Custom Context Managers
You can create your own context managers by defining a class that implements the methods “enter” and “exit”. The “enter” method is called when the “with” statement is executed, and it returns an object that will be used within the block of code. The “exit” method is called when the block of code is finished executing, and it takes care of releasing resources and performing any necessary cleanup actions.
Here’s an example of a custom context manager that implements a lock:
class Lock:
def __init__(self):
self.locked = False
def __enter__(self):
if self.locked:
raise Exception("Lock is already acquired")
self.locked = True
return self
def __exit__(self, type, value, traceback):
self.locked = False
with Lock():
# code that requires a lock
In this example, the “Lock” class implements a lock that can be acquired and released. The “with” statement creates a context in which the lock is acquired, and the code within the block is executed. When the block is finished executing, the lock is automatically released, even if an exception occurs within the block.