How to Use the Numpy random.randn() Function in Python
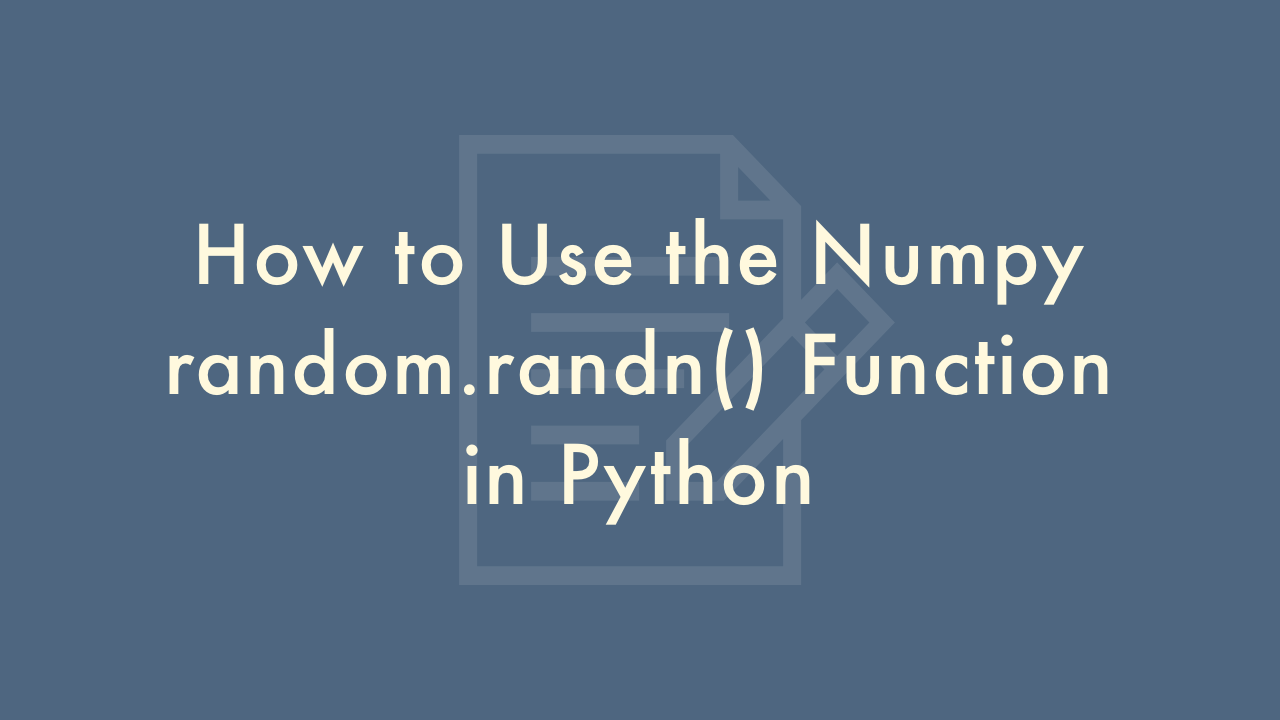
Contents
In this article, you will learn how to use the Numpy random.randn() function in Python.
The Numpy random.randn() Function
The numpy.random.randn() function is a tool in Python’s numpy library that can generate an array of random numbers from the standard normal distribution (mean=0, standard deviation=1).
Here’s how you can use it in your Python program:
Import the numpy library
Before using the numpy library, you must first import it into your Python script. This is done by writing the following line at the beginning of your script:
import numpy as np
This line tells Python to import the numpy library and create an alias for it called np. We use np instead of numpy throughout the code to save time and effort.
Call the random.randn() function
Once you have imported numpy, you can generate random numbers using the random.randn() function. This function returns an array of random numbers drawn from the standard normal distribution.
arr = np.random.randn(5)
print(arr)
This code generates an array of 5 random numbers from the standard normal distribution and prints it to the console.
Set the shape of the output array
You can also generate an array of random numbers with a specific shape using the random.randn() function. The shape of the array is determined by passing a tuple of integers to the function.
arr = np.random.randn(3, 4)
print(arr)
This code generates an array with 3 rows and 4 columns, each element drawn from the standard normal distribution.
Seed the random number generator
By default, the numpy random number generator is initialized with a seed value of None, which means it generates different random numbers every time you run the code. However, you can set a specific seed value to generate the same random numbers every time you run the code.
np.random.seed(42)
arr = np.random.randn(5)
print(arr)
This code sets the seed value to 42 and generates an array of 5 random numbers from the standard normal distribution. Running this code multiple times will generate the same array every time.